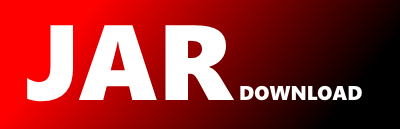
org.cloudgraph.test.socialgraph.actor.Node Maven / Gradle / Ivy
package org.cloudgraph.test.socialgraph.actor;
import java.util.Date;
import org.cloudgraph.test.socialgraph.actor.Edge;
import org.plasma.sdo.PlasmaDataObject;
/**
* In a social network all entities such as people, groups,
* events, topics, images, stories, etc. are represented as
* nodes. Nodes have some common characteristics. For
* example: title, description, unique URL, and Geo-location
* information. All nodes can be tagged and commented on.
*
* Generated interface representing the domain model entity Node. This SDO interface directly reflects the
* class (single or multiple) inheritance lattice of the source domain model(s) and is part of namespace http://cloudgraph.org/test/socialgraph/actor defined within the Configuration.
*
*
* Data Store Mapping:
* Corresponds to the physical data store entity ND.
*
*
* @see org.cloudgraph.test.socialgraph.actor.Edge Edge
*/
public interface Node extends PlasmaDataObject
{
/** The SDO namespace URI associated with the Type for this class. */
public static final String NAMESPACE_URI = "http://cloudgraph.org/test/socialgraph/actor";
/** The entity or Type logical name associated with this class. */
public static final String TYPE_NAME_NODE = "Node";
/**
* Represents the logical Property sourceEdge which is part of the Type Node.
*
*
* Data Store Mapping:
* Corresponds to the physical data store element ND.SE.
*/
public static final String SOURCE_EDGE = "sourceEdge";
/**
* Represents the logical Property targetEdge which is part of the Type Node.
*
*
* Data Store Mapping:
* Corresponds to the physical data store element ND.TE.
*/
public static final String TARGET_EDGE = "targetEdge";
/**
* Represents the logical Property name which is part of the Type Node.
*
*
* Data Store Mapping:
* Corresponds to the physical data store element ND.NM.
*/
public static final String NAME = "name";
/**
* Represents the logical Property description which is part of the Type Node.
*
*
* Data Store Mapping:
* Corresponds to the physical data store element ND.DR.
*/
public static final String DESCRIPTION = "description";
/**
* Represents the logical Property createdDate which is part of the Type Node.
*
*
* Data Store Mapping:
* Corresponds to the physical data store element ND.CD.
*/
public static final String CREATED_DATE = "createdDate";
/**
* Represents the logical Property modifiedDate which is part of the Type Node.
*
*
* Data Store Mapping:
* Corresponds to the physical data store element ND.MD.
*/
public static final String MODIFIED_DATE = "modifiedDate";
/**
* Returns true if the sourceEdge property is set.
* @return true if the sourceEdge property is set.
*/
public boolean isSetSourceEdge();
/**
* Unsets the sourceEdge property, clearing the underlying collection. The property will no longer be
* considered set.
*/
public void unsetSourceEdge();
/**
* Creates and returns a new instance of the given subclass Type for abstract base Type {@link Edge} automatically establishing a containment relationship through the object's reference property, sourceEdge.
* @param clss the subclass Type
* Returns a new instance of the given subclass Type for abstract base Type {@link Edge}.
*/
public Edge createSourceEdge(Class extends Edge> clss);
/**
* Returns an array of Edge set for the object's multi-valued property sourceEdge.
* @return an array of Edge set for the object's multi-valued property sourceEdge.
*/
public Edge[] getSourceEdge();
/**
* Returns the Edge set for the object's multi-valued property sourceEdge based on the given index.
* @param idx the index
* @return the Edge set for the object's multi-valued property sourceEdge based on the given index.
*/
public Edge getSourceEdge(int idx);
/**
* Returns a count for multi-valued property sourceEdge.
* @return a count for multi-valued property sourceEdge.
*/
public int getSourceEdgeCount();
/**
* Sets the given array of Type Edge for the object's multi-valued property sourceEdge.
* @param value the array value
*/
public void setSourceEdge(Edge[] value);
/**
* Adds the given value of Type Edge for the object's multi-valued property sourceEdge.
* @param value the value to add
*/
public void addSourceEdge(Edge value);
/**
* Removes the given value of Type Edge for the object's multi-valued property sourceEdge.
* @param value the value to remove
*/
public void removeSourceEdge(Edge value);
/**
* Returns true if the targetEdge property is set.
* @return true if the targetEdge property is set.
*/
public boolean isSetTargetEdge();
/**
* Unsets the targetEdge property, clearing the underlying collection. The property will no longer be
* considered set.
*/
public void unsetTargetEdge();
/**
* Creates and returns a new instance of the given subclass Type for abstract base Type {@link Edge} automatically establishing a containment relationship through the object's reference property, targetEdge.
* @param clss the subclass Type
* Returns a new instance of the given subclass Type for abstract base Type {@link Edge}.
*/
public Edge createTargetEdge(Class extends Edge> clss);
/**
* Returns an array of Edge set for the object's multi-valued property targetEdge.
* @return an array of Edge set for the object's multi-valued property targetEdge.
*/
public Edge[] getTargetEdge();
/**
* Returns the Edge set for the object's multi-valued property targetEdge based on the given index.
* @param idx the index
* @return the Edge set for the object's multi-valued property targetEdge based on the given index.
*/
public Edge getTargetEdge(int idx);
/**
* Returns a count for multi-valued property targetEdge.
* @return a count for multi-valued property targetEdge.
*/
public int getTargetEdgeCount();
/**
* Sets the given array of Type Edge for the object's multi-valued property targetEdge.
* @param value the array value
*/
public void setTargetEdge(Edge[] value);
/**
* Adds the given value of Type Edge for the object's multi-valued property targetEdge.
* @param value the value to add
*/
public void addTargetEdge(Edge value);
/**
* Removes the given value of Type Edge for the object's multi-valued property targetEdge.
* @param value the value to remove
*/
public void removeTargetEdge(Edge value);
/**
* Returns true if the name property is set.
* @return true if the name property is set.
*/
public boolean isSetName();
/**
* Unsets the name property, the value
* of the property of the object being set to the property's
* default value. The property will no longer be
* considered set.
*/
public void unsetName();
/**
* Returns the value of the name property.
* @return the value of the name property.
*/
public String getName();
/**
* Sets the value of the name property to the given value.
*
* Value Constraints:
* maxLength: 32
*/
public void setName(String value);
/**
* Returns true if the description property is set.
* @return true if the description property is set.
*/
public boolean isSetDescription();
/**
* Unsets the description property, the value
* of the property of the object being set to the property's
* default value. The property will no longer be
* considered set.
*/
public void unsetDescription();
/**
* Returns the value of the description property.
* @return the value of the description property.
*/
public String getDescription();
/**
* Sets the value of the description property to the given value.
*/
public void setDescription(String value);
/**
* Returns true if the createdDate property is set.
* @return true if the createdDate property is set.
*/
public boolean isSetCreatedDate();
/**
* Unsets the createdDate property, the value
* of the property of the object being set to the property's
* default value. The property will no longer be
* considered set.
*/
public void unsetCreatedDate();
/**
* Returns the value of the createdDate property.
* @return the value of the createdDate property.
*/
public Date getCreatedDate();
/**
* Sets the value of the createdDate property to the given value.
*/
public void setCreatedDate(Date value);
/**
* Returns true if the modifiedDate property is set.
* @return true if the modifiedDate property is set.
*/
public boolean isSetModifiedDate();
/**
* Unsets the modifiedDate property, the value
* of the property of the object being set to the property's
* default value. The property will no longer be
* considered set.
*/
public void unsetModifiedDate();
/**
* Returns the value of the modifiedDate property.
* @return the value of the modifiedDate property.
*/
public Date getModifiedDate();
/**
* Sets the value of the modifiedDate property to the given value.
*/
public void setModifiedDate(Date value);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy