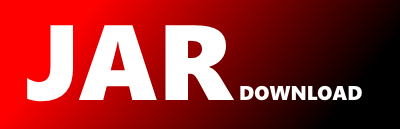
org.cloudfoundry.multiapps.controller.process.steps.PollServiceBindingOrKeyOperationExecution Maven / Gradle / Ivy
package org.cloudfoundry.multiapps.controller.process.steps;
import java.util.List;
import org.cloudfoundry.multiapps.controller.process.Messages;
import org.cloudfoundry.multiapps.controller.process.variables.Variables;
import com.sap.cloudfoundry.client.facade.CloudControllerClient;
import com.sap.cloudfoundry.client.facade.domain.CloudServiceBinding;
import com.sap.cloudfoundry.client.facade.domain.CloudServiceInstance;
import com.sap.cloudfoundry.client.facade.domain.CloudServiceKey;
import com.sap.cloudfoundry.client.facade.domain.ServiceCredentialBindingOperation;
public class PollServiceBindingOrKeyOperationExecution implements AsyncExecution {
@Override
public AsyncExecutionState execute(ProcessContext context) {
CloudControllerClient controllerClient = context.getControllerClient();
String serviceName = context.getVariable(Variables.SERVICE_WITH_BIND_IN_PROGRESS);
CloudServiceInstance service = controllerClient.getServiceInstance(serviceName);
List serviceBindings = controllerClient.getServiceAppBindings(service.getGuid());
List serviceKeys = controllerClient.getServiceKeys(serviceName);
if (doesServiceHaveBindingWithStateInProgress(serviceBindings) || doesServiceHaveKeysWithStateInProgress(serviceKeys)) {
return AsyncExecutionState.RUNNING;
}
context.setVariable(Variables.WAS_SERVICE_BINDING_KEY_OPERATION_ALREADY_DONE, true);
context.setVariable(Variables.IS_SERVICE_BINDING_KEY_OPERATION_IN_PROGRESS, false);
return AsyncExecutionState.FINISHED;
}
private boolean doesServiceHaveBindingWithStateInProgress(List serviceBindings) {
return serviceBindings.stream()
.anyMatch(serviceBinding -> serviceBinding.getServiceBindingOperation()
.getState()
.equals(ServiceCredentialBindingOperation.State.IN_PROGRESS));
}
private boolean doesServiceHaveKeysWithStateInProgress(List serviceKeys) {
return serviceKeys.stream()
.anyMatch(serviceKey -> serviceKey.getServiceKeyOperation()
.getState()
.equals(ServiceCredentialBindingOperation.State.IN_PROGRESS));
}
@Override
public String getPollingErrorMessage(ProcessContext context) {
return Messages.ERROR_MONITORING_OPERATION_OF_BINDING_OR_KEY_OF_SERVICE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy