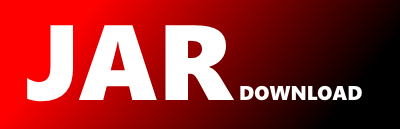
org.cloudfoundry.multiapps.controller.process.util.ApplicationArchiveContext Maven / Gradle / Ivy
package org.cloudfoundry.multiapps.controller.process.util;
import java.security.MessageDigest;
import java.security.NoSuchAlgorithmException;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
import org.cloudfoundry.multiapps.controller.persistence.Constants;
public class ApplicationArchiveContext {
private final String moduleFileName;
private final long maxSizeInBytes;
private final List archiveEntryWithStreamPositions;
private final String spaceId;
private final String appArchiveId;
private long currentSizeInBytes;
private DigestCalculator applicationDigestCalculator;
private Set alreadyUploadedFiles;
public ApplicationArchiveContext(String moduleFileName, long maxSizeInBytes,
List archiveEntryWithStreamPositions, String spaceId,
String appArchiveId) {
this.moduleFileName = moduleFileName;
this.maxSizeInBytes = maxSizeInBytes;
this.archiveEntryWithStreamPositions = archiveEntryWithStreamPositions;
this.spaceId = spaceId;
this.appArchiveId = appArchiveId;
createDigestCalculator(Constants.DIGEST_ALGORITHM);
}
private void createDigestCalculator(String algorithm) {
try {
this.applicationDigestCalculator = new DigestCalculator(MessageDigest.getInstance(algorithm));
} catch (NoSuchAlgorithmException e) {
throw new IllegalStateException(e);
}
}
public long getCurrentSizeInBytes() {
return currentSizeInBytes;
}
public void calculateCurrentSizeInBytes(long sizeInBytes) {
currentSizeInBytes += sizeInBytes;
}
public String getModuleFileName() {
return moduleFileName;
}
public long getMaxSizeInBytes() {
return maxSizeInBytes;
}
public DigestCalculator getDigestCalculator() {
return applicationDigestCalculator;
}
public Set getAlreadyUploadedFiles() {
if (alreadyUploadedFiles == null) {
return new HashSet<>();
}
return alreadyUploadedFiles;
}
public void setAlreadyUploadedFiles(Set alreadyUploadedFiles) {
this.alreadyUploadedFiles = alreadyUploadedFiles;
}
public List getArchiveEntryWithStreamPositions() {
return archiveEntryWithStreamPositions;
}
public String getSpaceId() {
return spaceId;
}
public String getAppArchiveId() {
return appArchiveId;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy