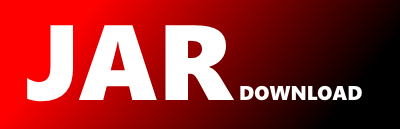
org.cloudfoundry.multiapps.controller.process.steps.DetectApplicationsToRenameStep Maven / Gradle / Ivy
package org.cloudfoundry.multiapps.controller.process.steps;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Set;
import java.util.stream.Collectors;
import jakarta.inject.Named;
import org.apache.commons.collections4.CollectionUtils;
import org.cloudfoundry.multiapps.controller.core.model.BlueGreenApplicationNameSuffix;
import org.cloudfoundry.multiapps.controller.core.model.DeployedMta;
import org.cloudfoundry.multiapps.controller.core.model.DeployedMtaApplication;
import org.cloudfoundry.multiapps.controller.core.model.ImmutableDeployedMta;
import org.cloudfoundry.multiapps.controller.core.model.ImmutableDeployedMtaApplication;
import org.cloudfoundry.multiapps.controller.core.util.CloudModelBuilderUtil;
import org.cloudfoundry.multiapps.controller.process.Messages;
import org.cloudfoundry.multiapps.controller.process.variables.Variables;
import org.springframework.beans.factory.config.BeanDefinition;
import org.springframework.context.annotation.Scope;
import com.sap.cloudfoundry.client.facade.CloudControllerClient;
import com.sap.cloudfoundry.client.facade.domain.CloudApplication;
@Named("detectApplicationsToRenameStep")
@Scope(BeanDefinition.SCOPE_PROTOTYPE)
public class DetectApplicationsToRenameStep extends SyncFlowableStep {
@Override
protected StepPhase executeStep(ProcessContext context) {
// This is set here in case of the step returning early or failing because the call activity
// following this step needs this variable, otherwise Flowable will throw an exception
context.setVariable(Variables.APPS_TO_UNDEPLOY, Collections.emptyList());
if (!context.getVariable(Variables.KEEP_ORIGINAL_APP_NAMES_AFTER_DEPLOY)) {
return StepPhase.DONE;
}
DeployedMta deployedMta = context.getVariable(Variables.DEPLOYED_MTA);
if (deployedMta == null) {
return StepPhase.DONE;
}
List selectedModules = context.getVariable(Variables.MODULES_FOR_DEPLOYMENT);
List appsToRename = computeOldAppsToRename(deployedMta, selectedModules);
List appsToUndeploy = computeLeftoverAppsToUndeploy(deployedMta);
context.setVariable(Variables.APPS_TO_RENAME, appsToRename);
setAppsToUndeploy(context, appsToUndeploy);
updateDeployedMta(context, deployedMta, appsToRename, appsToUndeploy);
return StepPhase.DONE;
}
private List computeOldAppsToRename(DeployedMta deployedMta, List selectedModules) {
return deployedMta.getApplications()
.stream()
.filter(app -> !BlueGreenApplicationNameSuffix.isSuffixContainedIn(app.getName()))
.filter(app -> isModuleSelectedForDeployment(app.getModuleName(), selectedModules))
.map(DeployedMtaApplication::getName)
.collect(Collectors.toList());
}
private boolean isModuleSelectedForDeployment(String moduleName, List selectedModules) {
if (CollectionUtils.isEmpty(selectedModules)) {
return true;
}
return selectedModules.contains(moduleName);
}
private List computeLeftoverAppsToUndeploy(DeployedMta deployedMta) {
Set appsToProcess = CloudModelBuilderUtil.getDeployedApplicationNames(deployedMta.getApplications());
return appsToProcess.stream()
.filter(appName -> appName.endsWith(BlueGreenApplicationNameSuffix.LIVE.asSuffix()))
.filter(appName -> appsToProcess.contains(BlueGreenApplicationNameSuffix.removeSuffix(appName)))
.collect(Collectors.toList());
}
private void setAppsToUndeploy(ProcessContext context, List appsToUndeploy) {
CloudControllerClient client = context.getControllerClient();
List apps = appsToUndeploy.stream()
.map(app -> client.getApplication(app, false))
.filter(Objects::nonNull)
.collect(Collectors.toList());
context.setVariable(Variables.APPS_TO_UNDEPLOY, apps);
}
private void updateDeployedMta(ProcessContext context, DeployedMta deployedMta, List appsToUpdate,
List appsToUndeploy) {
if (appsToUpdate.isEmpty()) {
return;
}
List apps = deployedMta.getApplications();
List updatedApps = apps.stream()
.filter(app -> !appsToUndeploy.contains(app.getName()))
.map(app -> updateDeployedAppNameIfNeeded(app, appsToUpdate))
.collect(Collectors.toList());
context.setVariable(Variables.DEPLOYED_MTA, ImmutableDeployedMta.copyOf(deployedMta)
.withApplications(updatedApps));
}
private DeployedMtaApplication updateDeployedAppNameIfNeeded(DeployedMtaApplication app, List appsToUpdate) {
if (appsToUpdate.contains(app.getName())) {
return ImmutableDeployedMtaApplication.copyOf(app)
.withName(app.getName() + BlueGreenApplicationNameSuffix.LIVE.asSuffix());
}
return app;
}
@Override
protected String getStepErrorMessage(ProcessContext context) {
return Messages.ERROR_DETECTING_APPLICATIONS_TO_RENAME;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy