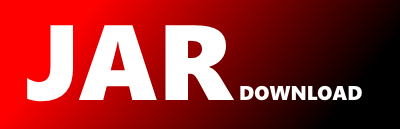
org.cloudfoundry.multiapps.controller.process.variables.ImmutableJsonStringVariable Maven / Gradle / Ivy
package org.cloudfoundry.multiapps.controller.process.variables;
import com.fasterxml.jackson.core.type.TypeReference;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
import org.cloudfoundry.multiapps.common.Nullable;
/**
* Immutable implementation of {@link JsonStringVariable}.
*
* Use the builder to create immutable instances:
* {@code ImmutableJsonStringVariable.builder()}.
*/
@SuppressWarnings({"all"})
public final class ImmutableJsonStringVariable
extends JsonStringVariable {
private final String name;
private final @Nullable T defaultValue;
private final TypeReference type;
private ImmutableJsonStringVariable(
String name,
@Nullable T defaultValue,
TypeReference type) {
this.name = name;
this.defaultValue = defaultValue;
this.type = type;
}
/**
* @return The value of the {@code name} attribute
*/
@Override
public String getName() {
return name;
}
/**
* @return The value of the {@code defaultValue} attribute
*/
@Override
public @Nullable T getDefaultValue() {
return defaultValue;
}
/**
* @return The value of the {@code type} attribute
*/
@Override
public TypeReference getType() {
return type;
}
/**
* Copy the current immutable object by setting a value for the {@link JsonStringVariable#getName() name} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for name
* @return A modified copy of the {@code this} object
*/
public final ImmutableJsonStringVariable withName(String value) {
String newValue = Objects.requireNonNull(value, "name");
if (this.name.equals(newValue)) return this;
return new ImmutableJsonStringVariable<>(newValue, this.defaultValue, this.type);
}
/**
* Copy the current immutable object by setting a value for the {@link JsonStringVariable#getDefaultValue() defaultValue} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for defaultValue (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableJsonStringVariable withDefaultValue(@Nullable T value) {
if (this.defaultValue == value) return this;
return new ImmutableJsonStringVariable<>(this.name, value, this.type);
}
/**
* Copy the current immutable object by setting a value for the {@link JsonStringVariable#getType() type} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for type
* @return A modified copy of the {@code this} object
*/
public final ImmutableJsonStringVariable withType(TypeReference value) {
if (this.type == value) return this;
TypeReference newValue = Objects.requireNonNull(value, "type");
return new ImmutableJsonStringVariable<>(this.name, this.defaultValue, newValue);
}
/**
* This instance is equal to all instances of {@code ImmutableJsonStringVariable} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(Object another) {
if (this == another) return true;
return another instanceof ImmutableJsonStringVariable>
&& equalTo(0, (ImmutableJsonStringVariable>) another);
}
private boolean equalTo(int synthetic, ImmutableJsonStringVariable> another) {
return name.equals(another.name)
&& Objects.equals(defaultValue, another.defaultValue)
&& type.equals(another.type);
}
/**
* Computes a hash code from attributes: {@code name}, {@code defaultValue}, {@code type}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 5381;
h += (h << 5) + name.hashCode();
h += (h << 5) + Objects.hashCode(defaultValue);
h += (h << 5) + type.hashCode();
return h;
}
/**
* Prints the immutable value {@code JsonStringVariable} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
return "JsonStringVariable{"
+ "name=" + name
+ ", defaultValue=" + defaultValue
+ ", type=" + type
+ "}";
}
/**
* Creates an immutable copy of a {@link JsonStringVariable} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param generic parameter T
* @param instance The instance to copy
* @return A copied immutable JsonStringVariable instance
*/
public static ImmutableJsonStringVariable copyOf(JsonStringVariable instance) {
if (instance instanceof ImmutableJsonStringVariable>) {
return (ImmutableJsonStringVariable) instance;
}
return ImmutableJsonStringVariable.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link ImmutableJsonStringVariable ImmutableJsonStringVariable}.
*
* ImmutableJsonStringVariable.<T>builder()
* .name(String) // required {@link JsonStringVariable#getName() name}
* .defaultValue(T | null) // nullable {@link JsonStringVariable#getDefaultValue() defaultValue}
* .type(com.fasterxml.jackson.core.type.TypeReference<T>) // required {@link JsonStringVariable#getType() type}
* .build();
*
* @param generic parameter T
* @return A new ImmutableJsonStringVariable builder
*/
public static ImmutableJsonStringVariable.Builder builder() {
return new ImmutableJsonStringVariable.Builder<>();
}
/**
* Builds instances of type {@link ImmutableJsonStringVariable ImmutableJsonStringVariable}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
public static final class Builder {
private static final long INIT_BIT_NAME = 0x1L;
private static final long INIT_BIT_TYPE = 0x2L;
private long initBits = 0x3L;
private String name;
private T defaultValue;
private TypeReference type;
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code org.cloudfoundry.multiapps.controller.process.variables.JsonStringVariable} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(JsonStringVariable instance) {
Objects.requireNonNull(instance, "instance");
from((short) 0, (Object) instance);
return this;
}
/**
* Fill a builder with attribute values from the provided {@code org.cloudfoundry.multiapps.controller.process.variables.Variable} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(Variable instance) {
Objects.requireNonNull(instance, "instance");
from((short) 0, (Object) instance);
return this;
}
@SuppressWarnings("unchecked")
private void from(short _unused, Object object) {
if (object instanceof JsonStringVariable>) {
JsonStringVariable instance = (JsonStringVariable) object;
this.type(instance.getType());
}
if (object instanceof Variable>) {
Variable instance = (Variable) object;
this.name(instance.getName());
@Nullable T defaultValueValue = instance.getDefaultValue();
if (defaultValueValue != null) {
defaultValue(defaultValueValue);
}
}
}
/**
* Initializes the value for the {@link JsonStringVariable#getName() name} attribute.
* @param name The value for name
* @return {@code this} builder for use in a chained invocation
*/
public final Builder name(String name) {
this.name = Objects.requireNonNull(name, "name");
initBits &= ~INIT_BIT_NAME;
return this;
}
/**
* Initializes the value for the {@link JsonStringVariable#getDefaultValue() defaultValue} attribute.
* @param defaultValue The value for defaultValue (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
public final Builder defaultValue(@Nullable T defaultValue) {
this.defaultValue = defaultValue;
return this;
}
/**
* Initializes the value for the {@link JsonStringVariable#getType() type} attribute.
* @param type The value for type
* @return {@code this} builder for use in a chained invocation
*/
public final Builder type(TypeReference type) {
this.type = Objects.requireNonNull(type, "type");
initBits &= ~INIT_BIT_TYPE;
return this;
}
/**
* Builds a new {@link ImmutableJsonStringVariable ImmutableJsonStringVariable}.
* @return An immutable instance of JsonStringVariable
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public ImmutableJsonStringVariable build() {
if (initBits != 0) {
throw new IllegalStateException(formatRequiredAttributesMessage());
}
return new ImmutableJsonStringVariable<>(name, defaultValue, type);
}
private String formatRequiredAttributesMessage() {
List attributes = new ArrayList<>();
if ((initBits & INIT_BIT_NAME) != 0) attributes.add("name");
if ((initBits & INIT_BIT_TYPE) != 0) attributes.add("type");
return "Cannot build JsonStringVariable, some of required attributes are not set " + attributes;
}
}
}