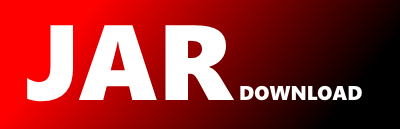
org.cloudgraph.hbase.mutation.Create Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cloudgraph-hbase Show documentation
Show all versions of cloudgraph-hbase Show documentation
CloudGraph(tm) is a suite of Service Data Object (SDO) 2.1 services designed for relational and big-table style "cloud" databases, such as HBase and others.
/**
* CloudGraph Community Edition (CE) License
*
* This is a community release of CloudGraph, a dual-license suite of
* Service Data Object (SDO) 2.1 services designed for relational and
* big-table style "cloud" databases, such as HBase and others.
* This particular copy of the software is released under the
* version 2 of the GNU General Public License. CloudGraph was developed by
* TerraMeta Software, Inc.
*
* Copyright (c) 2013, TerraMeta Software, Inc. All rights reserved.
*
* General License information can be found below.
*
* This distribution may include materials developed by third
* parties. For license and attribution notices for these
* materials, please refer to the documentation that accompanies
* this distribution (see the "Licenses for Third-Party Components"
* appendix) or view the online documentation at
* .
*/
package org.cloudgraph.hbase.mutation;
import java.io.IOException;
import java.util.List;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import org.cloudgraph.hbase.io.DistributedWriter;
import org.cloudgraph.hbase.io.RowWriter;
import org.cloudgraph.hbase.io.TableWriter;
import org.cloudgraph.hbase.service.HBaseDataConverter;
import org.cloudgraph.hbase.service.ServiceContext;
import org.plasma.sdo.PlasmaDataObject;
import org.plasma.sdo.PlasmaEdge;
import org.plasma.sdo.PlasmaNode;
import org.plasma.sdo.PlasmaProperty;
import org.plasma.sdo.PlasmaType;
import org.plasma.sdo.core.SnapshotMap;
import org.plasma.sdo.profile.KeyType;
import commonj.sdo.DataGraph;
import commonj.sdo.Property;
public class Create extends DefaultMutation implements Collector {
private static Log log = LogFactory.getLog(Create.class);
public Create(ServiceContext context, SnapshotMap snapshotMap, String username) {
super(context, snapshotMap, username);
}
/**
* Iterates over every property in the data object and pulls its value, as for 'create'
* operations, change summary does not register which properties are changed, only
* that the object is created.
*/
@Override
public void collect(DataGraph dataGraph,
PlasmaDataObject dataObject,
DistributedWriter graphWriter,
TableWriter tableWriter,
RowWriter rowWriter) throws IOException, IllegalAccessException
{
PlasmaType type = (PlasmaType)dataObject.getType();
if (log.isDebugEnabled())
log.debug(dataObject);
PlasmaNode dataNode = (PlasmaNode)dataObject;
this.updateKeys(dataObject, type, rowWriter);
this.updateOrigination(dataObject, type, rowWriter);
this.updateOptimistic(dataObject, type, rowWriter);
// For 'create' change summary has no changed property specifics
List properties = type.getProperties();
for (Property p : properties)
{
PlasmaProperty property = (PlasmaProperty)p;
if (property.isKey(KeyType.primary) && property.getType().isDataType())
continue; // processed above
if (property.getConcurrent() != null)
continue; // processed above
Object dataValue = dataObject.get(property);
if (dataValue == null)
continue; // nothing to create
byte[] valueBytes = null;
if (!property.getType().isDataType()) {
List edges = dataNode.getEdges(property);
// add the new value into graph state
this.addRowKeys(dataObject, dataNode, property, edges, graphWriter,
tableWriter, rowWriter);
List stateEdges = this.findUpdateEdges(dataNode, property,
edges, graphWriter, rowWriter);
if (stateEdges.size() > 0) {
rowWriter.getGraphState().addEdges(dataNode, stateEdges);
// create a formatted column value
// for this edge collection
valueBytes = this.createEdgeValueBytes(
dataNode, stateEdges, rowWriter);
this.updateCell(rowWriter, dataObject,
property, valueBytes);
}
}
else {
valueBytes = HBaseDataConverter.INSTANCE.toBytes(
property, dataValue);
this.updateCell(rowWriter, dataObject,
property, valueBytes);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy