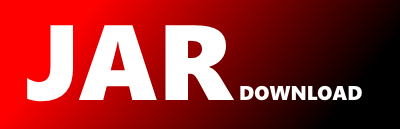
org.cloudfoundry.multiapps.controller.process.util.ImmutableAsyncJobToAsyncExecutionStateAdapter Maven / Gradle / Ivy
package org.cloudfoundry.multiapps.controller.process.util;
import com.sap.cloudfoundry.client.facade.domain.CloudAsyncJob;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
import java.util.function.Consumer;
/**
* Immutable implementation of {@link AsyncJobToAsyncExecutionStateAdapter}.
*
* Use the builder to create immutable instances:
* {@code ImmutableAsyncJobToAsyncExecutionStateAdapter.builder()}.
*/
@SuppressWarnings({"all"})
public final class ImmutableAsyncJobToAsyncExecutionStateAdapter
implements AsyncJobToAsyncExecutionStateAdapter {
private final Consumer onErrorHandler;
private final Consumer onErrorHandlerForOptionalResource;
private final Consumer onCompleteHandler;
private final Consumer inProgressHandler;
private final boolean isOptionalResource;
private ImmutableAsyncJobToAsyncExecutionStateAdapter(ImmutableAsyncJobToAsyncExecutionStateAdapter.Builder builder) {
this.onErrorHandler = builder.onErrorHandler;
this.onErrorHandlerForOptionalResource = builder.onErrorHandlerForOptionalResource;
this.onCompleteHandler = builder.onCompleteHandler;
this.inProgressHandler = builder.inProgressHandler;
this.isOptionalResource = builder.isOptionalResourceIsSet()
? builder.isOptionalResource
: AsyncJobToAsyncExecutionStateAdapter.super.isOptionalResource();
}
private ImmutableAsyncJobToAsyncExecutionStateAdapter(
Consumer onErrorHandler,
Consumer onErrorHandlerForOptionalResource,
Consumer onCompleteHandler,
Consumer inProgressHandler,
boolean isOptionalResource) {
this.onErrorHandler = onErrorHandler;
this.onErrorHandlerForOptionalResource = onErrorHandlerForOptionalResource;
this.onCompleteHandler = onCompleteHandler;
this.inProgressHandler = inProgressHandler;
this.isOptionalResource = isOptionalResource;
}
/**
* @return The value of the {@code onErrorHandler} attribute
*/
@Override
public Consumer getOnErrorHandler() {
return onErrorHandler;
}
/**
* @return The value of the {@code onErrorHandlerForOptionalResource} attribute
*/
@Override
public Consumer getOnErrorHandlerForOptionalResource() {
return onErrorHandlerForOptionalResource;
}
/**
* @return The value of the {@code onCompleteHandler} attribute
*/
@Override
public Consumer getOnCompleteHandler() {
return onCompleteHandler;
}
/**
* @return The value of the {@code inProgressHandler} attribute
*/
@Override
public Consumer getInProgressHandler() {
return inProgressHandler;
}
/**
* @return The value of the {@code isOptionalResource} attribute
*/
@Override
public boolean isOptionalResource() {
return isOptionalResource;
}
/**
* Copy the current immutable object by setting a value for the {@link AsyncJobToAsyncExecutionStateAdapter#getOnErrorHandler() onErrorHandler} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for onErrorHandler
* @return A modified copy of the {@code this} object
*/
public final ImmutableAsyncJobToAsyncExecutionStateAdapter withOnErrorHandler(Consumer value) {
if (this.onErrorHandler == value) return this;
Consumer newValue = Objects.requireNonNull(value, "onErrorHandler");
return new ImmutableAsyncJobToAsyncExecutionStateAdapter(
newValue,
this.onErrorHandlerForOptionalResource,
this.onCompleteHandler,
this.inProgressHandler,
this.isOptionalResource);
}
/**
* Copy the current immutable object by setting a value for the {@link AsyncJobToAsyncExecutionStateAdapter#getOnErrorHandlerForOptionalResource() onErrorHandlerForOptionalResource} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for onErrorHandlerForOptionalResource
* @return A modified copy of the {@code this} object
*/
public final ImmutableAsyncJobToAsyncExecutionStateAdapter withOnErrorHandlerForOptionalResource(Consumer value) {
if (this.onErrorHandlerForOptionalResource == value) return this;
Consumer newValue = Objects.requireNonNull(value, "onErrorHandlerForOptionalResource");
return new ImmutableAsyncJobToAsyncExecutionStateAdapter(
this.onErrorHandler,
newValue,
this.onCompleteHandler,
this.inProgressHandler,
this.isOptionalResource);
}
/**
* Copy the current immutable object by setting a value for the {@link AsyncJobToAsyncExecutionStateAdapter#getOnCompleteHandler() onCompleteHandler} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for onCompleteHandler
* @return A modified copy of the {@code this} object
*/
public final ImmutableAsyncJobToAsyncExecutionStateAdapter withOnCompleteHandler(Consumer value) {
if (this.onCompleteHandler == value) return this;
Consumer newValue = Objects.requireNonNull(value, "onCompleteHandler");
return new ImmutableAsyncJobToAsyncExecutionStateAdapter(
this.onErrorHandler,
this.onErrorHandlerForOptionalResource,
newValue,
this.inProgressHandler,
this.isOptionalResource);
}
/**
* Copy the current immutable object by setting a value for the {@link AsyncJobToAsyncExecutionStateAdapter#getInProgressHandler() inProgressHandler} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for inProgressHandler
* @return A modified copy of the {@code this} object
*/
public final ImmutableAsyncJobToAsyncExecutionStateAdapter withInProgressHandler(Consumer value) {
if (this.inProgressHandler == value) return this;
Consumer newValue = Objects.requireNonNull(value, "inProgressHandler");
return new ImmutableAsyncJobToAsyncExecutionStateAdapter(
this.onErrorHandler,
this.onErrorHandlerForOptionalResource,
this.onCompleteHandler,
newValue,
this.isOptionalResource);
}
/**
* Copy the current immutable object by setting a value for the {@link AsyncJobToAsyncExecutionStateAdapter#isOptionalResource() isOptionalResource} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for isOptionalResource
* @return A modified copy of the {@code this} object
*/
public final ImmutableAsyncJobToAsyncExecutionStateAdapter withIsOptionalResource(boolean value) {
if (this.isOptionalResource == value) return this;
return new ImmutableAsyncJobToAsyncExecutionStateAdapter(
this.onErrorHandler,
this.onErrorHandlerForOptionalResource,
this.onCompleteHandler,
this.inProgressHandler,
value);
}
/**
* This instance is equal to all instances of {@code ImmutableAsyncJobToAsyncExecutionStateAdapter} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(Object another) {
if (this == another) return true;
return another instanceof ImmutableAsyncJobToAsyncExecutionStateAdapter
&& equalTo(0, (ImmutableAsyncJobToAsyncExecutionStateAdapter) another);
}
private boolean equalTo(int synthetic, ImmutableAsyncJobToAsyncExecutionStateAdapter another) {
return onErrorHandler.equals(another.onErrorHandler)
&& onErrorHandlerForOptionalResource.equals(another.onErrorHandlerForOptionalResource)
&& onCompleteHandler.equals(another.onCompleteHandler)
&& inProgressHandler.equals(another.inProgressHandler)
&& isOptionalResource == another.isOptionalResource;
}
/**
* Computes a hash code from attributes: {@code onErrorHandler}, {@code onErrorHandlerForOptionalResource}, {@code onCompleteHandler}, {@code inProgressHandler}, {@code isOptionalResource}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 5381;
h += (h << 5) + onErrorHandler.hashCode();
h += (h << 5) + onErrorHandlerForOptionalResource.hashCode();
h += (h << 5) + onCompleteHandler.hashCode();
h += (h << 5) + inProgressHandler.hashCode();
h += (h << 5) + Boolean.hashCode(isOptionalResource);
return h;
}
/**
* Prints the immutable value {@code AsyncJobToAsyncExecutionStateAdapter} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
return "AsyncJobToAsyncExecutionStateAdapter{"
+ "onErrorHandler=" + onErrorHandler
+ ", onErrorHandlerForOptionalResource=" + onErrorHandlerForOptionalResource
+ ", onCompleteHandler=" + onCompleteHandler
+ ", inProgressHandler=" + inProgressHandler
+ ", isOptionalResource=" + isOptionalResource
+ "}";
}
/**
* Creates an immutable copy of a {@link AsyncJobToAsyncExecutionStateAdapter} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable AsyncJobToAsyncExecutionStateAdapter instance
*/
public static ImmutableAsyncJobToAsyncExecutionStateAdapter copyOf(AsyncJobToAsyncExecutionStateAdapter instance) {
if (instance instanceof ImmutableAsyncJobToAsyncExecutionStateAdapter) {
return (ImmutableAsyncJobToAsyncExecutionStateAdapter) instance;
}
return ImmutableAsyncJobToAsyncExecutionStateAdapter.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link ImmutableAsyncJobToAsyncExecutionStateAdapter ImmutableAsyncJobToAsyncExecutionStateAdapter}.
*
* ImmutableAsyncJobToAsyncExecutionStateAdapter.builder()
* .onErrorHandler(function.Consumer<com.sap.cloudfoundry.client.facade.domain.CloudAsyncJob>) // required {@link AsyncJobToAsyncExecutionStateAdapter#getOnErrorHandler() onErrorHandler}
* .onErrorHandlerForOptionalResource(function.Consumer<com.sap.cloudfoundry.client.facade.domain.CloudAsyncJob>) // required {@link AsyncJobToAsyncExecutionStateAdapter#getOnErrorHandlerForOptionalResource() onErrorHandlerForOptionalResource}
* .onCompleteHandler(function.Consumer<com.sap.cloudfoundry.client.facade.domain.CloudAsyncJob>) // required {@link AsyncJobToAsyncExecutionStateAdapter#getOnCompleteHandler() onCompleteHandler}
* .inProgressHandler(function.Consumer<com.sap.cloudfoundry.client.facade.domain.CloudAsyncJob>) // required {@link AsyncJobToAsyncExecutionStateAdapter#getInProgressHandler() inProgressHandler}
* .isOptionalResource(boolean) // optional {@link AsyncJobToAsyncExecutionStateAdapter#isOptionalResource() isOptionalResource}
* .build();
*
* @return A new ImmutableAsyncJobToAsyncExecutionStateAdapter builder
*/
public static ImmutableAsyncJobToAsyncExecutionStateAdapter.Builder builder() {
return new ImmutableAsyncJobToAsyncExecutionStateAdapter.Builder();
}
/**
* Builds instances of type {@link ImmutableAsyncJobToAsyncExecutionStateAdapter ImmutableAsyncJobToAsyncExecutionStateAdapter}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
public static final class Builder {
private static final long INIT_BIT_ON_ERROR_HANDLER = 0x1L;
private static final long INIT_BIT_ON_ERROR_HANDLER_FOR_OPTIONAL_RESOURCE = 0x2L;
private static final long INIT_BIT_ON_COMPLETE_HANDLER = 0x4L;
private static final long INIT_BIT_IN_PROGRESS_HANDLER = 0x8L;
private static final long OPT_BIT_IS_OPTIONAL_RESOURCE = 0x1L;
private long initBits = 0xfL;
private long optBits;
private Consumer onErrorHandler;
private Consumer onErrorHandlerForOptionalResource;
private Consumer onCompleteHandler;
private Consumer inProgressHandler;
private boolean isOptionalResource;
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code AsyncJobToAsyncExecutionStateAdapter} instance.
* Regular attribute values will be replaced with those from the given instance.
* Absent optional values will not replace present values.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(AsyncJobToAsyncExecutionStateAdapter instance) {
Objects.requireNonNull(instance, "instance");
this.onErrorHandler(instance.getOnErrorHandler());
this.onErrorHandlerForOptionalResource(instance.getOnErrorHandlerForOptionalResource());
this.onCompleteHandler(instance.getOnCompleteHandler());
this.inProgressHandler(instance.getInProgressHandler());
this.isOptionalResource(instance.isOptionalResource());
return this;
}
/**
* Initializes the value for the {@link AsyncJobToAsyncExecutionStateAdapter#getOnErrorHandler() onErrorHandler} attribute.
* @param onErrorHandler The value for onErrorHandler
* @return {@code this} builder for use in a chained invocation
*/
public final Builder onErrorHandler(Consumer onErrorHandler) {
this.onErrorHandler = Objects.requireNonNull(onErrorHandler, "onErrorHandler");
initBits &= ~INIT_BIT_ON_ERROR_HANDLER;
return this;
}
/**
* Initializes the value for the {@link AsyncJobToAsyncExecutionStateAdapter#getOnErrorHandlerForOptionalResource() onErrorHandlerForOptionalResource} attribute.
* @param onErrorHandlerForOptionalResource The value for onErrorHandlerForOptionalResource
* @return {@code this} builder for use in a chained invocation
*/
public final Builder onErrorHandlerForOptionalResource(Consumer onErrorHandlerForOptionalResource) {
this.onErrorHandlerForOptionalResource = Objects.requireNonNull(onErrorHandlerForOptionalResource, "onErrorHandlerForOptionalResource");
initBits &= ~INIT_BIT_ON_ERROR_HANDLER_FOR_OPTIONAL_RESOURCE;
return this;
}
/**
* Initializes the value for the {@link AsyncJobToAsyncExecutionStateAdapter#getOnCompleteHandler() onCompleteHandler} attribute.
* @param onCompleteHandler The value for onCompleteHandler
* @return {@code this} builder for use in a chained invocation
*/
public final Builder onCompleteHandler(Consumer onCompleteHandler) {
this.onCompleteHandler = Objects.requireNonNull(onCompleteHandler, "onCompleteHandler");
initBits &= ~INIT_BIT_ON_COMPLETE_HANDLER;
return this;
}
/**
* Initializes the value for the {@link AsyncJobToAsyncExecutionStateAdapter#getInProgressHandler() inProgressHandler} attribute.
* @param inProgressHandler The value for inProgressHandler
* @return {@code this} builder for use in a chained invocation
*/
public final Builder inProgressHandler(Consumer inProgressHandler) {
this.inProgressHandler = Objects.requireNonNull(inProgressHandler, "inProgressHandler");
initBits &= ~INIT_BIT_IN_PROGRESS_HANDLER;
return this;
}
/**
* Initializes the value for the {@link AsyncJobToAsyncExecutionStateAdapter#isOptionalResource() isOptionalResource} attribute.
* If not set, this attribute will have a default value as returned by the initializer of {@link AsyncJobToAsyncExecutionStateAdapter#isOptionalResource() isOptionalResource}.
* @param isOptionalResource The value for isOptionalResource
* @return {@code this} builder for use in a chained invocation
*/
public final Builder isOptionalResource(boolean isOptionalResource) {
this.isOptionalResource = isOptionalResource;
optBits |= OPT_BIT_IS_OPTIONAL_RESOURCE;
return this;
}
/**
* Builds a new {@link ImmutableAsyncJobToAsyncExecutionStateAdapter ImmutableAsyncJobToAsyncExecutionStateAdapter}.
* @return An immutable instance of AsyncJobToAsyncExecutionStateAdapter
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public ImmutableAsyncJobToAsyncExecutionStateAdapter build() {
if (initBits != 0) {
throw new IllegalStateException(formatRequiredAttributesMessage());
}
return new ImmutableAsyncJobToAsyncExecutionStateAdapter(this);
}
private boolean isOptionalResourceIsSet() {
return (optBits & OPT_BIT_IS_OPTIONAL_RESOURCE) != 0;
}
private String formatRequiredAttributesMessage() {
List attributes = new ArrayList<>();
if ((initBits & INIT_BIT_ON_ERROR_HANDLER) != 0) attributes.add("onErrorHandler");
if ((initBits & INIT_BIT_ON_ERROR_HANDLER_FOR_OPTIONAL_RESOURCE) != 0) attributes.add("onErrorHandlerForOptionalResource");
if ((initBits & INIT_BIT_ON_COMPLETE_HANDLER) != 0) attributes.add("onCompleteHandler");
if ((initBits & INIT_BIT_IN_PROGRESS_HANDLER) != 0) attributes.add("inProgressHandler");
return "Cannot build AsyncJobToAsyncExecutionStateAdapter, some of required attributes are not set " + attributes;
}
}
}