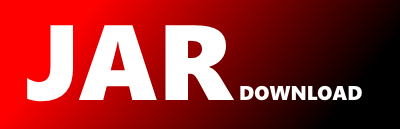
org.fabric3.policy.resolver.ImplementationPolicyResolverImpl Maven / Gradle / Ivy
/*
* Fabric3
* Copyright (c) 2009-2013 Metaform Systems
*
* Fabric3 is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as
* published by the Free Software Foundation, either version 3 of
* the License, or (at your option) any later version, with the
* following exception:
*
* Linking this software statically or dynamically with other
* modules is making a combined work based on this software.
* Thus, the terms and conditions of the GNU General Public
* License cover the whole combination.
*
* As a special exception, the copyright holders of this software
* give you permission to link this software with independent
* modules to produce an executable, regardless of the license
* terms of these independent modules, and to copy and distribute
* the resulting executable under terms of your choice, provided
* that you also meet, for each linked independent module, the
* terms and conditions of the license of that module. An
* independent module is a module which is not derived from or
* based on this software. If you modify this software, you may
* extend this exception to your version of the software, but
* you are not obligated to do so. If you do not wish to do so,
* delete this exception statement from your version.
*
* Fabric3 is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty
* of MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE.
* See the GNU General Public License for more details.
*
* You should have received a copy of the
* GNU General Public License along with Fabric3.
* If not, see .
*/
package org.fabric3.policy.resolver;
import javax.xml.namespace.QName;
import java.util.Collections;
import java.util.HashSet;
import java.util.LinkedHashSet;
import java.util.Set;
import org.fabric3.model.type.component.ComponentDefinition;
import org.fabric3.model.type.component.ComponentType;
import org.fabric3.model.type.component.Implementation;
import org.fabric3.model.type.definitions.ImplementationType;
import org.fabric3.model.type.definitions.Intent;
import org.fabric3.model.type.definitions.PolicySet;
import org.fabric3.policy.infoset.PolicyEvaluator;
import org.fabric3.spi.generator.policy.PolicyRegistry;
import org.fabric3.spi.generator.policy.PolicyResolutionException;
import org.fabric3.spi.lcm.LogicalComponentManager;
import org.fabric3.spi.model.instance.LogicalComponent;
import org.fabric3.spi.model.instance.LogicalOperation;
import org.fabric3.spi.model.instance.LogicalScaArtifact;
import org.oasisopen.sca.annotation.Reference;
/**
*
*/
public class ImplementationPolicyResolverImpl extends AbstractPolicyResolver implements ImplementationPolicyResolver {
public ImplementationPolicyResolverImpl(@Reference PolicyRegistry policyRegistry,
@Reference LogicalComponentManager lcm,
@Reference PolicyEvaluator policyEvaluator) {
super(policyRegistry, lcm, policyEvaluator);
}
public IntentPair resolveIntents(LogicalComponent> component, LogicalOperation operation) throws PolicyResolutionException {
Implementation> implementation = component.getDefinition().getImplementation();
QName type = implementation.getType();
ImplementationType implementationType = policyRegistry.getDefinition(type, ImplementationType.class);
Set mayProvidedIntents;
if (implementationType == null) {
// tolerate not having a registered implementation type definition
mayProvidedIntents = Collections.emptySet();
} else {
mayProvidedIntents = implementationType.getMayProvide();
}
Set requiredIntents = getRequestedIntents(component, operation);
Set intentsToBeProvided = new LinkedHashSet();
for (Intent intent : requiredIntents) {
if (mayProvidedIntents.contains(intent.getName())) {
intentsToBeProvided.add(intent);
}
}
return new IntentPair(requiredIntents, intentsToBeProvided);
}
public Set resolvePolicySets(LogicalComponent> component, LogicalOperation operation) throws PolicyResolutionException {
Implementation> implementation = component.getDefinition().getImplementation();
QName type = implementation.getType();
ImplementationType implementationType = policyRegistry.getDefinition(type, ImplementationType.class);
Set alwaysProvidedIntents = new LinkedHashSet();
Set mayProvidedIntents = new LinkedHashSet();
if (implementationType != null) {
// tolerate not having a registered implementation type definition
alwaysProvidedIntents = implementationType.getAlwaysProvide();
mayProvidedIntents = implementationType.getMayProvide();
}
Set requiredIntents = getRequestedIntents(component, operation);
Set requiredIntentsCopy = new HashSet(requiredIntents);
// Remove intents that are provided
for (Intent intent : requiredIntentsCopy) {
QName intentName = intent.getName();
if (alwaysProvidedIntents.contains(intentName) || mayProvidedIntents.contains(intentName)) {
requiredIntents.remove(intent);
}
}
Set policySets = aggregatePolicySets(operation, component);
if (requiredIntents.isEmpty() && policySets.isEmpty()) {
// short-circuit intent resolution
return Collections.emptySet();
}
Set policies;
if (!policySets.isEmpty()) {
// resolve intents against specified policy sets
policies = new LinkedHashSet();
for (QName name : policySets) {
PolicySet policySet = policyRegistry.getDefinition(name, PolicySet.class);
policies.add(policySet);
}
for (Intent intent : requiredIntents) {
boolean resolved = false;
for (PolicySet policy : policies) {
if (policy.doesProvide(intent.getName())) {
resolved = true;
break;
}
}
if (!resolved) {
throw new PolicyResolutionException("Intent not satisfied: " + intent.getName());
}
}
} else {
policies = resolvePolicies(requiredIntents, component);
if (!requiredIntents.isEmpty()) {
throw new IntentResolutionException("Unable to resolve all intents", requiredIntents);
}
for (QName name : policySets) {
PolicySet policySet = policyRegistry.getDefinition(name, PolicySet.class);
policies.add(policySet);
}
}
return policies;
}
private Set getRequestedIntents(LogicalComponent> logicalComponent, LogicalOperation operation) throws PolicyResolutionException {
// Aggregate all the intents from the ancestors
Set intentNames = new LinkedHashSet();
intentNames.addAll(operation.getIntents());
ComponentDefinition extends Implementation>> definition = logicalComponent.getDefinition();
Implementation> implementation = definition.getImplementation();
ComponentType componentType = implementation.getComponentType();
intentNames.addAll(implementation.getIntents());
intentNames.addAll(componentType.getIntents());
intentNames.addAll(aggregateIntents(logicalComponent));
// Expand all the profile intents
Set requiredIntents = resolveIntents(intentNames);
// Remove intents not applicable to the artifact
filterInvalidIntents(Intent.IMPLEMENTATION, requiredIntents);
filterMutuallyExclusiveIntents(requiredIntents);
return requiredIntents;
}
/**
* Aggregate policies from ancestors.
*
* @param operation the operation
* @param logicalComponent the target component
* @return the aggregated policy sets
*/
protected Set aggregatePolicySets(LogicalOperation operation, LogicalComponent> logicalComponent) {
LogicalScaArtifact> temp = operation;
Set policySetNames = new LinkedHashSet();
while (temp != null) {
policySetNames.addAll(temp.getPolicySets());
temp = temp.getParent();
}
policySetNames.addAll(logicalComponent.getPolicySets());
return policySetNames;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy