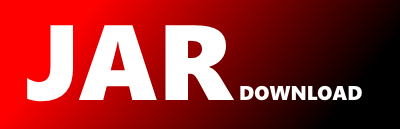
groovyx.gpars.dataflow.Dataflows Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gpars Show documentation
Show all versions of gpars Show documentation
The Groovy and Java high-level concurrency library offering actors, dataflow, CSP, agents, parallel collections, fork/join and more
// GPars - Groovy Parallel Systems
//
// Copyright © 2008-11 The original author or authors
//
// Licensed under the Apache License, Version 2.0 (the "License");
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// http://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
package groovyx.gpars.dataflow;
import groovy.lang.Closure;
import groovy.lang.GroovyObjectSupport;
import groovy.lang.MissingMethodException;
import org.codehaus.groovy.runtime.InvokerInvocationException;
import java.util.Iterator;
import java.util.Map;
import java.util.concurrent.ConcurrentHashMap;
import java.util.concurrent.ConcurrentMap;
/**
* Convenience class that makes working with DataflowVariables more comfortable.
*
* See the implementation of groovyx.gpars.samples.dataflow.DemoDataflows for a full example.
*
* A Dataflows instance is a bean with properties of type DataflowVariable.
* Property access is relayed to the access methods of DataflowVariable.
* Each property is initialized lazily the first time it is accessed.
* Non-String named properties can be also accessed using array-like indexing syntax
* This allows a rather compact usage of DataflowVariables like
*
*
* final df = new Dataflows()
* start { df[0] = df.x + df.y }
* start { df.x = 10 }
* start { df.y = 5 }
* assert 15 == df[0]
*
*
* @author Vaclav Pech, Dierk Koenig, Alex Tkachman
* Date: Sep 3, 2009
*/
public final class Dataflows extends GroovyObjectSupport {
private static final DataflowVariable
© 2015 - 2025 Weber Informatics LLC | Privacy Policy