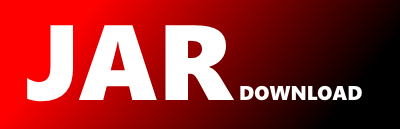
org.cloudfoundry.multiapps.controller.process.dynatrace.ImmutableDynatraceProcessEvent Maven / Gradle / Ivy
package org.cloudfoundry.multiapps.controller.process.dynatrace;
import com.fasterxml.jackson.annotation.JsonAutoDetect;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import com.fasterxml.jackson.databind.annotation.JsonSerialize;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
import org.cloudfoundry.multiapps.common.Nullable;
import org.cloudfoundry.multiapps.controller.api.model.ProcessType;
import org.cloudfoundry.multiapps.controller.api.model.ProcessTypeDeserializer;
import org.cloudfoundry.multiapps.controller.api.model.ProcessTypeSerializer;
/**
* Immutable implementation of {@link DynatraceProcessEvent}.
*
* Use the builder to create immutable instances:
* {@code ImmutableDynatraceProcessEvent.builder()}.
*/
@SuppressWarnings({"all"})
public final class ImmutableDynatraceProcessEvent
extends DynatraceProcessEvent {
private final @Nullable String processId;
private final @Nullable ProcessType processType;
private final @Nullable String spaceId;
private final @Nullable String mtaId;
private final DynatraceProcessEvent.EventType eventType;
private ImmutableDynatraceProcessEvent(
@Nullable String processId,
@Nullable ProcessType processType,
@Nullable String spaceId,
@Nullable String mtaId,
DynatraceProcessEvent.EventType eventType) {
this.processId = processId;
this.processType = processType;
this.spaceId = spaceId;
this.mtaId = mtaId;
this.eventType = eventType;
}
/**
* @return The value of the {@code processId} attribute
*/
@JsonProperty("processId")
@Override
public @Nullable String getProcessId() {
return processId;
}
/**
* @return The value of the {@code processType} attribute
*/
@JsonProperty("processType")
@JsonSerialize(using = ProcessTypeSerializer.class)
@JsonDeserialize(using = ProcessTypeDeserializer.class)
@Override
public @Nullable ProcessType getProcessType() {
return processType;
}
/**
* @return The value of the {@code spaceId} attribute
*/
@JsonProperty("spaceId")
@Override
public @Nullable String getSpaceId() {
return spaceId;
}
/**
* @return The value of the {@code mtaId} attribute
*/
@JsonProperty("mtaId")
@Override
public @Nullable String getMtaId() {
return mtaId;
}
/**
* @return The value of the {@code eventType} attribute
*/
@JsonProperty("eventType")
@Override
public DynatraceProcessEvent.EventType getEventType() {
return eventType;
}
/**
* Copy the current immutable object by setting a value for the {@link DynatraceProcessEvent#getProcessId() processId} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for processId (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableDynatraceProcessEvent withProcessId(@Nullable String value) {
if (Objects.equals(this.processId, value)) return this;
return new ImmutableDynatraceProcessEvent(value, this.processType, this.spaceId, this.mtaId, this.eventType);
}
/**
* Copy the current immutable object by setting a value for the {@link DynatraceProcessEvent#getProcessType() processType} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for processType (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableDynatraceProcessEvent withProcessType(@Nullable ProcessType value) {
if (this.processType == value) return this;
return new ImmutableDynatraceProcessEvent(this.processId, value, this.spaceId, this.mtaId, this.eventType);
}
/**
* Copy the current immutable object by setting a value for the {@link DynatraceProcessEvent#getSpaceId() spaceId} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for spaceId (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableDynatraceProcessEvent withSpaceId(@Nullable String value) {
if (Objects.equals(this.spaceId, value)) return this;
return new ImmutableDynatraceProcessEvent(this.processId, this.processType, value, this.mtaId, this.eventType);
}
/**
* Copy the current immutable object by setting a value for the {@link DynatraceProcessEvent#getMtaId() mtaId} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for mtaId (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableDynatraceProcessEvent withMtaId(@Nullable String value) {
if (Objects.equals(this.mtaId, value)) return this;
return new ImmutableDynatraceProcessEvent(this.processId, this.processType, this.spaceId, value, this.eventType);
}
/**
* Copy the current immutable object by setting a value for the {@link DynatraceProcessEvent#getEventType() eventType} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for eventType
* @return A modified copy of the {@code this} object
*/
public final ImmutableDynatraceProcessEvent withEventType(DynatraceProcessEvent.EventType value) {
DynatraceProcessEvent.EventType newValue = Objects.requireNonNull(value, "eventType");
if (this.eventType == newValue) return this;
return new ImmutableDynatraceProcessEvent(this.processId, this.processType, this.spaceId, this.mtaId, newValue);
}
/**
* This instance is equal to all instances of {@code ImmutableDynatraceProcessEvent} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(Object another) {
if (this == another) return true;
return another instanceof ImmutableDynatraceProcessEvent
&& equalTo(0, (ImmutableDynatraceProcessEvent) another);
}
private boolean equalTo(int synthetic, ImmutableDynatraceProcessEvent another) {
return Objects.equals(processId, another.processId)
&& Objects.equals(processType, another.processType)
&& Objects.equals(spaceId, another.spaceId)
&& Objects.equals(mtaId, another.mtaId)
&& eventType.equals(another.eventType);
}
/**
* Computes a hash code from attributes: {@code processId}, {@code processType}, {@code spaceId}, {@code mtaId}, {@code eventType}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 5381;
h += (h << 5) + Objects.hashCode(processId);
h += (h << 5) + Objects.hashCode(processType);
h += (h << 5) + Objects.hashCode(spaceId);
h += (h << 5) + Objects.hashCode(mtaId);
h += (h << 5) + eventType.hashCode();
return h;
}
/**
* Prints the immutable value {@code DynatraceProcessEvent} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
return "DynatraceProcessEvent{"
+ "processId=" + processId
+ ", processType=" + processType
+ ", spaceId=" + spaceId
+ ", mtaId=" + mtaId
+ ", eventType=" + eventType
+ "}";
}
/**
* Utility type used to correctly read immutable object from JSON representation.
* @deprecated Do not use this type directly, it exists only for the Jackson-binding infrastructure
*/
@Deprecated
@JsonDeserialize
@JsonAutoDetect(fieldVisibility = JsonAutoDetect.Visibility.NONE)
static final class Json
extends DynatraceProcessEvent {
String processId;
ProcessType processType;
String spaceId;
String mtaId;
DynatraceProcessEvent.EventType eventType;
@JsonProperty("processId")
public void setProcessId(@Nullable String processId) {
this.processId = processId;
}
@JsonProperty("processType")
@JsonSerialize(using = ProcessTypeSerializer.class)
@JsonDeserialize(using = ProcessTypeDeserializer.class)
public void setProcessType(@Nullable ProcessType processType) {
this.processType = processType;
}
@JsonProperty("spaceId")
public void setSpaceId(@Nullable String spaceId) {
this.spaceId = spaceId;
}
@JsonProperty("mtaId")
public void setMtaId(@Nullable String mtaId) {
this.mtaId = mtaId;
}
@JsonProperty("eventType")
public void setEventType(DynatraceProcessEvent.EventType eventType) {
this.eventType = eventType;
}
@Override
public String getProcessId() { throw new UnsupportedOperationException(); }
@Override
public ProcessType getProcessType() { throw new UnsupportedOperationException(); }
@Override
public String getSpaceId() { throw new UnsupportedOperationException(); }
@Override
public String getMtaId() { throw new UnsupportedOperationException(); }
@Override
public DynatraceProcessEvent.EventType getEventType() { throw new UnsupportedOperationException(); }
}
/**
* @param json A JSON-bindable data structure
* @return An immutable value type
* @deprecated Do not use this method directly, it exists only for the Jackson-binding infrastructure
*/
@Deprecated
@JsonCreator(mode = JsonCreator.Mode.DELEGATING)
static ImmutableDynatraceProcessEvent fromJson(Json json) {
ImmutableDynatraceProcessEvent.Builder builder = ImmutableDynatraceProcessEvent.builder();
if (json.processId != null) {
builder.processId(json.processId);
}
if (json.processType != null) {
builder.processType(json.processType);
}
if (json.spaceId != null) {
builder.spaceId(json.spaceId);
}
if (json.mtaId != null) {
builder.mtaId(json.mtaId);
}
if (json.eventType != null) {
builder.eventType(json.eventType);
}
return builder.build();
}
/**
* Creates an immutable copy of a {@link DynatraceProcessEvent} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable DynatraceProcessEvent instance
*/
public static ImmutableDynatraceProcessEvent copyOf(DynatraceProcessEvent instance) {
if (instance instanceof ImmutableDynatraceProcessEvent) {
return (ImmutableDynatraceProcessEvent) instance;
}
return ImmutableDynatraceProcessEvent.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link ImmutableDynatraceProcessEvent ImmutableDynatraceProcessEvent}.
*
* ImmutableDynatraceProcessEvent.builder()
* .processId(String | null) // nullable {@link DynatraceProcessEvent#getProcessId() processId}
* .processType(org.cloudfoundry.multiapps.controller.api.model.ProcessType | null) // nullable {@link DynatraceProcessEvent#getProcessType() processType}
* .spaceId(String | null) // nullable {@link DynatraceProcessEvent#getSpaceId() spaceId}
* .mtaId(String | null) // nullable {@link DynatraceProcessEvent#getMtaId() mtaId}
* .eventType(org.cloudfoundry.multiapps.controller.process.dynatrace.DynatraceProcessEvent.EventType) // required {@link DynatraceProcessEvent#getEventType() eventType}
* .build();
*
* @return A new ImmutableDynatraceProcessEvent builder
*/
public static ImmutableDynatraceProcessEvent.Builder builder() {
return new ImmutableDynatraceProcessEvent.Builder();
}
/**
* Builds instances of type {@link ImmutableDynatraceProcessEvent ImmutableDynatraceProcessEvent}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
public static final class Builder {
private static final long INIT_BIT_EVENT_TYPE = 0x1L;
private long initBits = 0x1L;
private String processId;
private ProcessType processType;
private String spaceId;
private String mtaId;
private DynatraceProcessEvent.EventType eventType;
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code org.cloudfoundry.multiapps.controller.process.dynatrace.DynatraceProcessEvent} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(DynatraceProcessEvent instance) {
Objects.requireNonNull(instance, "instance");
from((short) 0, (Object) instance);
return this;
}
/**
* Fill a builder with attribute values from the provided {@code org.cloudfoundry.multiapps.controller.process.dynatrace.DyntraceProcessEntity} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(DyntraceProcessEntity instance) {
Objects.requireNonNull(instance, "instance");
from((short) 0, (Object) instance);
return this;
}
private void from(short _unused, Object object) {
if (object instanceof DynatraceProcessEvent) {
DynatraceProcessEvent instance = (DynatraceProcessEvent) object;
this.eventType(instance.getEventType());
}
if (object instanceof DyntraceProcessEntity) {
DyntraceProcessEntity instance = (DyntraceProcessEntity) object;
@Nullable String spaceIdValue = instance.getSpaceId();
if (spaceIdValue != null) {
spaceId(spaceIdValue);
}
@Nullable String mtaIdValue = instance.getMtaId();
if (mtaIdValue != null) {
mtaId(mtaIdValue);
}
@Nullable ProcessType processTypeValue = instance.getProcessType();
if (processTypeValue != null) {
processType(processTypeValue);
}
@Nullable String processIdValue = instance.getProcessId();
if (processIdValue != null) {
processId(processIdValue);
}
}
}
/**
* Initializes the value for the {@link DynatraceProcessEvent#getProcessId() processId} attribute.
* @param processId The value for processId (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("processId")
public final Builder processId(@Nullable String processId) {
this.processId = processId;
return this;
}
/**
* Initializes the value for the {@link DynatraceProcessEvent#getProcessType() processType} attribute.
* @param processType The value for processType (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("processType")
@JsonSerialize(using = ProcessTypeSerializer.class)
@JsonDeserialize(using = ProcessTypeDeserializer.class)
public final Builder processType(@Nullable ProcessType processType) {
this.processType = processType;
return this;
}
/**
* Initializes the value for the {@link DynatraceProcessEvent#getSpaceId() spaceId} attribute.
* @param spaceId The value for spaceId (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("spaceId")
public final Builder spaceId(@Nullable String spaceId) {
this.spaceId = spaceId;
return this;
}
/**
* Initializes the value for the {@link DynatraceProcessEvent#getMtaId() mtaId} attribute.
* @param mtaId The value for mtaId (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("mtaId")
public final Builder mtaId(@Nullable String mtaId) {
this.mtaId = mtaId;
return this;
}
/**
* Initializes the value for the {@link DynatraceProcessEvent#getEventType() eventType} attribute.
* @param eventType The value for eventType
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("eventType")
public final Builder eventType(DynatraceProcessEvent.EventType eventType) {
this.eventType = Objects.requireNonNull(eventType, "eventType");
initBits &= ~INIT_BIT_EVENT_TYPE;
return this;
}
/**
* Builds a new {@link ImmutableDynatraceProcessEvent ImmutableDynatraceProcessEvent}.
* @return An immutable instance of DynatraceProcessEvent
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public ImmutableDynatraceProcessEvent build() {
if (initBits != 0) {
throw new IllegalStateException(formatRequiredAttributesMessage());
}
return new ImmutableDynatraceProcessEvent(processId, processType, spaceId, mtaId, eventType);
}
private String formatRequiredAttributesMessage() {
List attributes = new ArrayList<>();
if ((initBits & INIT_BIT_EVENT_TYPE) != 0) attributes.add("eventType");
return "Cannot build DynatraceProcessEvent, some of required attributes are not set " + attributes;
}
}
}