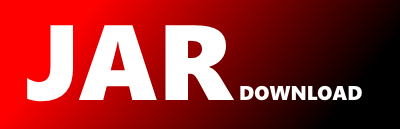
org.cloudgraph.hbase.client.HBaseDelete Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cloudgraph-hbase Show documentation
Show all versions of cloudgraph-hbase Show documentation
CloudGraph(tm) is a suite of Service Data Object (SDO) 2.1 services designed for relational and big-table style "cloud" databases, such as HBase and others.
The newest version!
package org.cloudgraph.hbase.client;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
import java.util.NavigableMap;
import java.util.TreeMap;
import org.cloudgraph.core.client.Cell;
import org.cloudgraph.core.client.Delete;
public class HBaseDelete extends HBaseMutation implements Delete {
private org.apache.hadoop.hbase.client.Delete delete;
public HBaseDelete(org.apache.hadoop.hbase.client.Delete delete) {
super(delete);
this.delete = delete;
}
public HBaseDelete(byte[] rowKey) {
this(new org.apache.hadoop.hbase.client.Delete(rowKey));
}
public org.apache.hadoop.hbase.client.Delete get() {
return this.delete;
}
@Override
public byte[] getRow() {
return this.delete.getRow();
}
@Override
public void setAttribute(String name, byte[] value) {
this.delete.setAttribute(name, value);
}
@Override
public byte[] getAttribute(String name) {
return this.delete.getAttribute(name);
}
@Override
public NavigableMap> getFamilyCellMap() {
NavigableMap> resultMap = new TreeMap<>();
NavigableMap> map = this.delete.getFamilyCellMap();
Iterator iter = map.keySet().iterator();
while (iter.hasNext()) {
byte[] key = iter.next();
List list = map.get(key);
List resultList = new ArrayList<>(list.size());
resultMap.put(key, resultList);
for (org.apache.hadoop.hbase.Cell cell : list) {
resultList.add(new HBaseCell(cell));
}
}
return resultMap;
}
@Override
public void addColumns(byte[] fam, byte[] qual) {
this.delete.addColumns(fam, qual);
}
}
|
© 2015 - 2025 Weber Informatics LLC | Privacy Policy