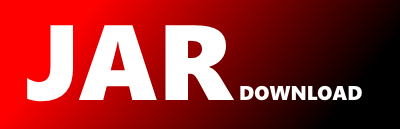
org.cloudfoundry.multiapps.controller.persistence.model.ImmutableAsyncUploadJobEntry Maven / Gradle / Ivy
package org.cloudfoundry.multiapps.controller.persistence.model;
import java.time.LocalDateTime;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
import org.cloudfoundry.multiapps.common.Nullable;
/**
* Immutable implementation of {@link AsyncUploadJobEntry}.
*
* Use the builder to create immutable instances:
* {@code ImmutableAsyncUploadJobEntry.builder()}.
*/
@SuppressWarnings({"all"})
public final class ImmutableAsyncUploadJobEntry
implements AsyncUploadJobEntry {
private final String id;
private final AsyncUploadJobEntry.State state;
private final String user;
private final String url;
private final @Nullable LocalDateTime addedAt;
private final @Nullable LocalDateTime startedAt;
private final @Nullable LocalDateTime finishedAt;
private final String spaceGuid;
private final @Nullable String namespace;
private final @Nullable String error;
private final @Nullable String fileId;
private final @Nullable String mtaId;
private final Integer instanceIndex;
private ImmutableAsyncUploadJobEntry(
String id,
AsyncUploadJobEntry.State state,
String user,
String url,
@Nullable LocalDateTime addedAt,
@Nullable LocalDateTime startedAt,
@Nullable LocalDateTime finishedAt,
String spaceGuid,
@Nullable String namespace,
@Nullable String error,
@Nullable String fileId,
@Nullable String mtaId,
Integer instanceIndex) {
this.id = id;
this.state = state;
this.user = user;
this.url = url;
this.addedAt = addedAt;
this.startedAt = startedAt;
this.finishedAt = finishedAt;
this.spaceGuid = spaceGuid;
this.namespace = namespace;
this.error = error;
this.fileId = fileId;
this.mtaId = mtaId;
this.instanceIndex = instanceIndex;
}
/**
* @return The value of the {@code id} attribute
*/
@Override
public String getId() {
return id;
}
/**
* @return The value of the {@code state} attribute
*/
@Override
public AsyncUploadJobEntry.State getState() {
return state;
}
/**
* @return The value of the {@code user} attribute
*/
@Override
public String getUser() {
return user;
}
/**
* @return The value of the {@code url} attribute
*/
@Override
public String getUrl() {
return url;
}
/**
* @return The value of the {@code addedAt} attribute
*/
@Override
public @Nullable LocalDateTime getAddedAt() {
return addedAt;
}
/**
* @return The value of the {@code startedAt} attribute
*/
@Override
public @Nullable LocalDateTime getStartedAt() {
return startedAt;
}
/**
* @return The value of the {@code finishedAt} attribute
*/
@Override
public @Nullable LocalDateTime getFinishedAt() {
return finishedAt;
}
/**
* @return The value of the {@code spaceGuid} attribute
*/
@Override
public String getSpaceGuid() {
return spaceGuid;
}
/**
* @return The value of the {@code namespace} attribute
*/
@Override
public @Nullable String getNamespace() {
return namespace;
}
/**
* @return The value of the {@code error} attribute
*/
@Override
public @Nullable String getError() {
return error;
}
/**
* @return The value of the {@code fileId} attribute
*/
@Override
public @Nullable String getFileId() {
return fileId;
}
/**
* @return The value of the {@code mtaId} attribute
*/
@Override
public @Nullable String getMtaId() {
return mtaId;
}
/**
* @return The value of the {@code instanceIndex} attribute
*/
@Override
public Integer getInstanceIndex() {
return instanceIndex;
}
/**
* Copy the current immutable object by setting a value for the {@link AsyncUploadJobEntry#getId() id} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for id
* @return A modified copy of the {@code this} object
*/
public final ImmutableAsyncUploadJobEntry withId(String value) {
String newValue = Objects.requireNonNull(value, "id");
if (this.id.equals(newValue)) return this;
return new ImmutableAsyncUploadJobEntry(
newValue,
this.state,
this.user,
this.url,
this.addedAt,
this.startedAt,
this.finishedAt,
this.spaceGuid,
this.namespace,
this.error,
this.fileId,
this.mtaId,
this.instanceIndex);
}
/**
* Copy the current immutable object by setting a value for the {@link AsyncUploadJobEntry#getState() state} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for state
* @return A modified copy of the {@code this} object
*/
public final ImmutableAsyncUploadJobEntry withState(AsyncUploadJobEntry.State value) {
AsyncUploadJobEntry.State newValue = Objects.requireNonNull(value, "state");
if (this.state == newValue) return this;
return new ImmutableAsyncUploadJobEntry(
this.id,
newValue,
this.user,
this.url,
this.addedAt,
this.startedAt,
this.finishedAt,
this.spaceGuid,
this.namespace,
this.error,
this.fileId,
this.mtaId,
this.instanceIndex);
}
/**
* Copy the current immutable object by setting a value for the {@link AsyncUploadJobEntry#getUser() user} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for user
* @return A modified copy of the {@code this} object
*/
public final ImmutableAsyncUploadJobEntry withUser(String value) {
String newValue = Objects.requireNonNull(value, "user");
if (this.user.equals(newValue)) return this;
return new ImmutableAsyncUploadJobEntry(
this.id,
this.state,
newValue,
this.url,
this.addedAt,
this.startedAt,
this.finishedAt,
this.spaceGuid,
this.namespace,
this.error,
this.fileId,
this.mtaId,
this.instanceIndex);
}
/**
* Copy the current immutable object by setting a value for the {@link AsyncUploadJobEntry#getUrl() url} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for url
* @return A modified copy of the {@code this} object
*/
public final ImmutableAsyncUploadJobEntry withUrl(String value) {
String newValue = Objects.requireNonNull(value, "url");
if (this.url.equals(newValue)) return this;
return new ImmutableAsyncUploadJobEntry(
this.id,
this.state,
this.user,
newValue,
this.addedAt,
this.startedAt,
this.finishedAt,
this.spaceGuid,
this.namespace,
this.error,
this.fileId,
this.mtaId,
this.instanceIndex);
}
/**
* Copy the current immutable object by setting a value for the {@link AsyncUploadJobEntry#getAddedAt() addedAt} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for addedAt (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableAsyncUploadJobEntry withAddedAt(@Nullable LocalDateTime value) {
if (this.addedAt == value) return this;
return new ImmutableAsyncUploadJobEntry(
this.id,
this.state,
this.user,
this.url,
value,
this.startedAt,
this.finishedAt,
this.spaceGuid,
this.namespace,
this.error,
this.fileId,
this.mtaId,
this.instanceIndex);
}
/**
* Copy the current immutable object by setting a value for the {@link AsyncUploadJobEntry#getStartedAt() startedAt} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for startedAt (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableAsyncUploadJobEntry withStartedAt(@Nullable LocalDateTime value) {
if (this.startedAt == value) return this;
return new ImmutableAsyncUploadJobEntry(
this.id,
this.state,
this.user,
this.url,
this.addedAt,
value,
this.finishedAt,
this.spaceGuid,
this.namespace,
this.error,
this.fileId,
this.mtaId,
this.instanceIndex);
}
/**
* Copy the current immutable object by setting a value for the {@link AsyncUploadJobEntry#getFinishedAt() finishedAt} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for finishedAt (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableAsyncUploadJobEntry withFinishedAt(@Nullable LocalDateTime value) {
if (this.finishedAt == value) return this;
return new ImmutableAsyncUploadJobEntry(
this.id,
this.state,
this.user,
this.url,
this.addedAt,
this.startedAt,
value,
this.spaceGuid,
this.namespace,
this.error,
this.fileId,
this.mtaId,
this.instanceIndex);
}
/**
* Copy the current immutable object by setting a value for the {@link AsyncUploadJobEntry#getSpaceGuid() spaceGuid} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for spaceGuid
* @return A modified copy of the {@code this} object
*/
public final ImmutableAsyncUploadJobEntry withSpaceGuid(String value) {
String newValue = Objects.requireNonNull(value, "spaceGuid");
if (this.spaceGuid.equals(newValue)) return this;
return new ImmutableAsyncUploadJobEntry(
this.id,
this.state,
this.user,
this.url,
this.addedAt,
this.startedAt,
this.finishedAt,
newValue,
this.namespace,
this.error,
this.fileId,
this.mtaId,
this.instanceIndex);
}
/**
* Copy the current immutable object by setting a value for the {@link AsyncUploadJobEntry#getNamespace() namespace} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for namespace (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableAsyncUploadJobEntry withNamespace(@Nullable String value) {
if (Objects.equals(this.namespace, value)) return this;
return new ImmutableAsyncUploadJobEntry(
this.id,
this.state,
this.user,
this.url,
this.addedAt,
this.startedAt,
this.finishedAt,
this.spaceGuid,
value,
this.error,
this.fileId,
this.mtaId,
this.instanceIndex);
}
/**
* Copy the current immutable object by setting a value for the {@link AsyncUploadJobEntry#getError() error} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for error (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableAsyncUploadJobEntry withError(@Nullable String value) {
if (Objects.equals(this.error, value)) return this;
return new ImmutableAsyncUploadJobEntry(
this.id,
this.state,
this.user,
this.url,
this.addedAt,
this.startedAt,
this.finishedAt,
this.spaceGuid,
this.namespace,
value,
this.fileId,
this.mtaId,
this.instanceIndex);
}
/**
* Copy the current immutable object by setting a value for the {@link AsyncUploadJobEntry#getFileId() fileId} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for fileId (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableAsyncUploadJobEntry withFileId(@Nullable String value) {
if (Objects.equals(this.fileId, value)) return this;
return new ImmutableAsyncUploadJobEntry(
this.id,
this.state,
this.user,
this.url,
this.addedAt,
this.startedAt,
this.finishedAt,
this.spaceGuid,
this.namespace,
this.error,
value,
this.mtaId,
this.instanceIndex);
}
/**
* Copy the current immutable object by setting a value for the {@link AsyncUploadJobEntry#getMtaId() mtaId} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for mtaId (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableAsyncUploadJobEntry withMtaId(@Nullable String value) {
if (Objects.equals(this.mtaId, value)) return this;
return new ImmutableAsyncUploadJobEntry(
this.id,
this.state,
this.user,
this.url,
this.addedAt,
this.startedAt,
this.finishedAt,
this.spaceGuid,
this.namespace,
this.error,
this.fileId,
value,
this.instanceIndex);
}
/**
* Copy the current immutable object by setting a value for the {@link AsyncUploadJobEntry#getInstanceIndex() instanceIndex} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for instanceIndex
* @return A modified copy of the {@code this} object
*/
public final ImmutableAsyncUploadJobEntry withInstanceIndex(Integer value) {
Integer newValue = Objects.requireNonNull(value, "instanceIndex");
if (this.instanceIndex.equals(newValue)) return this;
return new ImmutableAsyncUploadJobEntry(
this.id,
this.state,
this.user,
this.url,
this.addedAt,
this.startedAt,
this.finishedAt,
this.spaceGuid,
this.namespace,
this.error,
this.fileId,
this.mtaId,
newValue);
}
/**
* This instance is equal to all instances of {@code ImmutableAsyncUploadJobEntry} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(Object another) {
if (this == another) return true;
return another instanceof ImmutableAsyncUploadJobEntry
&& equalTo(0, (ImmutableAsyncUploadJobEntry) another);
}
private boolean equalTo(int synthetic, ImmutableAsyncUploadJobEntry another) {
return id.equals(another.id)
&& state.equals(another.state)
&& user.equals(another.user)
&& url.equals(another.url)
&& Objects.equals(addedAt, another.addedAt)
&& Objects.equals(startedAt, another.startedAt)
&& Objects.equals(finishedAt, another.finishedAt)
&& spaceGuid.equals(another.spaceGuid)
&& Objects.equals(namespace, another.namespace)
&& Objects.equals(error, another.error)
&& Objects.equals(fileId, another.fileId)
&& Objects.equals(mtaId, another.mtaId)
&& instanceIndex.equals(another.instanceIndex);
}
/**
* Computes a hash code from attributes: {@code id}, {@code state}, {@code user}, {@code url}, {@code addedAt}, {@code startedAt}, {@code finishedAt}, {@code spaceGuid}, {@code namespace}, {@code error}, {@code fileId}, {@code mtaId}, {@code instanceIndex}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 5381;
h += (h << 5) + id.hashCode();
h += (h << 5) + state.hashCode();
h += (h << 5) + user.hashCode();
h += (h << 5) + url.hashCode();
h += (h << 5) + Objects.hashCode(addedAt);
h += (h << 5) + Objects.hashCode(startedAt);
h += (h << 5) + Objects.hashCode(finishedAt);
h += (h << 5) + spaceGuid.hashCode();
h += (h << 5) + Objects.hashCode(namespace);
h += (h << 5) + Objects.hashCode(error);
h += (h << 5) + Objects.hashCode(fileId);
h += (h << 5) + Objects.hashCode(mtaId);
h += (h << 5) + instanceIndex.hashCode();
return h;
}
/**
* Prints the immutable value {@code AsyncUploadJobEntry} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
return "AsyncUploadJobEntry{"
+ "id=" + id
+ ", state=" + state
+ ", user=" + user
+ ", url=" + url
+ ", addedAt=" + addedAt
+ ", startedAt=" + startedAt
+ ", finishedAt=" + finishedAt
+ ", spaceGuid=" + spaceGuid
+ ", namespace=" + namespace
+ ", error=" + error
+ ", fileId=" + fileId
+ ", mtaId=" + mtaId
+ ", instanceIndex=" + instanceIndex
+ "}";
}
/**
* Creates an immutable copy of a {@link AsyncUploadJobEntry} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable AsyncUploadJobEntry instance
*/
public static ImmutableAsyncUploadJobEntry copyOf(AsyncUploadJobEntry instance) {
if (instance instanceof ImmutableAsyncUploadJobEntry) {
return (ImmutableAsyncUploadJobEntry) instance;
}
return ImmutableAsyncUploadJobEntry.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link ImmutableAsyncUploadJobEntry ImmutableAsyncUploadJobEntry}.
*
* ImmutableAsyncUploadJobEntry.builder()
* .id(String) // required {@link AsyncUploadJobEntry#getId() id}
* .state(org.cloudfoundry.multiapps.controller.persistence.model.AsyncUploadJobEntry.State) // required {@link AsyncUploadJobEntry#getState() state}
* .user(String) // required {@link AsyncUploadJobEntry#getUser() user}
* .url(String) // required {@link AsyncUploadJobEntry#getUrl() url}
* .addedAt(java.time.LocalDateTime | null) // nullable {@link AsyncUploadJobEntry#getAddedAt() addedAt}
* .startedAt(java.time.LocalDateTime | null) // nullable {@link AsyncUploadJobEntry#getStartedAt() startedAt}
* .finishedAt(java.time.LocalDateTime | null) // nullable {@link AsyncUploadJobEntry#getFinishedAt() finishedAt}
* .spaceGuid(String) // required {@link AsyncUploadJobEntry#getSpaceGuid() spaceGuid}
* .namespace(String | null) // nullable {@link AsyncUploadJobEntry#getNamespace() namespace}
* .error(String | null) // nullable {@link AsyncUploadJobEntry#getError() error}
* .fileId(String | null) // nullable {@link AsyncUploadJobEntry#getFileId() fileId}
* .mtaId(String | null) // nullable {@link AsyncUploadJobEntry#getMtaId() mtaId}
* .instanceIndex(Integer) // required {@link AsyncUploadJobEntry#getInstanceIndex() instanceIndex}
* .build();
*
* @return A new ImmutableAsyncUploadJobEntry builder
*/
public static ImmutableAsyncUploadJobEntry.Builder builder() {
return new ImmutableAsyncUploadJobEntry.Builder();
}
/**
* Builds instances of type {@link ImmutableAsyncUploadJobEntry ImmutableAsyncUploadJobEntry}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
public static final class Builder {
private static final long INIT_BIT_ID = 0x1L;
private static final long INIT_BIT_STATE = 0x2L;
private static final long INIT_BIT_USER = 0x4L;
private static final long INIT_BIT_URL = 0x8L;
private static final long INIT_BIT_SPACE_GUID = 0x10L;
private static final long INIT_BIT_INSTANCE_INDEX = 0x20L;
private long initBits = 0x3fL;
private String id;
private AsyncUploadJobEntry.State state;
private String user;
private String url;
private LocalDateTime addedAt;
private LocalDateTime startedAt;
private LocalDateTime finishedAt;
private String spaceGuid;
private String namespace;
private String error;
private String fileId;
private String mtaId;
private Integer instanceIndex;
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code AsyncUploadJobEntry} instance.
* Regular attribute values will be replaced with those from the given instance.
* Absent optional values will not replace present values.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(AsyncUploadJobEntry instance) {
Objects.requireNonNull(instance, "instance");
this.id(instance.getId());
this.state(instance.getState());
this.user(instance.getUser());
this.url(instance.getUrl());
@Nullable LocalDateTime addedAtValue = instance.getAddedAt();
if (addedAtValue != null) {
addedAt(addedAtValue);
}
@Nullable LocalDateTime startedAtValue = instance.getStartedAt();
if (startedAtValue != null) {
startedAt(startedAtValue);
}
@Nullable LocalDateTime finishedAtValue = instance.getFinishedAt();
if (finishedAtValue != null) {
finishedAt(finishedAtValue);
}
this.spaceGuid(instance.getSpaceGuid());
@Nullable String namespaceValue = instance.getNamespace();
if (namespaceValue != null) {
namespace(namespaceValue);
}
@Nullable String errorValue = instance.getError();
if (errorValue != null) {
error(errorValue);
}
@Nullable String fileIdValue = instance.getFileId();
if (fileIdValue != null) {
fileId(fileIdValue);
}
@Nullable String mtaIdValue = instance.getMtaId();
if (mtaIdValue != null) {
mtaId(mtaIdValue);
}
this.instanceIndex(instance.getInstanceIndex());
return this;
}
/**
* Initializes the value for the {@link AsyncUploadJobEntry#getId() id} attribute.
* @param id The value for id
* @return {@code this} builder for use in a chained invocation
*/
public final Builder id(String id) {
this.id = Objects.requireNonNull(id, "id");
initBits &= ~INIT_BIT_ID;
return this;
}
/**
* Initializes the value for the {@link AsyncUploadJobEntry#getState() state} attribute.
* @param state The value for state
* @return {@code this} builder for use in a chained invocation
*/
public final Builder state(AsyncUploadJobEntry.State state) {
this.state = Objects.requireNonNull(state, "state");
initBits &= ~INIT_BIT_STATE;
return this;
}
/**
* Initializes the value for the {@link AsyncUploadJobEntry#getUser() user} attribute.
* @param user The value for user
* @return {@code this} builder for use in a chained invocation
*/
public final Builder user(String user) {
this.user = Objects.requireNonNull(user, "user");
initBits &= ~INIT_BIT_USER;
return this;
}
/**
* Initializes the value for the {@link AsyncUploadJobEntry#getUrl() url} attribute.
* @param url The value for url
* @return {@code this} builder for use in a chained invocation
*/
public final Builder url(String url) {
this.url = Objects.requireNonNull(url, "url");
initBits &= ~INIT_BIT_URL;
return this;
}
/**
* Initializes the value for the {@link AsyncUploadJobEntry#getAddedAt() addedAt} attribute.
* @param addedAt The value for addedAt (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addedAt(@Nullable LocalDateTime addedAt) {
this.addedAt = addedAt;
return this;
}
/**
* Initializes the value for the {@link AsyncUploadJobEntry#getStartedAt() startedAt} attribute.
* @param startedAt The value for startedAt (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
public final Builder startedAt(@Nullable LocalDateTime startedAt) {
this.startedAt = startedAt;
return this;
}
/**
* Initializes the value for the {@link AsyncUploadJobEntry#getFinishedAt() finishedAt} attribute.
* @param finishedAt The value for finishedAt (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
public final Builder finishedAt(@Nullable LocalDateTime finishedAt) {
this.finishedAt = finishedAt;
return this;
}
/**
* Initializes the value for the {@link AsyncUploadJobEntry#getSpaceGuid() spaceGuid} attribute.
* @param spaceGuid The value for spaceGuid
* @return {@code this} builder for use in a chained invocation
*/
public final Builder spaceGuid(String spaceGuid) {
this.spaceGuid = Objects.requireNonNull(spaceGuid, "spaceGuid");
initBits &= ~INIT_BIT_SPACE_GUID;
return this;
}
/**
* Initializes the value for the {@link AsyncUploadJobEntry#getNamespace() namespace} attribute.
* @param namespace The value for namespace (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
public final Builder namespace(@Nullable String namespace) {
this.namespace = namespace;
return this;
}
/**
* Initializes the value for the {@link AsyncUploadJobEntry#getError() error} attribute.
* @param error The value for error (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
public final Builder error(@Nullable String error) {
this.error = error;
return this;
}
/**
* Initializes the value for the {@link AsyncUploadJobEntry#getFileId() fileId} attribute.
* @param fileId The value for fileId (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
public final Builder fileId(@Nullable String fileId) {
this.fileId = fileId;
return this;
}
/**
* Initializes the value for the {@link AsyncUploadJobEntry#getMtaId() mtaId} attribute.
* @param mtaId The value for mtaId (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
public final Builder mtaId(@Nullable String mtaId) {
this.mtaId = mtaId;
return this;
}
/**
* Initializes the value for the {@link AsyncUploadJobEntry#getInstanceIndex() instanceIndex} attribute.
* @param instanceIndex The value for instanceIndex
* @return {@code this} builder for use in a chained invocation
*/
public final Builder instanceIndex(Integer instanceIndex) {
this.instanceIndex = Objects.requireNonNull(instanceIndex, "instanceIndex");
initBits &= ~INIT_BIT_INSTANCE_INDEX;
return this;
}
/**
* Builds a new {@link ImmutableAsyncUploadJobEntry ImmutableAsyncUploadJobEntry}.
* @return An immutable instance of AsyncUploadJobEntry
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public ImmutableAsyncUploadJobEntry build() {
if (initBits != 0) {
throw new IllegalStateException(formatRequiredAttributesMessage());
}
return new ImmutableAsyncUploadJobEntry(
id,
state,
user,
url,
addedAt,
startedAt,
finishedAt,
spaceGuid,
namespace,
error,
fileId,
mtaId,
instanceIndex);
}
private String formatRequiredAttributesMessage() {
List attributes = new ArrayList<>();
if ((initBits & INIT_BIT_ID) != 0) attributes.add("id");
if ((initBits & INIT_BIT_STATE) != 0) attributes.add("state");
if ((initBits & INIT_BIT_USER) != 0) attributes.add("user");
if ((initBits & INIT_BIT_URL) != 0) attributes.add("url");
if ((initBits & INIT_BIT_SPACE_GUID) != 0) attributes.add("spaceGuid");
if ((initBits & INIT_BIT_INSTANCE_INDEX) != 0) attributes.add("instanceIndex");
return "Cannot build AsyncUploadJobEntry, some of required attributes are not set " + attributes;
}
}
}