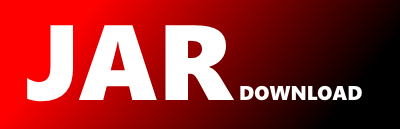
org.codegeny.beans.diff.DiffVisitor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of codegeny-beans Show documentation
Show all versions of codegeny-beans Show documentation
Library which provides meta-model, diffs, paths... for java beans
/*-
* #%L
* codegeny-beans
* %%
* Copyright (C) 2016 - 2018 Codegeny
* %%
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* #L%
*/
package org.codegeny.beans.diff;
import java.util.function.BiFunction;
import java.util.function.Function;
/**
* Visitor pattern for {@link Diff}
s.
*
* @param The type of {@link Diff}<T>
.
* @param The type of the result.
* @author Xavier DURY
*/
public interface DiffVisitor {
/**
* Create a visitor which process all type of {@link Diff} with the same function.
*
* @param function The function.
* @param The type of {@link Diff}<T>
.
* @param The type of the result.
* @return A visitor.
*/
static DiffVisitor adapter(Function super Diff, ? extends R> function) {
return new DiffVisitor() {
/**
* {@inheritDoc}
*/
@Override
public R visitBean(BeanDiff bean) {
return function.apply(bean);
}
/**
* {@inheritDoc}
*/
@Override
public R visitList(ListDiff list) {
return function.apply(list);
}
/**
* {@inheritDoc}
*/
@Override
public R visitMap(MapDiff map) {
return function.apply(map);
}
/**
* {@inheritDoc}
*/
@Override
public R visitSimple(SimpleDiff simple) {
return function.apply(simple);
}
};
}
/**
* Visitor which returns the {@link Diff} itself.
*
* @param The type of {@link Diff}<T>
.
* @return A visitor.
*/
static DiffVisitor> identity() {
return adapter(Function.identity());
}
/**
* Visitor which combine the result of the current visitor and the result of the given visitor.
*
* @param that The other visitor.
* @param combiner The combining function.
* @param The other other visitor return type.
* @param The type of the combined values (<R> and <X>).
* @return A visitor.
*/
default DiffVisitor andThen(DiffVisitor that, BiFunction super R, ? super X, ? extends Y> combiner) {
return adapter(diff -> combiner.apply(diff.accept(this), diff.accept(that)));
}
/**
* Visit a {@link BeanDiff}<T>
*
* @param bean The bean to visit.
* @return The computed result.
*/
R visitBean(BeanDiff bean);
/**
* Visit a {@link ListDiff}<T, E>
*
* @param list The list to visit.
* @param The type of elements.
* @return The computed result.
*/
R visitList(ListDiff list);
/**
* Visit a {@link MapDiff}<T, K, V>
*
* @param map The map to visit.
* @param The type of the map key.
* @param The type of the map value.
* @return The computed result.
*/
R visitMap(MapDiff map);
/**
* Visit a {@link SimpleDiff}<T>
*
* @param simple The simple value to visit.
* @return The computed result.
*/
R visitSimple(SimpleDiff simple);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy