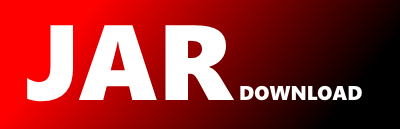
org.cloudfoundry.multiapps.controller.process.variables.VariableHandling Maven / Gradle / Ivy
package org.cloudfoundry.multiapps.controller.process.variables;
import org.flowable.common.engine.api.variable.VariableContainer;
import org.flowable.variable.api.delegate.VariableScope;
public final class VariableHandling {
private VariableHandling() {
}
public static void set(VariableContainer container, Variable variable, T value) {
if (value == null) {
container.setVariable(variable.getName(), null);
return;
}
Serializer serializer = variable.getSerializer();
container.setVariable(variable.getName(), serializer.serialize(value));
}
public static T get(VariableContainer container, Variable variable) {
Object serializedValue = container.getVariable(variable.getName());
if (serializedValue == null) {
return variable.getDefaultValue();
}
Serializer serializer = variable.getSerializer();
return serializer.deserialize(serializedValue);
}
public static T getIfSet(VariableContainer container, Variable variable) {
Object serializedValue = container.getVariable(variable.getName());
if (serializedValue == null) {
return null;
}
Serializer serializer = variable.getSerializer();
return serializer.deserialize(serializedValue);
}
public static T getBackwardsCompatible(VariableContainer container, Variable variable) {
Object serializedValue = container.getVariable(variable.getName());
if (serializedValue == null) {
return variable.getDefaultValue();
}
Serializer serializer = variable.getSerializer();
return serializer.deserialize(serializedValue, container);
}
public static void remove(VariableScope scope, Variable> variable) {
scope.removeVariable(variable.getName());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy