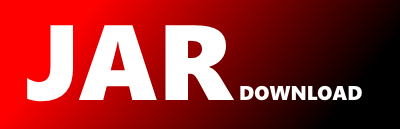
org.codehaus.cake.cache.policy.spi.AbstractArrayReplacementPolicy Maven / Gradle / Ivy
/*
* Copyright 2008 Kasper Nielsen.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* http://cake.codehaus.org/LICENSE
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package org.codehaus.cake.cache.policy.spi;
import java.util.ArrayList;
import org.codehaus.cake.attribute.IntAttribute;
import org.codehaus.cake.cache.CacheEntry;
/**
*
* @author Kasper Nielsen
* @version $Id: CacheLifecycle.java 511 2007-12-13 14:37:02Z kasper $
* @param
* the type of keys maintained by the cache
* @param
* the type of mapped values
*/
public abstract class AbstractArrayReplacementPolicy extends AbstractReplacementPolicy {
private final IntAttribute index = new IntAttribute("index", 0) {};
private final ArrayList> list = new ArrayList>();
public AbstractArrayReplacementPolicy() {
attachToEntry(index);
}
/** {@inheritDoc} */
public boolean add(CacheEntry entry) {
add0(entry);
return true;
}
protected int add0(CacheEntry entry) {
int i = list.size();
index.set(entry, i);
list.add(entry);
return i;
}
/** {@inheritDoc} */
public void clear() {
list.clear();
}
protected CacheEntry getFromIndex(int index) {
return list.get(index);
}
protected int getIndexOf(CacheEntry entry) {
return index.get(entry);
}
/** {@inheritDoc} */
public void remove(CacheEntry entry) {
remove0(entry);
}
protected CacheEntry removeByIndex(int index) {
CacheEntry entry = list.get(index);
remove0(entry);
return entry;
}
protected int remove0(CacheEntry entry) {
int lastIndex = list.size() - 1;
CacheEntry last = list.remove(lastIndex);
if (entry != last) {
int i = index.get(entry);
list.set(i, last);
index.set(last, i);
swap(lastIndex, i);
}
return lastIndex;
}
/** {@inheritDoc} */
public CacheEntry replace(CacheEntry previous, CacheEntry newEntry) {
replace0(previous, newEntry);
return newEntry;
}
protected void replace0(CacheEntry previous, CacheEntry newEntry) {
int i = index.get(previous);
index.set(newEntry, i);
list.set(i, newEntry);
}
protected int size() {
return list.size();
}
protected void swap(int prevIndex, int newIndex) {}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy