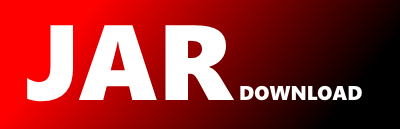
org.cloudgraph.hbase.client.HBaseMutation Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cloudgraph-hbase Show documentation
Show all versions of cloudgraph-hbase Show documentation
CloudGraph(tm) is a suite of Service Data Object (SDO) 2.1 services designed for relational and big-table style "cloud" databases, such as HBase and others.
The newest version!
package org.cloudgraph.hbase.client;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
import java.util.NavigableMap;
import java.util.TreeMap;
import org.cloudgraph.core.client.Cell;
import org.cloudgraph.core.client.Mutation;
public abstract class HBaseMutation extends HBaseRow implements Mutation {
private org.apache.hadoop.hbase.client.Mutation mutation;
protected HBaseMutation(org.apache.hadoop.hbase.client.Mutation mutation) {
super(mutation);
this.mutation = mutation;
}
@Override
public byte[] getRow() {
return this.mutation.getRow();
}
@Override
public void setAttribute(String name, byte[] value) {
this.mutation.setAttribute(name, value);
}
@Override
public byte[] getAttribute(String name) {
return this.mutation.getAttribute(name);
}
@Override
public NavigableMap> getFamilyCellMap() {
NavigableMap> resultMap = new TreeMap<>();
NavigableMap> map = this.mutation.getFamilyCellMap();
Iterator iter = map.keySet().iterator();
while (iter.hasNext()) {
byte[] key = iter.next();
List list = map.get(key);
List resultList = new ArrayList<>(list.size());
resultMap.put(key, resultList);
for (org.apache.hadoop.hbase.Cell cell : list) {
resultList.add(new HBaseCell(cell));
}
}
return resultMap;
}
}
|
© 2015 - 2025 Weber Informatics LLC | Privacy Policy