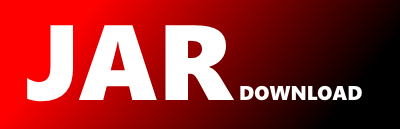
org.cloudfoundry.identity.uaa.web.ExceptionReportHttpMessageConverter Maven / Gradle / Ivy
Show all versions of cloudfoundry-identity-server Show documentation
/*******************************************************************************
* Cloud Foundry
* Copyright (c) [2009-2016] Pivotal Software, Inc. All Rights Reserved.
*
* This product is licensed to you under the Apache License, Version 2.0 (the "License").
* You may not use this product except in compliance with the License.
*
* This product includes a number of subcomponents with
* separate copyright notices and license terms. Your use of these
* subcomponents is subject to the terms and conditions of the
* subcomponent's license, as noted in the LICENSE file.
*******************************************************************************/
package org.cloudfoundry.identity.uaa.web;
import org.cloudfoundry.identity.uaa.util.UaaStringUtils;
import org.cloudfoundry.identity.uaa.web.ExceptionReport;
import org.springframework.http.HttpInputMessage;
import org.springframework.http.HttpOutputMessage;
import org.springframework.http.MediaType;
import org.springframework.http.converter.AbstractHttpMessageConverter;
import org.springframework.http.converter.HttpMessageConverter;
import org.springframework.http.converter.HttpMessageNotReadableException;
import org.springframework.http.converter.HttpMessageNotWritableException;
import org.springframework.web.client.RestTemplate;
import java.io.IOException;
import java.io.PrintWriter;
import java.io.StringWriter;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.LinkedHashSet;
import java.util.List;
import java.util.Map;
import java.util.Set;
/**
* @author Dave Syer
*
*/
public class ExceptionReportHttpMessageConverter extends AbstractHttpMessageConverter {
private static final HttpMessageConverter>[] DEFAULT_MESSAGE_CONVERTERS = new RestTemplate()
.getMessageConverters().toArray(
new HttpMessageConverter>[0]);
private HttpMessageConverter>[] messageConverters = DEFAULT_MESSAGE_CONVERTERS;
/**
* Set the message body converters to use.
*
* These converters are used to convert from and to HTTP requests and
* responses.
*/
public void setMessageConverters(HttpMessageConverter>[] messageConverters) {
this.messageConverters = messageConverters;
}
@Override
public List getSupportedMediaTypes() {
Set list = new LinkedHashSet();
for (HttpMessageConverter> converter : messageConverters) {
list.addAll(converter.getSupportedMediaTypes());
}
return new ArrayList(list);
}
@Override
protected boolean supports(Class> clazz) {
return ExceptionReport.class.isAssignableFrom(clazz);
}
@Override
protected ExceptionReport readInternal(Class extends ExceptionReport> clazz, HttpInputMessage inputMessage)
throws IOException, HttpMessageNotReadableException {
for (HttpMessageConverter> converter : messageConverters) {
for (MediaType mediaType : converter.getSupportedMediaTypes()) {
if (converter.canRead(Map.class, mediaType)) {
@SuppressWarnings({ "rawtypes", "unchecked" })
HttpMessageConverter