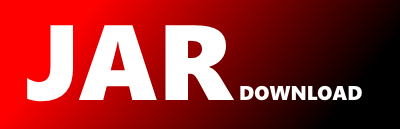
groovyx.gpars.actor.impl.MessageStream Maven / Gradle / Ivy
// GPars - Groovy Parallel Systems
//
// Copyright © 2008-10 The original author or authors
//
// Licensed under the Apache License, Version 2.0 (the "License");
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// http://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
package groovyx.gpars.actor.impl;
import groovy.time.Duration;
import groovyx.gpars.actor.Actor;
import groovyx.gpars.actor.ActorMessage;
import groovyx.gpars.remote.RemoteConnection;
import groovyx.gpars.remote.RemoteHost;
import groovyx.gpars.serial.RemoteSerialized;
import groovyx.gpars.serial.SerialMsg;
import groovyx.gpars.serial.WithSerialId;
import java.util.concurrent.TimeUnit;
import java.util.concurrent.locks.LockSupport;
/**
* Represents a stream of messages and forms the base class for actors
*
* @author Alex Tkachman, Vaclav Pech, Dierk Koenig
*/
public abstract class MessageStream extends WithSerialId {
private static final long serialVersionUID = 7644465423857532477L;
/**
* Send message to stream and return immediately
*
* @param message message to send
* @return always return message stream itself
*/
public abstract MessageStream send(Object message);
/**
* Convenience method for send(new Object()).
*
* @return always return message stream itself
*/
public final MessageStream send() {
return send(new Object());
}
/**
* Send message to stream and return immediately. Allows to specify an arbitrary actor to send replies to.
* By default replies are sent to the originator (sender) of each message, however, when a different actor
* is specified as the optional second argument to the send() method, this supplied actor will receive the replies instead.
*
* @param message message to send
* @param replyTo where to send reply
* @param type of message accepted by the stream
* @return always return message stream itself
*/
public final MessageStream send(final T message, final MessageStream replyTo) {
return send(new ActorMessage(message, replyTo));
}
/**
* Same as send
*
* @param message to send
* @return original stream
*/
public final MessageStream leftShift(final T message) {
return send(message);
}
/**
* Same as send
*
* @param message to send
* @return original stream
*/
public final MessageStream call(final T message) {
return send(message);
}
/**
* Sends a message and waits for a reply.
* Returns the reply or throws an IllegalStateException, if the target actor cannot reply.
*
* @param message message to send
* @return The message that came in reply to the original send.
* @throws InterruptedException if interrupted while waiting
*/
public final V sendAndWait(final T message) throws InterruptedException {
final ResultWaiter to = new ResultWaiter();
send(new ActorMessage(message, to));
return to.getResult();
}
/**
* Sends a message and waits for a reply. Timeouts after the specified timeout. In case of timeout returns null.
* Returns the reply or throws an IllegalStateException, if the target actor cannot reply.
*
* @param message message to send
* @param timeout timeout
* @param units units
* @return The message that came in reply to the original send.
* @throws InterruptedException if interrupted while waiting
*/
public final Object sendAndWait(final T message, final long timeout, final TimeUnit units) throws InterruptedException {
final ResultWaiter
© 2015 - 2025 Weber Informatics LLC | Privacy Policy