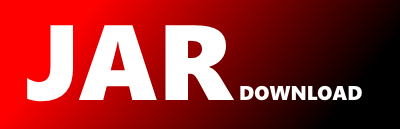
org.codefx.libfx.nesting.property.NestedStringProperty Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of LibFX Show documentation
Show all versions of LibFX Show documentation
LibFX provides usability classes for Java and JavaFX.
The newest version!
package org.codefx.libfx.nesting.property;
import javafx.beans.property.Property;
import javafx.beans.property.ReadOnlyBooleanProperty;
import javafx.beans.property.SimpleStringProperty;
import javafx.beans.property.StringProperty;
import org.codefx.libfx.nesting.Nesting;
/**
* A {@link StringProperty} which also implements {@link NestedProperty}.
*/
public class NestedStringProperty extends SimpleStringProperty implements NestedProperty {
private final NestedPropertyInternals internals;
// #begin CONSTUCTION
/**
* Creates a new property.
*
* @param nesting
* the nesting this property is based on
* @param innerObservableMissingBehavior
* defines the behavior for the case that the inner observable is missing
* @param bean
* the bean which owns this property; can be null
* @param name
* this property's name; can be null
*/
NestedStringProperty(
Nesting extends Property> nesting,
InnerObservableMissingBehavior innerObservableMissingBehavior,
Object bean,
String name) {
super(bean, name);
assert nesting != null : "The argument 'nesting' must not be null.";
assert innerObservableMissingBehavior != null : "The argument 'innerObservableMissingBehavior' must not be null.";
this.internals = new NestedPropertyInternals<>(
this, nesting, innerObservableMissingBehavior, this::setValueSuper);
internals.initializeBinding();
}
//#end CONSTUCTION
// #begin OVERRIDE SET(VALUE)
@Override
public void set(String newValue) {
internals.setCheckingMissingInnerObservable(newValue);
}
@Override
public void setValue(String newValue) {
internals.setCheckingMissingInnerObservable(newValue);
}
private void setValueSuper(String newValue) {
super.set(newValue);
}
// #end OVERRIDE SET(VALUE)
// #begin IMPLEMENTATION OF 'NestedProperty'
@Override
public ReadOnlyBooleanProperty innerObservablePresentProperty() {
return internals.innerObservablePresentProperty();
}
@Override
public boolean isInnerObservablePresent() {
return internals.innerObservablePresentProperty().get();
}
//#end IMPLEMENTATION OF 'NestedProperty'
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy