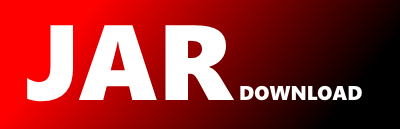
org.cloudgraph.store.lang.AssemblerSupport Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cloudgraph-common Show documentation
Show all versions of cloudgraph-common Show documentation
CloudGraph(tm) is a suite of Service Data Object (SDO) 2.1 services designed for relational and big-table style "cloud" databases, such as HBase and others.
/**
* CloudGraph Community Edition (CE) License
*
* This is a community release of CloudGraph, a dual-license suite of
* Service Data Object (SDO) 2.1 services designed for relational and
* big-table style "cloud" databases, such as HBase and others.
* This particular copy of the software is released under the
* version 2 of the GNU General Public License. CloudGraph was developed by
* TerraMeta Software, Inc.
*
* Copyright (c) 2013, TerraMeta Software, Inc. All rights reserved.
*
* General License information can be found below.
*
* This distribution may include materials developed by third
* parties. For license and attribution notices for these
* materials, please refer to the documentation that accompanies
* this distribution (see the "Licenses for Third-Party Components"
* appendix) or view the online documentation at
* .
*/
package org.cloudgraph.store.lang;
import java.util.ArrayList;
import java.util.List;
import java.util.Set;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import org.cloudgraph.store.service.GraphServiceException;
import org.plasma.query.collector.SelectionCollector;
import org.plasma.query.model.Where;
import org.plasma.sdo.PlasmaDataObject;
import org.plasma.sdo.PlasmaProperty;
import org.plasma.sdo.PlasmaType;
import org.plasma.sdo.access.DataAccessException;
import org.plasma.sdo.access.provider.common.PropertyPair;
import org.plasma.sdo.profile.KeyType;
import commonj.sdo.Property;
import commonj.sdo.Type;
/**
* Default assembler functionality without any shared objects related
* to graph assembly, such that it is usable by parallel tasks.
*
* @author Scott Cinnamond
* @since 0.6.2
*/
public abstract class AssemblerSupport {
private static Log log = LogFactory.getLog(AssemblerSupport.class);
protected SelectionCollector collector;
protected StatementFactory statementFactory;
protected StatementExecutor statementExecutor;
@SuppressWarnings("unused")
private AssemblerSupport() {}
public AssemblerSupport(SelectionCollector collector,
StatementFactory statementFactory, StatementExecutor statementExecutor) {
this.collector = collector;
this.statementFactory = statementFactory;
this.statementExecutor = statementExecutor;
}
public StatementFactory getStatementFactory() {
return statementFactory;
}
public StatementExecutor getStatementExecutor() {
return statementExecutor;
}
protected List getChildKeyPairs(PropertyPair pair)
{
List childKeyProps = new ArrayList();
PlasmaProperty supplier = pair.getProp().getKeySupplier();
if (supplier != null) {
PropertyPair childPair = new PropertyPair(supplier,
pair.getValue());
childPair.setValueProp(supplier);
childKeyProps.add(childPair);
}
else {
List childPkProps = ((PlasmaType)pair.getProp().getType()).findProperties(KeyType.primary);
if (childPkProps.size() == 1) {
childKeyProps.add(
new PropertyPair((PlasmaProperty)childPkProps.get(0),
pair.getValue()));
}
else
throwPriKeyError(childPkProps,
pair.getProp().getType(), pair.getProp());
}
return childKeyProps;
}
protected List getChildKeyPairs(PlasmaDataObject dataObject, PlasmaProperty prop)
{
PlasmaProperty opposite = (PlasmaProperty)prop.getOpposite();
if (opposite == null)
throw new DataAccessException("no opposite property found"
+ " - cannot map from many property, " + prop.toString());
List childKeyProps = new ArrayList();
List pkProps = ((PlasmaType)dataObject.getType()).findProperties(KeyType.primary);
if (pkProps.size() == 1) {
PlasmaProperty pkProp = (PlasmaProperty)pkProps.get(0);
Object value = dataObject.get(pkProp);
if (value != null) {
PropertyPair pair = new PropertyPair(opposite, value);
pair.setValueProp(pkProp);
childKeyProps.add(pair);
}
else
throw new GraphServiceException("no value found for key property, "
+ pkProp.toString());
}
else
throwPriKeyError(pkProps,
dataObject.getType(), prop);
return childKeyProps;
}
protected List> getPredicateResult(PlasmaType targetType, PlasmaProperty sourceProperty,
Set props,
List childKeyPairs) {
List> result = null;
Where where = this.collector.getPredicate(sourceProperty);
if (where == null) {
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy