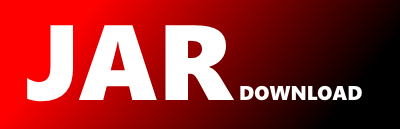
org.cloudfoundry.multiapps.controller.process.steps.ImmutableProcessStepHelper Maven / Gradle / Ivy
package org.cloudfoundry.multiapps.controller.process.steps;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
import org.cloudfoundry.multiapps.controller.persistence.services.ProcessLoggerPersister;
import org.cloudfoundry.multiapps.controller.persistence.services.ProgressMessageService;
import org.cloudfoundry.multiapps.controller.process.util.ProcessHelper;
import org.cloudfoundry.multiapps.controller.process.util.StepLogger;
import org.flowable.engine.ProcessEngineConfiguration;
/**
* Immutable implementation of {@link ProcessStepHelper}.
*
* Use the builder to create immutable instances:
* {@code ImmutableProcessStepHelper.builder()}.
*/
@SuppressWarnings({"all"})
public final class ImmutableProcessStepHelper
extends ProcessStepHelper {
private final ProgressMessageService progressMessageService;
private final StepLogger stepLogger;
private final ProcessLoggerPersister processLoggerPersister;
private final ProcessEngineConfiguration processEngineConfiguration;
private final ProcessHelper processHelper;
private ImmutableProcessStepHelper(
ProgressMessageService progressMessageService,
StepLogger stepLogger,
ProcessLoggerPersister processLoggerPersister,
ProcessEngineConfiguration processEngineConfiguration,
ProcessHelper processHelper) {
this.progressMessageService = progressMessageService;
this.stepLogger = stepLogger;
this.processLoggerPersister = processLoggerPersister;
this.processEngineConfiguration = processEngineConfiguration;
this.processHelper = processHelper;
}
/**
* @return The value of the {@code progressMessageService} attribute
*/
@Override
public ProgressMessageService getProgressMessageService() {
return progressMessageService;
}
/**
* @return The value of the {@code stepLogger} attribute
*/
@Override
public StepLogger getStepLogger() {
return stepLogger;
}
/**
* @return The value of the {@code processLoggerPersister} attribute
*/
@Override
public ProcessLoggerPersister getProcessLoggerPersister() {
return processLoggerPersister;
}
/**
* @return The value of the {@code processEngineConfiguration} attribute
*/
@Override
public ProcessEngineConfiguration getProcessEngineConfiguration() {
return processEngineConfiguration;
}
/**
* @return The value of the {@code processHelper} attribute
*/
@Override
public ProcessHelper getProcessHelper() {
return processHelper;
}
/**
* Copy the current immutable object by setting a value for the {@link ProcessStepHelper#getProgressMessageService() progressMessageService} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for progressMessageService
* @return A modified copy of the {@code this} object
*/
public final ImmutableProcessStepHelper withProgressMessageService(ProgressMessageService value) {
if (this.progressMessageService == value) return this;
ProgressMessageService newValue = Objects.requireNonNull(value, "progressMessageService");
return new ImmutableProcessStepHelper(
newValue,
this.stepLogger,
this.processLoggerPersister,
this.processEngineConfiguration,
this.processHelper);
}
/**
* Copy the current immutable object by setting a value for the {@link ProcessStepHelper#getStepLogger() stepLogger} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for stepLogger
* @return A modified copy of the {@code this} object
*/
public final ImmutableProcessStepHelper withStepLogger(StepLogger value) {
if (this.stepLogger == value) return this;
StepLogger newValue = Objects.requireNonNull(value, "stepLogger");
return new ImmutableProcessStepHelper(
this.progressMessageService,
newValue,
this.processLoggerPersister,
this.processEngineConfiguration,
this.processHelper);
}
/**
* Copy the current immutable object by setting a value for the {@link ProcessStepHelper#getProcessLoggerPersister() processLoggerPersister} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for processLoggerPersister
* @return A modified copy of the {@code this} object
*/
public final ImmutableProcessStepHelper withProcessLoggerPersister(ProcessLoggerPersister value) {
if (this.processLoggerPersister == value) return this;
ProcessLoggerPersister newValue = Objects.requireNonNull(value, "processLoggerPersister");
return new ImmutableProcessStepHelper(
this.progressMessageService,
this.stepLogger,
newValue,
this.processEngineConfiguration,
this.processHelper);
}
/**
* Copy the current immutable object by setting a value for the {@link ProcessStepHelper#getProcessEngineConfiguration() processEngineConfiguration} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for processEngineConfiguration
* @return A modified copy of the {@code this} object
*/
public final ImmutableProcessStepHelper withProcessEngineConfiguration(ProcessEngineConfiguration value) {
if (this.processEngineConfiguration == value) return this;
ProcessEngineConfiguration newValue = Objects.requireNonNull(value, "processEngineConfiguration");
return new ImmutableProcessStepHelper(
this.progressMessageService,
this.stepLogger,
this.processLoggerPersister,
newValue,
this.processHelper);
}
/**
* Copy the current immutable object by setting a value for the {@link ProcessStepHelper#getProcessHelper() processHelper} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for processHelper
* @return A modified copy of the {@code this} object
*/
public final ImmutableProcessStepHelper withProcessHelper(ProcessHelper value) {
if (this.processHelper == value) return this;
ProcessHelper newValue = Objects.requireNonNull(value, "processHelper");
return new ImmutableProcessStepHelper(
this.progressMessageService,
this.stepLogger,
this.processLoggerPersister,
this.processEngineConfiguration,
newValue);
}
/**
* This instance is equal to all instances of {@code ImmutableProcessStepHelper} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(Object another) {
if (this == another) return true;
return another instanceof ImmutableProcessStepHelper
&& equalTo(0, (ImmutableProcessStepHelper) another);
}
private boolean equalTo(int synthetic, ImmutableProcessStepHelper another) {
return progressMessageService.equals(another.progressMessageService)
&& stepLogger.equals(another.stepLogger)
&& processLoggerPersister.equals(another.processLoggerPersister)
&& processEngineConfiguration.equals(another.processEngineConfiguration)
&& processHelper.equals(another.processHelper);
}
/**
* Computes a hash code from attributes: {@code progressMessageService}, {@code stepLogger}, {@code processLoggerPersister}, {@code processEngineConfiguration}, {@code processHelper}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 5381;
h += (h << 5) + progressMessageService.hashCode();
h += (h << 5) + stepLogger.hashCode();
h += (h << 5) + processLoggerPersister.hashCode();
h += (h << 5) + processEngineConfiguration.hashCode();
h += (h << 5) + processHelper.hashCode();
return h;
}
/**
* Prints the immutable value {@code ProcessStepHelper} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
return "ProcessStepHelper{"
+ "progressMessageService=" + progressMessageService
+ ", stepLogger=" + stepLogger
+ ", processLoggerPersister=" + processLoggerPersister
+ ", processEngineConfiguration=" + processEngineConfiguration
+ ", processHelper=" + processHelper
+ "}";
}
/**
* Creates an immutable copy of a {@link ProcessStepHelper} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable ProcessStepHelper instance
*/
public static ImmutableProcessStepHelper copyOf(ProcessStepHelper instance) {
if (instance instanceof ImmutableProcessStepHelper) {
return (ImmutableProcessStepHelper) instance;
}
return ImmutableProcessStepHelper.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link ImmutableProcessStepHelper ImmutableProcessStepHelper}.
*
* ImmutableProcessStepHelper.builder()
* .progressMessageService(org.cloudfoundry.multiapps.controller.persistence.services.ProgressMessageService) // required {@link ProcessStepHelper#getProgressMessageService() progressMessageService}
* .stepLogger(org.cloudfoundry.multiapps.controller.process.util.StepLogger) // required {@link ProcessStepHelper#getStepLogger() stepLogger}
* .processLoggerPersister(org.cloudfoundry.multiapps.controller.persistence.services.ProcessLoggerPersister) // required {@link ProcessStepHelper#getProcessLoggerPersister() processLoggerPersister}
* .processEngineConfiguration(org.flowable.engine.ProcessEngineConfiguration) // required {@link ProcessStepHelper#getProcessEngineConfiguration() processEngineConfiguration}
* .processHelper(org.cloudfoundry.multiapps.controller.process.util.ProcessHelper) // required {@link ProcessStepHelper#getProcessHelper() processHelper}
* .build();
*
* @return A new ImmutableProcessStepHelper builder
*/
public static ImmutableProcessStepHelper.Builder builder() {
return new ImmutableProcessStepHelper.Builder();
}
/**
* Builds instances of type {@link ImmutableProcessStepHelper ImmutableProcessStepHelper}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
public static final class Builder {
private static final long INIT_BIT_PROGRESS_MESSAGE_SERVICE = 0x1L;
private static final long INIT_BIT_STEP_LOGGER = 0x2L;
private static final long INIT_BIT_PROCESS_LOGGER_PERSISTER = 0x4L;
private static final long INIT_BIT_PROCESS_ENGINE_CONFIGURATION = 0x8L;
private static final long INIT_BIT_PROCESS_HELPER = 0x10L;
private long initBits = 0x1fL;
private ProgressMessageService progressMessageService;
private StepLogger stepLogger;
private ProcessLoggerPersister processLoggerPersister;
private ProcessEngineConfiguration processEngineConfiguration;
private ProcessHelper processHelper;
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code ProcessStepHelper} instance.
* Regular attribute values will be replaced with those from the given instance.
* Absent optional values will not replace present values.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(ProcessStepHelper instance) {
Objects.requireNonNull(instance, "instance");
this.progressMessageService(instance.getProgressMessageService());
this.stepLogger(instance.getStepLogger());
this.processLoggerPersister(instance.getProcessLoggerPersister());
this.processEngineConfiguration(instance.getProcessEngineConfiguration());
this.processHelper(instance.getProcessHelper());
return this;
}
/**
* Initializes the value for the {@link ProcessStepHelper#getProgressMessageService() progressMessageService} attribute.
* @param progressMessageService The value for progressMessageService
* @return {@code this} builder for use in a chained invocation
*/
public final Builder progressMessageService(ProgressMessageService progressMessageService) {
this.progressMessageService = Objects.requireNonNull(progressMessageService, "progressMessageService");
initBits &= ~INIT_BIT_PROGRESS_MESSAGE_SERVICE;
return this;
}
/**
* Initializes the value for the {@link ProcessStepHelper#getStepLogger() stepLogger} attribute.
* @param stepLogger The value for stepLogger
* @return {@code this} builder for use in a chained invocation
*/
public final Builder stepLogger(StepLogger stepLogger) {
this.stepLogger = Objects.requireNonNull(stepLogger, "stepLogger");
initBits &= ~INIT_BIT_STEP_LOGGER;
return this;
}
/**
* Initializes the value for the {@link ProcessStepHelper#getProcessLoggerPersister() processLoggerPersister} attribute.
* @param processLoggerPersister The value for processLoggerPersister
* @return {@code this} builder for use in a chained invocation
*/
public final Builder processLoggerPersister(ProcessLoggerPersister processLoggerPersister) {
this.processLoggerPersister = Objects.requireNonNull(processLoggerPersister, "processLoggerPersister");
initBits &= ~INIT_BIT_PROCESS_LOGGER_PERSISTER;
return this;
}
/**
* Initializes the value for the {@link ProcessStepHelper#getProcessEngineConfiguration() processEngineConfiguration} attribute.
* @param processEngineConfiguration The value for processEngineConfiguration
* @return {@code this} builder for use in a chained invocation
*/
public final Builder processEngineConfiguration(ProcessEngineConfiguration processEngineConfiguration) {
this.processEngineConfiguration = Objects.requireNonNull(processEngineConfiguration, "processEngineConfiguration");
initBits &= ~INIT_BIT_PROCESS_ENGINE_CONFIGURATION;
return this;
}
/**
* Initializes the value for the {@link ProcessStepHelper#getProcessHelper() processHelper} attribute.
* @param processHelper The value for processHelper
* @return {@code this} builder for use in a chained invocation
*/
public final Builder processHelper(ProcessHelper processHelper) {
this.processHelper = Objects.requireNonNull(processHelper, "processHelper");
initBits &= ~INIT_BIT_PROCESS_HELPER;
return this;
}
/**
* Builds a new {@link ImmutableProcessStepHelper ImmutableProcessStepHelper}.
* @return An immutable instance of ProcessStepHelper
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public ImmutableProcessStepHelper build() {
if (initBits != 0) {
throw new IllegalStateException(formatRequiredAttributesMessage());
}
return new ImmutableProcessStepHelper(
progressMessageService,
stepLogger,
processLoggerPersister,
processEngineConfiguration,
processHelper);
}
private String formatRequiredAttributesMessage() {
List attributes = new ArrayList<>();
if ((initBits & INIT_BIT_PROGRESS_MESSAGE_SERVICE) != 0) attributes.add("progressMessageService");
if ((initBits & INIT_BIT_STEP_LOGGER) != 0) attributes.add("stepLogger");
if ((initBits & INIT_BIT_PROCESS_LOGGER_PERSISTER) != 0) attributes.add("processLoggerPersister");
if ((initBits & INIT_BIT_PROCESS_ENGINE_CONFIGURATION) != 0) attributes.add("processEngineConfiguration");
if ((initBits & INIT_BIT_PROCESS_HELPER) != 0) attributes.add("processHelper");
return "Cannot build ProcessStepHelper, some of required attributes are not set " + attributes;
}
}
}