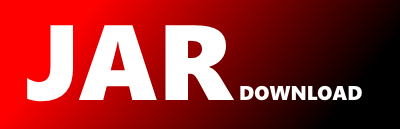
org.cloudfoundry.multiapps.controller.api.model.ImmutableOperation Maven / Gradle / Ivy
package org.cloudfoundry.multiapps.controller.api.model;
import com.fasterxml.jackson.annotation.JsonAutoDetect;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import com.fasterxml.jackson.databind.annotation.JsonSerialize;
import java.time.ZonedDateTime;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import org.cloudfoundry.multiapps.common.Nullable;
/**
* Immutable implementation of {@link Operation}.
*
* Use the builder to create immutable instances:
* {@code ImmutableOperation.builder()}.
*/
@SuppressWarnings({"all"})
public final class ImmutableOperation extends Operation {
private final @Nullable String processId;
private final @Nullable ProcessType processType;
private final @Nullable ZonedDateTime startedAt;
private final @Nullable ZonedDateTime endedAt;
private final @Nullable String spaceId;
private final @Nullable String mtaId;
private final @Nullable String namespace;
private final @Nullable String user;
private final @Nullable Boolean hasAcquiredLock;
private final @Nullable Operation.State state;
private final @Nullable ErrorType errorType;
private final List messages;
private final Map parameters;
private ImmutableOperation(
@Nullable String processId,
@Nullable ProcessType processType,
@Nullable ZonedDateTime startedAt,
@Nullable ZonedDateTime endedAt,
@Nullable String spaceId,
@Nullable String mtaId,
@Nullable String namespace,
@Nullable String user,
@Nullable Boolean hasAcquiredLock,
@Nullable Operation.State state,
@Nullable ErrorType errorType,
List messages,
Map parameters) {
this.processId = processId;
this.processType = processType;
this.startedAt = startedAt;
this.endedAt = endedAt;
this.spaceId = spaceId;
this.mtaId = mtaId;
this.namespace = namespace;
this.user = user;
this.hasAcquiredLock = hasAcquiredLock;
this.state = state;
this.errorType = errorType;
this.messages = messages;
this.parameters = parameters;
}
/**
* @return The value of the {@code processId} attribute
*/
@JsonProperty("processId")
@Override
public @Nullable String getProcessId() {
return processId;
}
/**
* @return The value of the {@code processType} attribute
*/
@JsonProperty("processType")
@JsonSerialize(using = ProcessTypeSerializer.class)
@JsonDeserialize(using = ProcessTypeDeserializer.class)
@Override
public @Nullable ProcessType getProcessType() {
return processType;
}
/**
* @return The value of the {@code startedAt} attribute
*/
@JsonProperty("startedAt")
@JsonSerialize(using = ZonedDateTimeSerializer.class)
@JsonDeserialize(using = ZonedDateTimeDeserializer.class)
@Override
public @Nullable ZonedDateTime getStartedAt() {
return startedAt;
}
/**
* @return The value of the {@code endedAt} attribute
*/
@JsonProperty("endedAt")
@JsonSerialize(using = ZonedDateTimeSerializer.class)
@JsonDeserialize(using = ZonedDateTimeDeserializer.class)
@Override
public @Nullable ZonedDateTime getEndedAt() {
return endedAt;
}
/**
* @return The value of the {@code spaceId} attribute
*/
@JsonProperty("spaceId")
@Override
public @Nullable String getSpaceId() {
return spaceId;
}
/**
* @return The value of the {@code mtaId} attribute
*/
@JsonProperty("mtaId")
@Override
public @Nullable String getMtaId() {
return mtaId;
}
/**
* @return The value of the {@code namespace} attribute
*/
@JsonProperty("namespace")
@Override
public @Nullable String getNamespace() {
return namespace;
}
/**
* @return The value of the {@code user} attribute
*/
@JsonProperty("user")
@Override
public @Nullable String getUser() {
return user;
}
/**
* @return The value of the {@code hasAcquiredLock} attribute
*/
@JsonProperty("acquiredLock")
@Override
public @Nullable Boolean hasAcquiredLock() {
return hasAcquiredLock;
}
/**
* @return The value of the {@code state} attribute
*/
@JsonProperty("state")
@Override
public @Nullable Operation.State getState() {
return state;
}
/**
* @return The value of the {@code errorType} attribute
*/
@JsonProperty("errorType")
@Override
public @Nullable ErrorType getErrorType() {
return errorType;
}
/**
* @return The value of the {@code messages} attribute
*/
@JsonProperty("messages")
@Override
public List getMessages() {
return messages;
}
/**
* @return The value of the {@code parameters} attribute
*/
@JsonProperty("parameters")
@Override
public Map getParameters() {
return parameters;
}
/**
* Copy the current immutable object by setting a value for the {@link Operation#getProcessId() processId} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for processId (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableOperation withProcessId(@Nullable String value) {
if (Objects.equals(this.processId, value)) return this;
return new ImmutableOperation(
value,
this.processType,
this.startedAt,
this.endedAt,
this.spaceId,
this.mtaId,
this.namespace,
this.user,
this.hasAcquiredLock,
this.state,
this.errorType,
this.messages,
this.parameters);
}
/**
* Copy the current immutable object by setting a value for the {@link Operation#getProcessType() processType} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for processType (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableOperation withProcessType(@Nullable ProcessType value) {
if (this.processType == value) return this;
return new ImmutableOperation(
this.processId,
value,
this.startedAt,
this.endedAt,
this.spaceId,
this.mtaId,
this.namespace,
this.user,
this.hasAcquiredLock,
this.state,
this.errorType,
this.messages,
this.parameters);
}
/**
* Copy the current immutable object by setting a value for the {@link Operation#getStartedAt() startedAt} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for startedAt (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableOperation withStartedAt(@Nullable ZonedDateTime value) {
if (this.startedAt == value) return this;
return new ImmutableOperation(
this.processId,
this.processType,
value,
this.endedAt,
this.spaceId,
this.mtaId,
this.namespace,
this.user,
this.hasAcquiredLock,
this.state,
this.errorType,
this.messages,
this.parameters);
}
/**
* Copy the current immutable object by setting a value for the {@link Operation#getEndedAt() endedAt} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for endedAt (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableOperation withEndedAt(@Nullable ZonedDateTime value) {
if (this.endedAt == value) return this;
return new ImmutableOperation(
this.processId,
this.processType,
this.startedAt,
value,
this.spaceId,
this.mtaId,
this.namespace,
this.user,
this.hasAcquiredLock,
this.state,
this.errorType,
this.messages,
this.parameters);
}
/**
* Copy the current immutable object by setting a value for the {@link Operation#getSpaceId() spaceId} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for spaceId (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableOperation withSpaceId(@Nullable String value) {
if (Objects.equals(this.spaceId, value)) return this;
return new ImmutableOperation(
this.processId,
this.processType,
this.startedAt,
this.endedAt,
value,
this.mtaId,
this.namespace,
this.user,
this.hasAcquiredLock,
this.state,
this.errorType,
this.messages,
this.parameters);
}
/**
* Copy the current immutable object by setting a value for the {@link Operation#getMtaId() mtaId} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for mtaId (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableOperation withMtaId(@Nullable String value) {
if (Objects.equals(this.mtaId, value)) return this;
return new ImmutableOperation(
this.processId,
this.processType,
this.startedAt,
this.endedAt,
this.spaceId,
value,
this.namespace,
this.user,
this.hasAcquiredLock,
this.state,
this.errorType,
this.messages,
this.parameters);
}
/**
* Copy the current immutable object by setting a value for the {@link Operation#getNamespace() namespace} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for namespace (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableOperation withNamespace(@Nullable String value) {
if (Objects.equals(this.namespace, value)) return this;
return new ImmutableOperation(
this.processId,
this.processType,
this.startedAt,
this.endedAt,
this.spaceId,
this.mtaId,
value,
this.user,
this.hasAcquiredLock,
this.state,
this.errorType,
this.messages,
this.parameters);
}
/**
* Copy the current immutable object by setting a value for the {@link Operation#getUser() user} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for user (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableOperation withUser(@Nullable String value) {
if (Objects.equals(this.user, value)) return this;
return new ImmutableOperation(
this.processId,
this.processType,
this.startedAt,
this.endedAt,
this.spaceId,
this.mtaId,
this.namespace,
value,
this.hasAcquiredLock,
this.state,
this.errorType,
this.messages,
this.parameters);
}
/**
* Copy the current immutable object by setting a value for the {@link Operation#hasAcquiredLock() hasAcquiredLock} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for hasAcquiredLock (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableOperation withHasAcquiredLock(@Nullable Boolean value) {
if (Objects.equals(this.hasAcquiredLock, value)) return this;
return new ImmutableOperation(
this.processId,
this.processType,
this.startedAt,
this.endedAt,
this.spaceId,
this.mtaId,
this.namespace,
this.user,
value,
this.state,
this.errorType,
this.messages,
this.parameters);
}
/**
* Copy the current immutable object by setting a value for the {@link Operation#getState() state} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for state (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableOperation withState(@Nullable Operation.State value) {
if (this.state == value) return this;
return new ImmutableOperation(
this.processId,
this.processType,
this.startedAt,
this.endedAt,
this.spaceId,
this.mtaId,
this.namespace,
this.user,
this.hasAcquiredLock,
value,
this.errorType,
this.messages,
this.parameters);
}
/**
* Copy the current immutable object by setting a value for the {@link Operation#getErrorType() errorType} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for errorType (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableOperation withErrorType(@Nullable ErrorType value) {
if (this.errorType == value) return this;
return new ImmutableOperation(
this.processId,
this.processType,
this.startedAt,
this.endedAt,
this.spaceId,
this.mtaId,
this.namespace,
this.user,
this.hasAcquiredLock,
this.state,
value,
this.messages,
this.parameters);
}
/**
* Copy the current immutable object with elements that replace the content of {@link Operation#getMessages() messages}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableOperation withMessages(Message... elements) {
List newValue = createUnmodifiableList(false, createSafeList(Arrays.asList(elements), true, false));
return new ImmutableOperation(
this.processId,
this.processType,
this.startedAt,
this.endedAt,
this.spaceId,
this.mtaId,
this.namespace,
this.user,
this.hasAcquiredLock,
this.state,
this.errorType,
newValue,
this.parameters);
}
/**
* Copy the current immutable object with elements that replace the content of {@link Operation#getMessages() messages}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of messages elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableOperation withMessages(Iterable extends Message> elements) {
if (this.messages == elements) return this;
List newValue = createUnmodifiableList(false, createSafeList(elements, true, false));
return new ImmutableOperation(
this.processId,
this.processType,
this.startedAt,
this.endedAt,
this.spaceId,
this.mtaId,
this.namespace,
this.user,
this.hasAcquiredLock,
this.state,
this.errorType,
newValue,
this.parameters);
}
/**
* Copy the current immutable object by replacing the {@link Operation#getParameters() parameters} map with the specified map.
* Nulls are not permitted as keys or values.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param entries The entries to be added to the parameters map
* @return A modified copy of {@code this} object
*/
public final ImmutableOperation withParameters(Map entries) {
if (this.parameters == entries) return this;
Map newValue = createUnmodifiableMap(false, false, entries);
return new ImmutableOperation(
this.processId,
this.processType,
this.startedAt,
this.endedAt,
this.spaceId,
this.mtaId,
this.namespace,
this.user,
this.hasAcquiredLock,
this.state,
this.errorType,
this.messages,
newValue);
}
/**
* This instance is equal to all instances of {@code ImmutableOperation} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(Object another) {
if (this == another) return true;
return another instanceof ImmutableOperation
&& equalTo(0, (ImmutableOperation) another);
}
private boolean equalTo(int synthetic, ImmutableOperation another) {
return Objects.equals(processId, another.processId)
&& Objects.equals(processType, another.processType)
&& Objects.equals(startedAt, another.startedAt)
&& Objects.equals(endedAt, another.endedAt)
&& Objects.equals(spaceId, another.spaceId)
&& Objects.equals(mtaId, another.mtaId)
&& Objects.equals(namespace, another.namespace)
&& Objects.equals(user, another.user)
&& Objects.equals(hasAcquiredLock, another.hasAcquiredLock)
&& Objects.equals(state, another.state)
&& Objects.equals(errorType, another.errorType)
&& messages.equals(another.messages)
&& parameters.equals(another.parameters);
}
/**
* Computes a hash code from attributes: {@code processId}, {@code processType}, {@code startedAt}, {@code endedAt}, {@code spaceId}, {@code mtaId}, {@code namespace}, {@code user}, {@code hasAcquiredLock}, {@code state}, {@code errorType}, {@code messages}, {@code parameters}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 5381;
h += (h << 5) + Objects.hashCode(processId);
h += (h << 5) + Objects.hashCode(processType);
h += (h << 5) + Objects.hashCode(startedAt);
h += (h << 5) + Objects.hashCode(endedAt);
h += (h << 5) + Objects.hashCode(spaceId);
h += (h << 5) + Objects.hashCode(mtaId);
h += (h << 5) + Objects.hashCode(namespace);
h += (h << 5) + Objects.hashCode(user);
h += (h << 5) + Objects.hashCode(hasAcquiredLock);
h += (h << 5) + Objects.hashCode(state);
h += (h << 5) + Objects.hashCode(errorType);
h += (h << 5) + messages.hashCode();
h += (h << 5) + parameters.hashCode();
return h;
}
/**
* Prints the immutable value {@code Operation} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
return "Operation{"
+ "processId=" + processId
+ ", processType=" + processType
+ ", startedAt=" + startedAt
+ ", endedAt=" + endedAt
+ ", spaceId=" + spaceId
+ ", mtaId=" + mtaId
+ ", namespace=" + namespace
+ ", user=" + user
+ ", hasAcquiredLock=" + hasAcquiredLock
+ ", state=" + state
+ ", errorType=" + errorType
+ ", messages=" + messages
+ ", parameters=" + parameters
+ "}";
}
/**
* Utility type used to correctly read immutable object from JSON representation.
* @deprecated Do not use this type directly, it exists only for the Jackson-binding infrastructure
*/
@Deprecated
@JsonDeserialize
@JsonAutoDetect(fieldVisibility = JsonAutoDetect.Visibility.NONE)
static final class Json extends Operation {
String processId;
ProcessType processType;
ZonedDateTime startedAt;
ZonedDateTime endedAt;
String spaceId;
String mtaId;
String namespace;
String user;
Boolean hasAcquiredLock;
Operation.State state;
ErrorType errorType;
List messages = Collections.emptyList();
Map parameters = Collections.emptyMap();
@JsonProperty("processId")
public void setProcessId(@Nullable String processId) {
this.processId = processId;
}
@JsonProperty("processType")
@JsonSerialize(using = ProcessTypeSerializer.class)
@JsonDeserialize(using = ProcessTypeDeserializer.class)
public void setProcessType(@Nullable ProcessType processType) {
this.processType = processType;
}
@JsonProperty("startedAt")
@JsonSerialize(using = ZonedDateTimeSerializer.class)
@JsonDeserialize(using = ZonedDateTimeDeserializer.class)
public void setStartedAt(@Nullable ZonedDateTime startedAt) {
this.startedAt = startedAt;
}
@JsonProperty("endedAt")
@JsonSerialize(using = ZonedDateTimeSerializer.class)
@JsonDeserialize(using = ZonedDateTimeDeserializer.class)
public void setEndedAt(@Nullable ZonedDateTime endedAt) {
this.endedAt = endedAt;
}
@JsonProperty("spaceId")
public void setSpaceId(@Nullable String spaceId) {
this.spaceId = spaceId;
}
@JsonProperty("mtaId")
public void setMtaId(@Nullable String mtaId) {
this.mtaId = mtaId;
}
@JsonProperty("namespace")
public void setNamespace(@Nullable String namespace) {
this.namespace = namespace;
}
@JsonProperty("user")
public void setUser(@Nullable String user) {
this.user = user;
}
@JsonProperty("acquiredLock")
public void setHasAcquiredLock(@Nullable Boolean hasAcquiredLock) {
this.hasAcquiredLock = hasAcquiredLock;
}
@JsonProperty("state")
public void setState(@Nullable Operation.State state) {
this.state = state;
}
@JsonProperty("errorType")
public void setErrorType(@Nullable ErrorType errorType) {
this.errorType = errorType;
}
@JsonProperty("messages")
public void setMessages(List messages) {
this.messages = messages;
}
@JsonProperty("parameters")
public void setParameters(Map parameters) {
this.parameters = parameters;
}
@Override
public String getProcessId() { throw new UnsupportedOperationException(); }
@Override
public ProcessType getProcessType() { throw new UnsupportedOperationException(); }
@Override
public ZonedDateTime getStartedAt() { throw new UnsupportedOperationException(); }
@Override
public ZonedDateTime getEndedAt() { throw new UnsupportedOperationException(); }
@Override
public String getSpaceId() { throw new UnsupportedOperationException(); }
@Override
public String getMtaId() { throw new UnsupportedOperationException(); }
@Override
public String getNamespace() { throw new UnsupportedOperationException(); }
@Override
public String getUser() { throw new UnsupportedOperationException(); }
@Override
public Boolean hasAcquiredLock() { throw new UnsupportedOperationException(); }
@Override
public Operation.State getState() { throw new UnsupportedOperationException(); }
@Override
public ErrorType getErrorType() { throw new UnsupportedOperationException(); }
@Override
public List getMessages() { throw new UnsupportedOperationException(); }
@Override
public Map getParameters() { throw new UnsupportedOperationException(); }
}
/**
* @param json A JSON-bindable data structure
* @return An immutable value type
* @deprecated Do not use this method directly, it exists only for the Jackson-binding infrastructure
*/
@Deprecated
@JsonCreator(mode = JsonCreator.Mode.DELEGATING)
static ImmutableOperation fromJson(Json json) {
ImmutableOperation.Builder builder = ImmutableOperation.builder();
if (json.processId != null) {
builder.processId(json.processId);
}
if (json.processType != null) {
builder.processType(json.processType);
}
if (json.startedAt != null) {
builder.startedAt(json.startedAt);
}
if (json.endedAt != null) {
builder.endedAt(json.endedAt);
}
if (json.spaceId != null) {
builder.spaceId(json.spaceId);
}
if (json.mtaId != null) {
builder.mtaId(json.mtaId);
}
if (json.namespace != null) {
builder.namespace(json.namespace);
}
if (json.user != null) {
builder.user(json.user);
}
if (json.hasAcquiredLock != null) {
builder.hasAcquiredLock(json.hasAcquiredLock);
}
if (json.state != null) {
builder.state(json.state);
}
if (json.errorType != null) {
builder.errorType(json.errorType);
}
if (json.messages != null) {
builder.addAllMessages(json.messages);
}
if (json.parameters != null) {
builder.putAllParameters(json.parameters);
}
return builder.build();
}
/**
* Creates an immutable copy of a {@link Operation} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable Operation instance
*/
public static ImmutableOperation copyOf(Operation instance) {
if (instance instanceof ImmutableOperation) {
return (ImmutableOperation) instance;
}
return ImmutableOperation.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link ImmutableOperation ImmutableOperation}.
*
* ImmutableOperation.builder()
* .processId(String | null) // nullable {@link Operation#getProcessId() processId}
* .processType(org.cloudfoundry.multiapps.controller.api.model.ProcessType | null) // nullable {@link Operation#getProcessType() processType}
* .startedAt(java.time.ZonedDateTime | null) // nullable {@link Operation#getStartedAt() startedAt}
* .endedAt(java.time.ZonedDateTime | null) // nullable {@link Operation#getEndedAt() endedAt}
* .spaceId(String | null) // nullable {@link Operation#getSpaceId() spaceId}
* .mtaId(String | null) // nullable {@link Operation#getMtaId() mtaId}
* .namespace(String | null) // nullable {@link Operation#getNamespace() namespace}
* .user(String | null) // nullable {@link Operation#getUser() user}
* .hasAcquiredLock(Boolean | null) // nullable {@link Operation#hasAcquiredLock() hasAcquiredLock}
* .state(org.cloudfoundry.multiapps.controller.api.model.Operation.State | null) // nullable {@link Operation#getState() state}
* .errorType(org.cloudfoundry.multiapps.controller.api.model.ErrorType | null) // nullable {@link Operation#getErrorType() errorType}
* .addMessage|addAllMessages(org.cloudfoundry.multiapps.controller.api.model.Message) // {@link Operation#getMessages() messages} elements
* .putParameter|putAllParameters(String => Object) // {@link Operation#getParameters() parameters} mappings
* .build();
*
* @return A new ImmutableOperation builder
*/
public static ImmutableOperation.Builder builder() {
return new ImmutableOperation.Builder();
}
/**
* Builds instances of type {@link ImmutableOperation ImmutableOperation}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
public static final class Builder {
private String processId;
private ProcessType processType;
private ZonedDateTime startedAt;
private ZonedDateTime endedAt;
private String spaceId;
private String mtaId;
private String namespace;
private String user;
private Boolean hasAcquiredLock;
private Operation.State state;
private ErrorType errorType;
private List messages = new ArrayList();
private Map parameters = new LinkedHashMap();
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code Operation} instance.
* Regular attribute values will be replaced with those from the given instance.
* Absent optional values will not replace present values.
* Collection elements and entries will be added, not replaced.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(Operation instance) {
Objects.requireNonNull(instance, "instance");
@Nullable String processIdValue = instance.getProcessId();
if (processIdValue != null) {
processId(processIdValue);
}
@Nullable ProcessType processTypeValue = instance.getProcessType();
if (processTypeValue != null) {
processType(processTypeValue);
}
@Nullable ZonedDateTime startedAtValue = instance.getStartedAt();
if (startedAtValue != null) {
startedAt(startedAtValue);
}
@Nullable ZonedDateTime endedAtValue = instance.getEndedAt();
if (endedAtValue != null) {
endedAt(endedAtValue);
}
@Nullable String spaceIdValue = instance.getSpaceId();
if (spaceIdValue != null) {
spaceId(spaceIdValue);
}
@Nullable String mtaIdValue = instance.getMtaId();
if (mtaIdValue != null) {
mtaId(mtaIdValue);
}
@Nullable String namespaceValue = instance.getNamespace();
if (namespaceValue != null) {
namespace(namespaceValue);
}
@Nullable String userValue = instance.getUser();
if (userValue != null) {
user(userValue);
}
@Nullable Boolean hasAcquiredLockValue = instance.hasAcquiredLock();
if (hasAcquiredLockValue != null) {
hasAcquiredLock(hasAcquiredLockValue);
}
@Nullable Operation.State stateValue = instance.getState();
if (stateValue != null) {
state(stateValue);
}
@Nullable ErrorType errorTypeValue = instance.getErrorType();
if (errorTypeValue != null) {
errorType(errorTypeValue);
}
addAllMessages(instance.getMessages());
putAllParameters(instance.getParameters());
return this;
}
/**
* Initializes the value for the {@link Operation#getProcessId() processId} attribute.
* @param processId The value for processId (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("processId")
public final Builder processId(@Nullable String processId) {
this.processId = processId;
return this;
}
/**
* Initializes the value for the {@link Operation#getProcessType() processType} attribute.
* @param processType The value for processType (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("processType")
@JsonSerialize(using = ProcessTypeSerializer.class)
@JsonDeserialize(using = ProcessTypeDeserializer.class)
public final Builder processType(@Nullable ProcessType processType) {
this.processType = processType;
return this;
}
/**
* Initializes the value for the {@link Operation#getStartedAt() startedAt} attribute.
* @param startedAt The value for startedAt (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("startedAt")
@JsonSerialize(using = ZonedDateTimeSerializer.class)
@JsonDeserialize(using = ZonedDateTimeDeserializer.class)
public final Builder startedAt(@Nullable ZonedDateTime startedAt) {
this.startedAt = startedAt;
return this;
}
/**
* Initializes the value for the {@link Operation#getEndedAt() endedAt} attribute.
* @param endedAt The value for endedAt (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("endedAt")
@JsonSerialize(using = ZonedDateTimeSerializer.class)
@JsonDeserialize(using = ZonedDateTimeDeserializer.class)
public final Builder endedAt(@Nullable ZonedDateTime endedAt) {
this.endedAt = endedAt;
return this;
}
/**
* Initializes the value for the {@link Operation#getSpaceId() spaceId} attribute.
* @param spaceId The value for spaceId (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("spaceId")
public final Builder spaceId(@Nullable String spaceId) {
this.spaceId = spaceId;
return this;
}
/**
* Initializes the value for the {@link Operation#getMtaId() mtaId} attribute.
* @param mtaId The value for mtaId (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("mtaId")
public final Builder mtaId(@Nullable String mtaId) {
this.mtaId = mtaId;
return this;
}
/**
* Initializes the value for the {@link Operation#getNamespace() namespace} attribute.
* @param namespace The value for namespace (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("namespace")
public final Builder namespace(@Nullable String namespace) {
this.namespace = namespace;
return this;
}
/**
* Initializes the value for the {@link Operation#getUser() user} attribute.
* @param user The value for user (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("user")
public final Builder user(@Nullable String user) {
this.user = user;
return this;
}
/**
* Initializes the value for the {@link Operation#hasAcquiredLock() hasAcquiredLock} attribute.
* @param hasAcquiredLock The value for hasAcquiredLock (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("acquiredLock")
public final Builder hasAcquiredLock(@Nullable Boolean hasAcquiredLock) {
this.hasAcquiredLock = hasAcquiredLock;
return this;
}
/**
* Initializes the value for the {@link Operation#getState() state} attribute.
* @param state The value for state (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("state")
public final Builder state(@Nullable Operation.State state) {
this.state = state;
return this;
}
/**
* Initializes the value for the {@link Operation#getErrorType() errorType} attribute.
* @param errorType The value for errorType (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("errorType")
public final Builder errorType(@Nullable ErrorType errorType) {
this.errorType = errorType;
return this;
}
/**
* Adds one element to {@link Operation#getMessages() messages} list.
* @param element A messages element
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addMessage(Message element) {
this.messages.add(Objects.requireNonNull(element, "messages element"));
return this;
}
/**
* Adds elements to {@link Operation#getMessages() messages} list.
* @param elements An array of messages elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addMessages(Message... elements) {
for (Message element : elements) {
this.messages.add(Objects.requireNonNull(element, "messages element"));
}
return this;
}
/**
* Sets or replaces all elements for {@link Operation#getMessages() messages} list.
* @param elements An iterable of messages elements
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("messages")
public final Builder messages(Iterable extends Message> elements) {
this.messages.clear();
return addAllMessages(elements);
}
/**
* Adds elements to {@link Operation#getMessages() messages} list.
* @param elements An iterable of messages elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addAllMessages(Iterable extends Message> elements) {
for (Message element : elements) {
this.messages.add(Objects.requireNonNull(element, "messages element"));
}
return this;
}
/**
* Put one entry to the {@link Operation#getParameters() parameters} map.
* @param key The key in the parameters map
* @param value The associated value in the parameters map
* @return {@code this} builder for use in a chained invocation
*/
public final Builder putParameter(String key, Object value) {
this.parameters.put(key, value);
return this;
}
/**
* Put one entry to the {@link Operation#getParameters() parameters} map. Nulls are not permitted
* @param entry The key and value entry
* @return {@code this} builder for use in a chained invocation
*/
public final Builder putParameter(Map.Entry entry) {
String k = entry.getKey();
Object v = entry.getValue();
this.parameters.put(k, v);
return this;
}
/**
* Sets or replaces all mappings from the specified map as entries for the {@link Operation#getParameters() parameters} map. Nulls are not permitted
* @param entries The entries that will be added to the parameters map
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("parameters")
public final Builder parameters(Map entries) {
this.parameters.clear();
return putAllParameters(entries);
}
/**
* Put all mappings from the specified map as entries to {@link Operation#getParameters() parameters} map. Nulls are not permitted
* @param entries The entries that will be added to the parameters map
* @return {@code this} builder for use in a chained invocation
*/
public final Builder putAllParameters(Map entries) {
for (Map.Entry e : entries.entrySet()) {
String k = e.getKey();
Object v = e.getValue();
this.parameters.put(k, v);
}
return this;
}
/**
* Builds a new {@link ImmutableOperation ImmutableOperation}.
* @return An immutable instance of Operation
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public ImmutableOperation build() {
return new ImmutableOperation(
processId,
processType,
startedAt,
endedAt,
spaceId,
mtaId,
namespace,
user,
hasAcquiredLock,
state,
errorType,
createUnmodifiableList(true, messages),
createUnmodifiableMap(false, false, parameters));
}
}
private static List createSafeList(Iterable extends T> iterable, boolean checkNulls, boolean skipNulls) {
ArrayList list;
if (iterable instanceof Collection>) {
int size = ((Collection>) iterable).size();
if (size == 0) return Collections.emptyList();
list = new ArrayList<>(size);
} else {
list = new ArrayList<>();
}
for (T element : iterable) {
if (skipNulls && element == null) continue;
if (checkNulls) Objects.requireNonNull(element, "element");
list.add(element);
}
return list;
}
private static List createUnmodifiableList(boolean clone, List list) {
switch(list.size()) {
case 0: return Collections.emptyList();
case 1: return Collections.singletonList(list.get(0));
default:
if (clone) {
return Collections.unmodifiableList(new ArrayList<>(list));
} else {
if (list instanceof ArrayList>) {
((ArrayList>) list).trimToSize();
}
return Collections.unmodifiableList(list);
}
}
}
private static Map createUnmodifiableMap(boolean checkNulls, boolean skipNulls, Map extends K, ? extends V> map) {
switch (map.size()) {
case 0: return Collections.emptyMap();
case 1: {
Map.Entry extends K, ? extends V> e = map.entrySet().iterator().next();
K k = e.getKey();
V v = e.getValue();
if (checkNulls) {
Objects.requireNonNull(k, "key");
Objects.requireNonNull(v, v == null ? "value for key: " + k : null);
}
if (skipNulls && (k == null || v == null)) {
return Collections.emptyMap();
}
return Collections.singletonMap(k, v);
}
default: {
Map linkedMap = new LinkedHashMap<>(map.size() * 4 / 3 + 1);
if (skipNulls || checkNulls) {
for (Map.Entry extends K, ? extends V> e : map.entrySet()) {
K k = e.getKey();
V v = e.getValue();
if (skipNulls) {
if (k == null || v == null) continue;
} else if (checkNulls) {
Objects.requireNonNull(k, "key");
Objects.requireNonNull(v, v == null ? "value for key: " + k : null);
}
linkedMap.put(k, v);
}
} else {
linkedMap.putAll(map);
}
return Collections.unmodifiableMap(linkedMap);
}
}
}
}