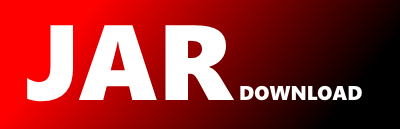
org.cloudgraph.rocksdb.connect.PooledConnectionFactory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cloudgraph-rocksdb Show documentation
Show all versions of cloudgraph-rocksdb Show documentation
CloudGraph(tm) is a suite of Service Data Object (SDO) 2.1 services designed for relational and big-table style "cloud" databases, such as HBase and others.
The newest version!
/**
* Copyright 2017 TerraMeta Software, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.cloudgraph.rocksdb.connect;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import org.apache.commons.pool2.BasePooledObjectFactory;
import org.apache.commons.pool2.ObjectPool;
import org.apache.commons.pool2.PooledObject;
import org.apache.commons.pool2.impl.DefaultPooledObject;
import org.cloudgraph.core.Connection;
import org.cloudgraph.core.ServiceContext;
import org.cloudgraph.store.mapping.StoreMappingContext;
/**
* Connection factory which creates pooled connections.
*
*
* The new HBase Client API changes removed the existing connection pool
* implementation and placed the responsibility of managing the lifecycle of
* connections on the caller. A pool is necessary as creating connections to the
* cluster via. zookeeper is fairly expensive.
*
* @author Scott Cinnamond
* @since 0.6.3
*/
public class PooledConnectionFactory extends BasePooledObjectFactory {
private static Log log = LogFactory.getLog(PooledConnectionFactory.class);
private ObjectPool pool;
public PooledConnectionFactory() {
super();
}
public void setPool(ObjectPool pool) {
this.pool = pool;
}
@Override
public Connection create() throws Exception {
if (log.isDebugEnabled())
log.debug("creating new hbase connection");
return new RocksDBConnection(this.pool);
}
@Override
public PooledObject wrap(Connection con) {
return new DefaultPooledObject(con);
}
@Override
public void destroyObject(PooledObject p) throws Exception {
if (log.isDebugEnabled())
log.debug("destroying connection" + p.getObject());
p.getObject().destroy();
super.destroyObject(p);
}
@Override
public boolean validateObject(PooledObject p) {
if (log.isDebugEnabled())
log.debug("validating connection" + p.getObject());
return super.validateObject(p);
}
@Override
public void activateObject(PooledObject p) throws Exception {
if (log.isDebugEnabled())
log.debug("activate connection" + p.getObject());
super.activateObject(p);
}
@Override
public void passivateObject(PooledObject p) throws Exception {
if (log.isDebugEnabled())
log.debug("passivate connection" + p.getObject());
super.passivateObject(p);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy