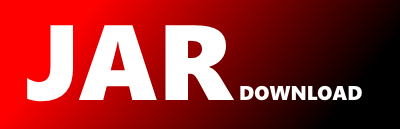
org.cloudfoundry.multiapps.controller.process.variables.Variables Maven / Gradle / Ivy
package org.cloudfoundry.multiapps.controller.process.variables;
import java.time.Duration;
import java.time.Instant;
import java.time.LocalDateTime;
import java.time.ZoneId;
import java.util.Collections;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.UUID;
import org.cloudfoundry.multiapps.controller.client.lib.domain.CloudApplicationExtended;
import org.cloudfoundry.multiapps.controller.client.lib.domain.CloudServiceInstanceExtended;
import org.cloudfoundry.multiapps.controller.core.cf.DeploymentMode;
import org.cloudfoundry.multiapps.controller.core.cf.apps.ApplicationStateAction;
import org.cloudfoundry.multiapps.controller.core.helpers.MtaArchiveElements;
import org.cloudfoundry.multiapps.controller.core.model.ApplicationColor;
import org.cloudfoundry.multiapps.controller.core.model.DeployedMta;
import org.cloudfoundry.multiapps.controller.core.model.DeployedMtaServiceKey;
import org.cloudfoundry.multiapps.controller.core.model.DynamicResolvableParameter;
import org.cloudfoundry.multiapps.controller.core.model.ErrorType;
import org.cloudfoundry.multiapps.controller.core.model.IncrementalAppInstanceUpdateConfiguration;
import org.cloudfoundry.multiapps.controller.core.model.Phase;
import org.cloudfoundry.multiapps.controller.core.model.SubprocessPhase;
import org.cloudfoundry.multiapps.controller.persistence.model.ConfigurationEntry;
import org.cloudfoundry.multiapps.controller.persistence.model.ConfigurationSubscription;
import org.cloudfoundry.multiapps.controller.persistence.model.FileEntry;
import org.cloudfoundry.multiapps.controller.process.DeployStrategy;
import org.cloudfoundry.multiapps.controller.process.steps.StepPhase;
import org.cloudfoundry.multiapps.controller.process.util.ArchiveEntryWithStreamPositions;
import org.cloudfoundry.multiapps.controller.process.util.ServiceAction;
import org.cloudfoundry.multiapps.controller.process.util.ServiceDeletionActions;
import org.cloudfoundry.multiapps.mta.model.DeploymentDescriptor;
import org.cloudfoundry.multiapps.mta.model.ExtensionDescriptor;
import org.cloudfoundry.multiapps.mta.model.Hook;
import org.cloudfoundry.multiapps.mta.model.Module;
import org.cloudfoundry.multiapps.mta.model.VersionRule;
import com.fasterxml.jackson.core.type.TypeReference;
import com.sap.cloudfoundry.client.facade.domain.CloudApplication;
import com.sap.cloudfoundry.client.facade.domain.CloudPackage;
import com.sap.cloudfoundry.client.facade.domain.CloudRoute;
import com.sap.cloudfoundry.client.facade.domain.CloudServiceBinding;
import com.sap.cloudfoundry.client.facade.domain.CloudServiceBroker;
import com.sap.cloudfoundry.client.facade.domain.CloudServiceKey;
import com.sap.cloudfoundry.client.facade.domain.CloudTask;
import com.sap.cloudfoundry.client.facade.domain.ServiceOperation;
public interface Variables {
Variable CORRELATION_ID = ImmutableSimpleVariable. builder()
.name("correlationId")
.build();
Variable ORGANIZATION_NAME = ImmutableSimpleVariable. builder()
.name("org")
.build();
Variable ORGANIZATION_GUID = ImmutableSimpleVariable. builder()
.name("orgId")
.build();
Variable SPACE_NAME = ImmutableSimpleVariable. builder()
.name("space")
.build();
Variable SPACE_GUID = ImmutableSimpleVariable. builder()
.name(org.cloudfoundry.multiapps.controller.persistence.Constants.VARIABLE_NAME_SPACE_ID)
.build();
Variable SUBPROCESS_ID = ImmutableSimpleVariable. builder()
.name("subProcessId")
.build();
Variable SERVICE_ID = ImmutableSimpleVariable. builder()
.name(org.cloudfoundry.multiapps.controller.persistence.Constants.VARIABLE_NAME_SERVICE_ID)
.build();
Variable TASK_ID = ImmutableSimpleVariable. builder()
.name("__TASK_ID")
.build();
Variable TIMESTAMP = ImmutableSimpleVariable. builder()
.name("timestamp")
.build();
Variable SERVICE_TO_PROCESS_NAME = ImmutableSimpleVariable. builder()
.name("serviceToProcessName")
.build();
Variable APP_ARCHIVE_ID = ImmutableSimpleVariable. builder()
.name("appArchiveId")
.build();
Variable EXT_DESCRIPTOR_FILE_ID = ImmutableSimpleVariable. builder()
.name("mtaExtDescriptorId")
.build();
Variable MTA_ID = ImmutableSimpleVariable. builder()
.name("mtaId")
.build();
Variable MTA_NAMESPACE = ImmutableSimpleVariable. builder()
.name("namespace")
.build();
Variable APPLY_NAMESPACE_APP_NAMES = ImmutableSimpleVariable. builder()
.name("applyNamespaceAppNames")
.defaultValue(null)
.build();
Variable APPLY_NAMESPACE_SERVICE_NAMES = ImmutableSimpleVariable. builder()
.name("applyNamespaceServiceNames")
.defaultValue(null)
.build();
Variable APPLY_NAMESPACE_APP_ROUTES = ImmutableSimpleVariable. builder()
.name("applyNamespaceAppRoutes")
.defaultValue(null)
.build();
Variable APPLY_NAMESPACE_AS_SUFFIX = ImmutableSimpleVariable. builder()
.name("applyNamespaceAsSuffix")
.defaultValue(null)
.build();
Variable CTS_PROCESS_ID = ImmutableSimpleVariable. builder()
.name("ctsProcessId")
.build();
Variable DEPLOY_URI = ImmutableSimpleVariable. builder()
.name("deployUri")
.build();
Variable CTS_USERNAME = ImmutableSimpleVariable. builder()
.name("userId")
.build();
Variable CTS_PASSWORD = ImmutableSimpleVariable. builder()
.name("password")
.build();
Variable TRANSFER_TYPE = ImmutableSimpleVariable. builder()
.name("transferType")
.build();
Variable APPLICATION_TYPE = ImmutableSimpleVariable. builder()
.name("applType")
.build();
Variable USER = ImmutableSimpleVariable. builder()
.name("user")
.build();
Variable SERVICE_OFFERING = ImmutableSimpleVariable. builder()
.name("serviceOffering")
.build();
Variable INDEX_VARIABLE_NAME = ImmutableSimpleVariable. builder()
.name("indexVariableName")
.build();
Variable STEP_EXECUTION = ImmutableSimpleVariable. builder()
.name("StepExecution")
.build();
Variable APP_CONTENT_CHANGED = ImmutableSimpleVariable. builder()
.name("appContentChanged")
.defaultValue(false)
.build();
Variable START_APPS = ImmutableSimpleVariable. builder()
.name("startApps")
.defaultValue(true)
.build();
Variable MTA_MAJOR_SCHEMA_VERSION = ImmutableSimpleVariable. builder()
.name("mtaMajorSchemaVersion")
.build();
Variable APPS_START_TIMEOUT_PROCESS_VARIABLE = ImmutableSimpleVariable. builder()
.name("startTimeout")
.defaultValue(Duration.ofHours(1))
.build();
Variable APPS_STAGE_TIMEOUT_PROCESS_VARIABLE = ImmutableSimpleVariable. builder()
.name("appsStageTimeout")
.defaultValue(Duration.ofHours(1))
.build();
Variable APPS_TASK_EXECUTION_TIMEOUT_PROCESS_VARIABLE = ImmutableSimpleVariable. builder()
.name("appsTaskExecutionTimeout")
.defaultValue(Duration.ofHours(12))
.build();
Variable APPS_UPLOAD_TIMEOUT_PROCESS_VARIABLE = ImmutableSimpleVariable. builder()
.name("appsUploadTimeout")
.defaultValue(Duration.ofHours(1))
.build();
Variable UPDATED_SERVICE_BROKER_SUBSCRIBERS_COUNT = ImmutableSimpleVariable. builder()
.name("updatedServiceBrokerSubscribersCount")
.build();
Variable UPDATED_SERVICE_BROKER_SUBSCRIBERS_INDEX = ImmutableSimpleVariable. builder()
.name("updatedServiceBrokerSubscribersIndex")
.build();
Variable MODULES_COUNT = ImmutableSimpleVariable. builder()
.name("modulesCount")
.build();
Variable MODULES_INDEX = ImmutableSimpleVariable. builder()
.name("modulesIndex")
.build();
Variable MTARS_COUNT = ImmutableSimpleVariable. builder()
.name("mtarsCount")
.build();
Variable MTARS_INDEX = ImmutableSimpleVariable. builder()
.name("mtarsIndex")
.build();
Variable TASKS_COUNT = ImmutableSimpleVariable. builder()
.name("tasksCount")
.build();
Variable TASKS_INDEX = ImmutableSimpleVariable. builder()
.name("tasksIndex")
.build();
Variable SERVICES_TO_CREATE_COUNT = ImmutableSimpleVariable. builder()
.name("servicesToCreateCount")
.build();
Variable ASYNC_STEP_EXECUTION_INDEX = ImmutableSimpleVariable. builder()
.name("asyncStepExecutionIndex")
.build();
Variable START_TIME = ImmutableSimpleVariable. builder()
.name("startTime")
.build();
Variable SKIP_MANAGE_SERVICE_BROKER = ImmutableSimpleVariable. builder()
.name("skipManageServiceBroker")
.build();
Variable DELETE_IDLE_URIS = ImmutableSimpleVariable. builder()
.name("deleteIdleUris")
.defaultValue(false)
.build();
Variable USE_IDLE_URIS = ImmutableSimpleVariable. builder()
.name("useIdleUris")
.defaultValue(false)
.build();
Variable IS_SERVICE_UPDATED = ImmutableSimpleVariable. builder()
.name("isServiceUpdated")
.defaultValue(false)
.build();
Variable SKIP_UPDATE_CONFIGURATION_ENTRIES = ImmutableSimpleVariable. builder()
.name("skipUpdateConfigurationEntries")
.defaultValue(false)
.build();
Variable USER_PROPERTIES_CHANGED = ImmutableSimpleVariable. builder()
.name("vcapUserPropertiesChanged")
.defaultValue(false)
.build();
Variable APP_NEEDS_RESTAGE = ImmutableSimpleVariable. builder()
.name("appNeedsRestage")
.defaultValue(false)
.build();
Variable VCAP_APP_PROPERTIES_CHANGED = ImmutableSimpleVariable. builder()
.name("vcapAppPropertiesChanged")
.defaultValue(false)
.build();
Variable VCAP_SERVICES_PROPERTIES_CHANGED = ImmutableSimpleVariable. builder()
.name("vcapServicesPropertiesChanged")
.defaultValue(false)
.build();
Variable SHOULD_SKIP_SERVICE_REBINDING = ImmutableSimpleVariable. builder()
.name("shouldSkipServiceRebinding")
.defaultValue(false)
.build();
Variable DELETE_SERVICES = ImmutableSimpleVariable. builder()
.name("deleteServices")
.defaultValue(false)
.build();
Variable DELETE_SERVICE_KEYS = ImmutableSimpleVariable. builder()
.name("deleteServiceKeys")
.defaultValue(false)
.build();
Variable DELETE_SERVICE_BROKERS = ImmutableSimpleVariable. builder()
.name("deleteServiceBrokers")
.defaultValue(false)
.build();
Variable NO_START = ImmutableSimpleVariable. builder()
.name("noStart")
.defaultValue(false)
.build();
Variable KEEP_FILES = ImmutableSimpleVariable. builder()
.name("keepFiles")
.defaultValue(false)
.build();
Variable NO_CONFIRM = ImmutableSimpleVariable. builder()
.name("noConfirm")
.defaultValue(false)
.build();
Variable NO_RESTART_SUBSCRIBED_APPS = ImmutableSimpleVariable. builder()
.name("noRestartSubscribedApps")
.defaultValue(false)
.build();
Variable NO_FAIL_ON_MISSING_PERMISSIONS = ImmutableSimpleVariable. builder()
.name("noFailOnMissingPermissions")
.defaultValue(false)
.build();
Variable ABORT_ON_ERROR = ImmutableSimpleVariable. builder()
.name("abortOnError")
.defaultValue(false)
.build();
Variable KEEP_ORIGINAL_APP_NAMES_AFTER_DEPLOY = ImmutableSimpleVariable. builder()
.name("keepOriginalAppNamesAfterDeploy")
.defaultValue(false)
.build();
Variable SKIP_IDLE_START = ImmutableSimpleVariable. builder()
.name("skipIdleStart")
.defaultValue(false)
.build();
Variable EXECUTE_ONE_OFF_TASKS = ImmutableSimpleVariable. builder()
.name("executeOneOffTasks")
.build();
Variable SHOULD_UPLOAD_APPLICATION_CONTENT = ImmutableSimpleVariable. builder()
.name("shouldUploadApplicationContent")
.build();
Variable REBUILD_APP_ENV = ImmutableSimpleVariable. builder()
.name("rebuildAppEnv")
.build();
Variable BUILD_GUID = ImmutableSimpleVariable. builder()
.name("buildGuid")
.build();
Variable DEPLOYMENT_DESCRIPTOR = ImmutableJsonStringVariable. builder()
.name("mtaDeploymentDescriptor")
.type(Variable.typeReference(DeploymentDescriptor.class))
.build();
Variable DEPLOYMENT_DESCRIPTOR_WITH_SYSTEM_PARAMETERS = ImmutableJsonStringVariable. builder()
.name("mtaDeploymentDescriptorWithSystemParameters")
.type(Variable.typeReference(DeploymentDescriptor.class))
.build();
Variable COMPLETE_DEPLOYMENT_DESCRIPTOR = ImmutableJsonStringVariable. builder()
.name("completeMtaDeploymentDescriptor")
.type(Variable.typeReference(DeploymentDescriptor.class))
.build();
Variable APP_TO_PROCESS = ImmutableJsonStringVariable. builder()
.name("appToProcess")
.type(Variable.typeReference(CloudApplicationExtended.class))
.build();
Variable BINDING_NAME = ImmutableSimpleVariable. builder()
.name("bindingName")
.defaultValue(null)
.build();
Variable MTA_ARCHIVE_ELEMENTS = ImmutableJsonStringVariable. builder()
.name("mtaArchiveElements")
.type(Variable.typeReference(MtaArchiveElements.class))
.defaultValue(new MtaArchiveElements())
.build();
Variable SERVICE_TO_PROCESS = ImmutableJsonStringVariable. builder()
.name("serviceToProcess")
.type(Variable.typeReference(CloudServiceInstanceExtended.class))
.build();
Variable CLOUD_PACKAGE = ImmutableJsonStringVariable. builder()
.name("uploadedCloudPackage")
.type(Variable.typeReference(CloudPackage.class))
.build();
Variable HOOK_FOR_EXECUTION = ImmutableJsonStringVariable. builder()
.name("hookForExecution")
.type(Variable.typeReference(Hook.class))
.build();
Variable> APPS_TO_DEPLOY = ImmutableJsonBinaryVariable.> builder()
.name("appsToDeploy")
.type(new TypeReference<>() {
})
.build();
Variable> APPS_TO_RENAME = ImmutableJsonBinaryVariable.> builder()
.name("appsToRename")
.type(new TypeReference<>() {
})
.build();
Variable> CONFIGURATION_ENTRIES_TO_PUBLISH = ImmutableJsonBinaryVariable.> builder()
.name("configurationEntriesToPublish")
.type(new TypeReference<>() {
})
.build();
Variable> DELETED_ENTRIES = ImmutableJsonBinaryVariable.> builder()
.name("deletedEntries")
.type(new TypeReference<>() {
})
.defaultValue(Collections.emptyList())
.build();
Variable> PUBLISHED_ENTRIES = ImmutableJsonBinaryVariable.> builder()
.name("publishedEntries")
.type(new TypeReference<>() {
})
.build();
Variable CREATED_OR_UPDATED_SERVICE_BROKER = ImmutableJsonBinaryVariable. builder()
.name("createdOrUpdatedServiceBroker")
.type(Variable.typeReference(CloudServiceBroker.class))
.build();
Variable> CUSTOM_DOMAINS = ImmutableJsonBinaryVariable.> builder()
.name("customDomains")
.type(new TypeReference<>() {
})
.build();
Variable DEPLOYED_MTA = ImmutableJsonBinaryVariable. builder()
.name("deployedMta")
.type(Variable.typeReference(DeployedMta.class))
.build();
Variable> DEPLOYED_MTA_SERVICE_KEYS = ImmutableJsonBinaryVariable.> builder()
.name("deployedMtaServiceKeys")
.type(new TypeReference<>() {
})
.defaultValue(Collections.emptyList())
.build();
Variable EXISTING_APP = ImmutableJsonBinaryVariable. builder()
.name("existingApp")
.type(Variable.typeReference(CloudApplication.class))
.build();
Variable MODULE_TO_DEPLOY = ImmutableJsonBinaryVariable. builder()
.name("moduleToDeploy")
.type(Variable.typeReference(Module.class))
.build();
Variable> MTA_ARCHIVE_MODULES = ImmutableJsonBinaryVariable.> builder()
.name("mtaArchiveModules")
.type(new TypeReference<>() {
})
.build();
Variable> MTA_MODULES = ImmutableJsonBinaryVariable.> builder()
.name("mtaModules")
.type(new TypeReference<>() {
})
.build();
Variable