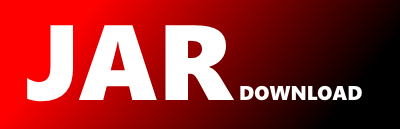
org.cloudfoundry.multiapps.controller.process.context.ImmutableApplicationToUploadContext Maven / Gradle / Ivy
package org.cloudfoundry.multiapps.controller.process.context;
import com.fasterxml.jackson.annotation.JsonAutoDetect;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import org.cloudfoundry.Nullable;
import org.cloudfoundry.multiapps.controller.client.lib.domain.CloudApplicationExtended;
import org.cloudfoundry.multiapps.controller.process.util.ArchiveEntryWithStreamPositions;
import org.cloudfoundry.multiapps.controller.process.util.StepLogger;
/**
* Immutable implementation of {@link ApplicationToUploadContext}.
*
* Use the builder to create immutable instances:
* {@code ImmutableApplicationToUploadContext.builder()}.
*/
@SuppressWarnings({"all"})
public final class ImmutableApplicationToUploadContext
implements ApplicationToUploadContext {
private final StepLogger stepLogger;
private final CloudApplicationExtended application;
private final String moduleFileName;
private final String spaceGuid;
private final String correlationId;
private final String taskId;
private final String appArchiveId;
private final @Nullable List archiveEntries;
private ImmutableApplicationToUploadContext(
StepLogger stepLogger,
CloudApplicationExtended application,
String moduleFileName,
String spaceGuid,
String correlationId,
String taskId,
String appArchiveId,
@Nullable List archiveEntries) {
this.stepLogger = stepLogger;
this.application = application;
this.moduleFileName = moduleFileName;
this.spaceGuid = spaceGuid;
this.correlationId = correlationId;
this.taskId = taskId;
this.appArchiveId = appArchiveId;
this.archiveEntries = archiveEntries;
}
/**
* @return The value of the {@code stepLogger} attribute
*/
@JsonProperty("stepLogger")
@Override
public StepLogger getStepLogger() {
return stepLogger;
}
/**
* @return The value of the {@code application} attribute
*/
@JsonProperty("application")
@Override
public CloudApplicationExtended getApplication() {
return application;
}
/**
* @return The value of the {@code moduleFileName} attribute
*/
@JsonProperty("moduleFileName")
@Override
public String getModuleFileName() {
return moduleFileName;
}
/**
* @return The value of the {@code spaceGuid} attribute
*/
@JsonProperty("spaceGuid")
@Override
public String getSpaceGuid() {
return spaceGuid;
}
/**
* @return The value of the {@code correlationId} attribute
*/
@JsonProperty("correlationId")
@Override
public String getCorrelationId() {
return correlationId;
}
/**
* @return The value of the {@code taskId} attribute
*/
@JsonProperty("taskId")
@Override
public String getTaskId() {
return taskId;
}
/**
* @return The value of the {@code appArchiveId} attribute
*/
@JsonProperty("appArchiveId")
@Override
public String getAppArchiveId() {
return appArchiveId;
}
/**
* @return The value of the {@code archiveEntries} attribute
*/
@JsonProperty("archiveEntries")
@Override
public @Nullable List getArchiveEntries() {
return archiveEntries;
}
/**
* Copy the current immutable object by setting a value for the {@link ApplicationToUploadContext#getStepLogger() stepLogger} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for stepLogger
* @return A modified copy of the {@code this} object
*/
public final ImmutableApplicationToUploadContext withStepLogger(StepLogger value) {
if (this.stepLogger == value) return this;
StepLogger newValue = Objects.requireNonNull(value, "stepLogger");
return new ImmutableApplicationToUploadContext(
newValue,
this.application,
this.moduleFileName,
this.spaceGuid,
this.correlationId,
this.taskId,
this.appArchiveId,
this.archiveEntries);
}
/**
* Copy the current immutable object by setting a value for the {@link ApplicationToUploadContext#getApplication() application} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for application
* @return A modified copy of the {@code this} object
*/
public final ImmutableApplicationToUploadContext withApplication(CloudApplicationExtended value) {
if (this.application == value) return this;
CloudApplicationExtended newValue = Objects.requireNonNull(value, "application");
return new ImmutableApplicationToUploadContext(
this.stepLogger,
newValue,
this.moduleFileName,
this.spaceGuid,
this.correlationId,
this.taskId,
this.appArchiveId,
this.archiveEntries);
}
/**
* Copy the current immutable object by setting a value for the {@link ApplicationToUploadContext#getModuleFileName() moduleFileName} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for moduleFileName
* @return A modified copy of the {@code this} object
*/
public final ImmutableApplicationToUploadContext withModuleFileName(String value) {
String newValue = Objects.requireNonNull(value, "moduleFileName");
if (this.moduleFileName.equals(newValue)) return this;
return new ImmutableApplicationToUploadContext(
this.stepLogger,
this.application,
newValue,
this.spaceGuid,
this.correlationId,
this.taskId,
this.appArchiveId,
this.archiveEntries);
}
/**
* Copy the current immutable object by setting a value for the {@link ApplicationToUploadContext#getSpaceGuid() spaceGuid} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for spaceGuid
* @return A modified copy of the {@code this} object
*/
public final ImmutableApplicationToUploadContext withSpaceGuid(String value) {
String newValue = Objects.requireNonNull(value, "spaceGuid");
if (this.spaceGuid.equals(newValue)) return this;
return new ImmutableApplicationToUploadContext(
this.stepLogger,
this.application,
this.moduleFileName,
newValue,
this.correlationId,
this.taskId,
this.appArchiveId,
this.archiveEntries);
}
/**
* Copy the current immutable object by setting a value for the {@link ApplicationToUploadContext#getCorrelationId() correlationId} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for correlationId
* @return A modified copy of the {@code this} object
*/
public final ImmutableApplicationToUploadContext withCorrelationId(String value) {
String newValue = Objects.requireNonNull(value, "correlationId");
if (this.correlationId.equals(newValue)) return this;
return new ImmutableApplicationToUploadContext(
this.stepLogger,
this.application,
this.moduleFileName,
this.spaceGuid,
newValue,
this.taskId,
this.appArchiveId,
this.archiveEntries);
}
/**
* Copy the current immutable object by setting a value for the {@link ApplicationToUploadContext#getTaskId() taskId} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for taskId
* @return A modified copy of the {@code this} object
*/
public final ImmutableApplicationToUploadContext withTaskId(String value) {
String newValue = Objects.requireNonNull(value, "taskId");
if (this.taskId.equals(newValue)) return this;
return new ImmutableApplicationToUploadContext(
this.stepLogger,
this.application,
this.moduleFileName,
this.spaceGuid,
this.correlationId,
newValue,
this.appArchiveId,
this.archiveEntries);
}
/**
* Copy the current immutable object by setting a value for the {@link ApplicationToUploadContext#getAppArchiveId() appArchiveId} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for appArchiveId
* @return A modified copy of the {@code this} object
*/
public final ImmutableApplicationToUploadContext withAppArchiveId(String value) {
String newValue = Objects.requireNonNull(value, "appArchiveId");
if (this.appArchiveId.equals(newValue)) return this;
return new ImmutableApplicationToUploadContext(
this.stepLogger,
this.application,
this.moduleFileName,
this.spaceGuid,
this.correlationId,
this.taskId,
newValue,
this.archiveEntries);
}
/**
* Copy the current immutable object with elements that replace the content of {@link ApplicationToUploadContext#getArchiveEntries() archiveEntries}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableApplicationToUploadContext withArchiveEntries(@Nullable ArchiveEntryWithStreamPositions... elements) {
if (elements == null) {
return new ImmutableApplicationToUploadContext(
this.stepLogger,
this.application,
this.moduleFileName,
this.spaceGuid,
this.correlationId,
this.taskId,
this.appArchiveId,
null);
}
List newValue = Arrays.asList(elements) == null ? null : createUnmodifiableList(false, createSafeList(Arrays.asList(elements), true, false));
return new ImmutableApplicationToUploadContext(
this.stepLogger,
this.application,
this.moduleFileName,
this.spaceGuid,
this.correlationId,
this.taskId,
this.appArchiveId,
newValue);
}
/**
* Copy the current immutable object with elements that replace the content of {@link ApplicationToUploadContext#getArchiveEntries() archiveEntries}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of archiveEntries elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableApplicationToUploadContext withArchiveEntries(@Nullable Iterable extends ArchiveEntryWithStreamPositions> elements) {
if (this.archiveEntries == elements) return this;
List newValue = elements == null ? null : createUnmodifiableList(false, createSafeList(elements, true, false));
return new ImmutableApplicationToUploadContext(
this.stepLogger,
this.application,
this.moduleFileName,
this.spaceGuid,
this.correlationId,
this.taskId,
this.appArchiveId,
newValue);
}
/**
* This instance is equal to all instances of {@code ImmutableApplicationToUploadContext} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(Object another) {
if (this == another) return true;
return another instanceof ImmutableApplicationToUploadContext
&& equalTo(0, (ImmutableApplicationToUploadContext) another);
}
private boolean equalTo(int synthetic, ImmutableApplicationToUploadContext another) {
return stepLogger.equals(another.stepLogger)
&& application.equals(another.application)
&& moduleFileName.equals(another.moduleFileName)
&& spaceGuid.equals(another.spaceGuid)
&& correlationId.equals(another.correlationId)
&& taskId.equals(another.taskId)
&& appArchiveId.equals(another.appArchiveId)
&& Objects.equals(archiveEntries, another.archiveEntries);
}
/**
* Computes a hash code from attributes: {@code stepLogger}, {@code application}, {@code moduleFileName}, {@code spaceGuid}, {@code correlationId}, {@code taskId}, {@code appArchiveId}, {@code archiveEntries}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 5381;
h += (h << 5) + stepLogger.hashCode();
h += (h << 5) + application.hashCode();
h += (h << 5) + moduleFileName.hashCode();
h += (h << 5) + spaceGuid.hashCode();
h += (h << 5) + correlationId.hashCode();
h += (h << 5) + taskId.hashCode();
h += (h << 5) + appArchiveId.hashCode();
h += (h << 5) + Objects.hashCode(archiveEntries);
return h;
}
/**
* Prints the immutable value {@code ApplicationToUploadContext} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
return "ApplicationToUploadContext{"
+ "stepLogger=" + stepLogger
+ ", application=" + application
+ ", moduleFileName=" + moduleFileName
+ ", spaceGuid=" + spaceGuid
+ ", correlationId=" + correlationId
+ ", taskId=" + taskId
+ ", appArchiveId=" + appArchiveId
+ ", archiveEntries=" + archiveEntries
+ "}";
}
/**
* Utility type used to correctly read immutable object from JSON representation.
* @deprecated Do not use this type directly, it exists only for the Jackson-binding infrastructure
*/
@Deprecated
@JsonDeserialize
@JsonAutoDetect(fieldVisibility = JsonAutoDetect.Visibility.NONE)
static final class Json
implements ApplicationToUploadContext {
StepLogger stepLogger;
CloudApplicationExtended application;
String moduleFileName;
String spaceGuid;
String correlationId;
String taskId;
String appArchiveId;
List archiveEntries = null;
@JsonProperty("stepLogger")
public void setStepLogger(StepLogger stepLogger) {
this.stepLogger = stepLogger;
}
@JsonProperty("application")
public void setApplication(CloudApplicationExtended application) {
this.application = application;
}
@JsonProperty("moduleFileName")
public void setModuleFileName(String moduleFileName) {
this.moduleFileName = moduleFileName;
}
@JsonProperty("spaceGuid")
public void setSpaceGuid(String spaceGuid) {
this.spaceGuid = spaceGuid;
}
@JsonProperty("correlationId")
public void setCorrelationId(String correlationId) {
this.correlationId = correlationId;
}
@JsonProperty("taskId")
public void setTaskId(String taskId) {
this.taskId = taskId;
}
@JsonProperty("appArchiveId")
public void setAppArchiveId(String appArchiveId) {
this.appArchiveId = appArchiveId;
}
@JsonProperty("archiveEntries")
public void setArchiveEntries(@Nullable List archiveEntries) {
this.archiveEntries = archiveEntries;
}
@Override
public StepLogger getStepLogger() { throw new UnsupportedOperationException(); }
@Override
public CloudApplicationExtended getApplication() { throw new UnsupportedOperationException(); }
@Override
public String getModuleFileName() { throw new UnsupportedOperationException(); }
@Override
public String getSpaceGuid() { throw new UnsupportedOperationException(); }
@Override
public String getCorrelationId() { throw new UnsupportedOperationException(); }
@Override
public String getTaskId() { throw new UnsupportedOperationException(); }
@Override
public String getAppArchiveId() { throw new UnsupportedOperationException(); }
@Override
public List getArchiveEntries() { throw new UnsupportedOperationException(); }
}
/**
* @param json A JSON-bindable data structure
* @return An immutable value type
* @deprecated Do not use this method directly, it exists only for the Jackson-binding infrastructure
*/
@Deprecated
@JsonCreator(mode = JsonCreator.Mode.DELEGATING)
static ImmutableApplicationToUploadContext fromJson(Json json) {
ImmutableApplicationToUploadContext.Builder builder = ImmutableApplicationToUploadContext.builder();
if (json.stepLogger != null) {
builder.stepLogger(json.stepLogger);
}
if (json.application != null) {
builder.application(json.application);
}
if (json.moduleFileName != null) {
builder.moduleFileName(json.moduleFileName);
}
if (json.spaceGuid != null) {
builder.spaceGuid(json.spaceGuid);
}
if (json.correlationId != null) {
builder.correlationId(json.correlationId);
}
if (json.taskId != null) {
builder.taskId(json.taskId);
}
if (json.appArchiveId != null) {
builder.appArchiveId(json.appArchiveId);
}
if (json.archiveEntries != null) {
builder.addAllArchiveEntries(json.archiveEntries);
}
return builder.build();
}
/**
* Creates an immutable copy of a {@link ApplicationToUploadContext} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable ApplicationToUploadContext instance
*/
public static ImmutableApplicationToUploadContext copyOf(ApplicationToUploadContext instance) {
if (instance instanceof ImmutableApplicationToUploadContext) {
return (ImmutableApplicationToUploadContext) instance;
}
return ImmutableApplicationToUploadContext.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link ImmutableApplicationToUploadContext ImmutableApplicationToUploadContext}.
*
* ImmutableApplicationToUploadContext.builder()
* .stepLogger(org.cloudfoundry.multiapps.controller.process.util.StepLogger) // required {@link ApplicationToUploadContext#getStepLogger() stepLogger}
* .application(org.cloudfoundry.multiapps.controller.client.lib.domain.CloudApplicationExtended) // required {@link ApplicationToUploadContext#getApplication() application}
* .moduleFileName(String) // required {@link ApplicationToUploadContext#getModuleFileName() moduleFileName}
* .spaceGuid(String) // required {@link ApplicationToUploadContext#getSpaceGuid() spaceGuid}
* .correlationId(String) // required {@link ApplicationToUploadContext#getCorrelationId() correlationId}
* .taskId(String) // required {@link ApplicationToUploadContext#getTaskId() taskId}
* .appArchiveId(String) // required {@link ApplicationToUploadContext#getAppArchiveId() appArchiveId}
* .archiveEntries(List<org.cloudfoundry.multiapps.controller.process.util.ArchiveEntryWithStreamPositions> | null) // nullable {@link ApplicationToUploadContext#getArchiveEntries() archiveEntries}
* .build();
*
* @return A new ImmutableApplicationToUploadContext builder
*/
public static ImmutableApplicationToUploadContext.Builder builder() {
return new ImmutableApplicationToUploadContext.Builder();
}
/**
* Builds instances of type {@link ImmutableApplicationToUploadContext ImmutableApplicationToUploadContext}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
public static final class Builder {
private static final long INIT_BIT_STEP_LOGGER = 0x1L;
private static final long INIT_BIT_APPLICATION = 0x2L;
private static final long INIT_BIT_MODULE_FILE_NAME = 0x4L;
private static final long INIT_BIT_SPACE_GUID = 0x8L;
private static final long INIT_BIT_CORRELATION_ID = 0x10L;
private static final long INIT_BIT_TASK_ID = 0x20L;
private static final long INIT_BIT_APP_ARCHIVE_ID = 0x40L;
private long initBits = 0x7fL;
private StepLogger stepLogger;
private CloudApplicationExtended application;
private String moduleFileName;
private String spaceGuid;
private String correlationId;
private String taskId;
private String appArchiveId;
private List archiveEntries = null;
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code ApplicationToUploadContext} instance.
* Regular attribute values will be replaced with those from the given instance.
* Absent optional values will not replace present values.
* Collection elements and entries will be added, not replaced.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(ApplicationToUploadContext instance) {
Objects.requireNonNull(instance, "instance");
this.stepLogger(instance.getStepLogger());
this.application(instance.getApplication());
this.moduleFileName(instance.getModuleFileName());
this.spaceGuid(instance.getSpaceGuid());
this.correlationId(instance.getCorrelationId());
this.taskId(instance.getTaskId());
this.appArchiveId(instance.getAppArchiveId());
List archiveEntriesValue = instance.getArchiveEntries();
if (archiveEntriesValue != null) {
addAllArchiveEntries(archiveEntriesValue);
}
return this;
}
/**
* Initializes the value for the {@link ApplicationToUploadContext#getStepLogger() stepLogger} attribute.
* @param stepLogger The value for stepLogger
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("stepLogger")
public final Builder stepLogger(StepLogger stepLogger) {
this.stepLogger = Objects.requireNonNull(stepLogger, "stepLogger");
initBits &= ~INIT_BIT_STEP_LOGGER;
return this;
}
/**
* Initializes the value for the {@link ApplicationToUploadContext#getApplication() application} attribute.
* @param application The value for application
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("application")
public final Builder application(CloudApplicationExtended application) {
this.application = Objects.requireNonNull(application, "application");
initBits &= ~INIT_BIT_APPLICATION;
return this;
}
/**
* Initializes the value for the {@link ApplicationToUploadContext#getModuleFileName() moduleFileName} attribute.
* @param moduleFileName The value for moduleFileName
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("moduleFileName")
public final Builder moduleFileName(String moduleFileName) {
this.moduleFileName = Objects.requireNonNull(moduleFileName, "moduleFileName");
initBits &= ~INIT_BIT_MODULE_FILE_NAME;
return this;
}
/**
* Initializes the value for the {@link ApplicationToUploadContext#getSpaceGuid() spaceGuid} attribute.
* @param spaceGuid The value for spaceGuid
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("spaceGuid")
public final Builder spaceGuid(String spaceGuid) {
this.spaceGuid = Objects.requireNonNull(spaceGuid, "spaceGuid");
initBits &= ~INIT_BIT_SPACE_GUID;
return this;
}
/**
* Initializes the value for the {@link ApplicationToUploadContext#getCorrelationId() correlationId} attribute.
* @param correlationId The value for correlationId
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("correlationId")
public final Builder correlationId(String correlationId) {
this.correlationId = Objects.requireNonNull(correlationId, "correlationId");
initBits &= ~INIT_BIT_CORRELATION_ID;
return this;
}
/**
* Initializes the value for the {@link ApplicationToUploadContext#getTaskId() taskId} attribute.
* @param taskId The value for taskId
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("taskId")
public final Builder taskId(String taskId) {
this.taskId = Objects.requireNonNull(taskId, "taskId");
initBits &= ~INIT_BIT_TASK_ID;
return this;
}
/**
* Initializes the value for the {@link ApplicationToUploadContext#getAppArchiveId() appArchiveId} attribute.
* @param appArchiveId The value for appArchiveId
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("appArchiveId")
public final Builder appArchiveId(String appArchiveId) {
this.appArchiveId = Objects.requireNonNull(appArchiveId, "appArchiveId");
initBits &= ~INIT_BIT_APP_ARCHIVE_ID;
return this;
}
/**
* Adds one element to {@link ApplicationToUploadContext#getArchiveEntries() archiveEntries} list.
* @param element A archiveEntries element
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addArchiveEntry(ArchiveEntryWithStreamPositions element) {
if (this.archiveEntries == null) {
this.archiveEntries = new ArrayList();
}
this.archiveEntries.add(Objects.requireNonNull(element, "archiveEntries element"));
return this;
}
/**
* Adds elements to {@link ApplicationToUploadContext#getArchiveEntries() archiveEntries} list.
* @param elements An array of archiveEntries elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addArchiveEntries(ArchiveEntryWithStreamPositions... elements) {
if (this.archiveEntries == null) {
this.archiveEntries = new ArrayList();
}
for (ArchiveEntryWithStreamPositions element : elements) {
this.archiveEntries.add(Objects.requireNonNull(element, "archiveEntries element"));
}
return this;
}
/**
* Sets or replaces all elements for {@link ApplicationToUploadContext#getArchiveEntries() archiveEntries} list.
* @param elements An iterable of archiveEntries elements
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("archiveEntries")
public final Builder archiveEntries(@Nullable Iterable extends ArchiveEntryWithStreamPositions> elements) {
if (elements == null) {
this.archiveEntries = null;
return this;
}
this.archiveEntries = new ArrayList();
return addAllArchiveEntries(elements);
}
/**
* Adds elements to {@link ApplicationToUploadContext#getArchiveEntries() archiveEntries} list.
* @param elements An iterable of archiveEntries elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addAllArchiveEntries(Iterable extends ArchiveEntryWithStreamPositions> elements) {
Objects.requireNonNull(elements, "archiveEntries element");
if (this.archiveEntries == null) {
this.archiveEntries = new ArrayList();
}
for (ArchiveEntryWithStreamPositions element : elements) {
this.archiveEntries.add(Objects.requireNonNull(element, "archiveEntries element"));
}
return this;
}
/**
* Builds a new {@link ImmutableApplicationToUploadContext ImmutableApplicationToUploadContext}.
* @return An immutable instance of ApplicationToUploadContext
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public ImmutableApplicationToUploadContext build() {
if (initBits != 0) {
throw new IllegalStateException(formatRequiredAttributesMessage());
}
return new ImmutableApplicationToUploadContext(
stepLogger,
application,
moduleFileName,
spaceGuid,
correlationId,
taskId,
appArchiveId,
archiveEntries == null ? null : createUnmodifiableList(true, archiveEntries));
}
private String formatRequiredAttributesMessage() {
List attributes = new ArrayList<>();
if ((initBits & INIT_BIT_STEP_LOGGER) != 0) attributes.add("stepLogger");
if ((initBits & INIT_BIT_APPLICATION) != 0) attributes.add("application");
if ((initBits & INIT_BIT_MODULE_FILE_NAME) != 0) attributes.add("moduleFileName");
if ((initBits & INIT_BIT_SPACE_GUID) != 0) attributes.add("spaceGuid");
if ((initBits & INIT_BIT_CORRELATION_ID) != 0) attributes.add("correlationId");
if ((initBits & INIT_BIT_TASK_ID) != 0) attributes.add("taskId");
if ((initBits & INIT_BIT_APP_ARCHIVE_ID) != 0) attributes.add("appArchiveId");
return "Cannot build ApplicationToUploadContext, some of required attributes are not set " + attributes;
}
}
private static List createSafeList(Iterable extends T> iterable, boolean checkNulls, boolean skipNulls) {
ArrayList list;
if (iterable instanceof Collection>) {
int size = ((Collection>) iterable).size();
if (size == 0) return Collections.emptyList();
list = new ArrayList<>(size);
} else {
list = new ArrayList<>();
}
for (T element : iterable) {
if (skipNulls && element == null) continue;
if (checkNulls) Objects.requireNonNull(element, "element");
list.add(element);
}
return list;
}
private static List createUnmodifiableList(boolean clone, List list) {
switch(list.size()) {
case 0: return Collections.emptyList();
case 1: return Collections.singletonList(list.get(0));
default:
if (clone) {
return Collections.unmodifiableList(new ArrayList<>(list));
} else {
if (list instanceof ArrayList>) {
((ArrayList>) list).trimToSize();
}
return Collections.unmodifiableList(list);
}
}
}
}