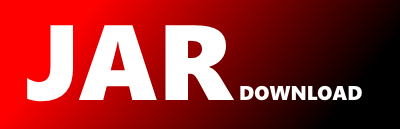
griffon.javafx.MappingBindingsExtension.groovy Maven / Gradle / Ivy
/*
* SPDX-License-Identifier: Apache-2.0
*
* Copyright 2008-2021 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package griffon.javafx
import griffon.javafx.beans.binding.MappingBindings
import groovy.transform.CompileStatic
import javafx.beans.binding.BooleanBinding
import javafx.beans.binding.DoubleBinding
import javafx.beans.binding.FloatBinding
import javafx.beans.binding.IntegerBinding
import javafx.beans.binding.LongBinding
import javafx.beans.binding.ObjectBinding
import javafx.beans.binding.StringBinding
import javafx.beans.value.ObservableBooleanValue
import javafx.beans.value.ObservableDoubleValue
import javafx.beans.value.ObservableFloatValue
import javafx.beans.value.ObservableIntegerValue
import javafx.beans.value.ObservableLongValue
import javafx.beans.value.ObservableObjectValue
import javafx.beans.value.ObservableStringValue
import javafx.beans.value.ObservableValue
import javafx.collections.ObservableList
import javax.annotation.Nonnull
import javax.annotation.Nullable
import java.util.function.BiFunction
import java.util.function.Function
import java.util.function.Supplier
/**
* @author Andres Almiray
* @since 2.13.0
*/
@CompileStatic
final class MappingBindingsExtension {
@Nonnull
static ObjectBinding mapAsObject(@Nonnull ObservableValue observable, @Nonnull Function mapper) {
MappingBindings.mapAsObject(observable, mapper)
}
@Nonnull
static ObjectBinding mapAsObject(
@Nonnull ObservableValue observable, @Nonnull ObservableValue> mapper) {
MappingBindings.mapAsObject(observable, mapper)
}
@Nonnull
static BooleanBinding mapAsBoolean(
@Nonnull ObservableValue observable, @Nonnull Function mapper) {
MappingBindings.mapAsBoolean(observable, mapper)
}
@Nonnull
static BooleanBinding mapAsBoolean(
@Nonnull ObservableValue observable, @Nonnull ObservableValue> mapper) {
MappingBindings.mapAsBoolean(observable, mapper)
}
@Nonnull
static IntegerBinding mapAsInteger(
@Nonnull ObservableValue observable, @Nonnull Function mapper) {
MappingBindings.mapAsInteger(observable, mapper)
}
@Nonnull
static IntegerBinding mapAsInteger(
@Nonnull ObservableValue observable, @Nonnull ObservableValue> mapper) {
MappingBindings.mapAsInteger(observable, mapper)
}
@Nonnull
static LongBinding mapAsLong(@Nonnull ObservableValue observable, @Nonnull Function mapper) {
MappingBindings.mapAsLong(observable, mapper)
}
@Nonnull
static LongBinding mapAsLong(
@Nonnull ObservableValue observable, @Nonnull ObservableValue> mapper) {
MappingBindings.mapAsLong(observable, mapper)
}
@Nonnull
static FloatBinding mapAsFloat(@Nonnull ObservableValue observable, @Nonnull Function mapper) {
MappingBindings.mapAsFloat(observable, mapper)
}
@Nonnull
static FloatBinding mapAsFloat(
@Nonnull ObservableValue observable, @Nonnull ObservableValue> mapper) {
MappingBindings.mapAsFloat(observable, mapper)
}
@Nonnull
static DoubleBinding mapAsDouble(@Nonnull ObservableValue observable, @Nonnull Function mapper) {
MappingBindings.mapAsDouble(observable, mapper)
}
@Nonnull
static DoubleBinding mapAsDouble(
@Nonnull ObservableValue observable, @Nonnull ObservableValue> mapper) {
MappingBindings.mapAsDouble(observable, mapper)
}
@Nonnull
static StringBinding mapAsString(@Nonnull ObservableValue observable, @Nonnull Function mapper) {
MappingBindings.mapAsString(observable, mapper)
}
@Nonnull
static StringBinding mapAsString(
@Nonnull ObservableValue observable, @Nonnull ObservableValue> mapper) {
MappingBindings.mapAsString(observable, mapper)
}
@Nonnull
static ObjectBinding mapToObject(@Nonnull ObservableStringValue observable) {
MappingBindings.mapToObject(observable)
}
@Nonnull
static ObjectBinding mapToObject(@Nonnull ObservableBooleanValue observable) {
MappingBindings.mapToObject(observable)
}
@Nonnull
static ObjectBinding mapToObject(@Nonnull ObservableIntegerValue observable) {
MappingBindings.mapToObject(observable)
}
@Nonnull
static ObjectBinding mapToObject(@Nonnull ObservableLongValue observable) {
MappingBindings.mapToObject(observable)
}
@Nonnull
static ObjectBinding mapToObject(@Nonnull ObservableFloatValue observable) {
MappingBindings.mapToObject(observable)
}
@Nonnull
static ObjectBinding mapToObject(@Nonnull ObservableDoubleValue observable) {
MappingBindings.mapToObject(observable)
}
@Nonnull
static BooleanBinding mapToBoolean(@Nonnull ObservableObjectValue observable) {
MappingBindings.mapToBoolean(observable)
}
@Nonnull
static IntegerBinding mapToInteger(@Nonnull ObservableObjectValue observable) {
MappingBindings.mapToInteger(observable)
}
@Nonnull
static LongBinding mapToLong(@Nonnull ObservableObjectValue observable) {
MappingBindings.mapToLong(observable)
}
@Nonnull
static FloatBinding mapToFloat(@Nonnull ObservableObjectValue observable) {
MappingBindings.mapToFloat(observable)
}
@Nonnull
static DoubleBinding mapToDouble(@Nonnull ObservableObjectValue observable) {
MappingBindings.mapToDouble(observable)
}
@Nonnull
static StringBinding mapToString(@Nonnull ObservableObjectValue observable) {
MappingBindings.mapToString(observable)
}
@Nonnull
static ObservableList mapList(@Nonnull ObservableList super S> source, @Nonnull Function mapper) {
MappingBindings.mapList(source, mapper)
}
@Nonnull
static ObservableList mapList(
@Nonnull ObservableList source, @Nonnull ObservableValue> mapper) {
MappingBindings.mapList(source, mapper)
}
@Nonnull
static ObjectBinding mapObject(
@Nonnull ObservableValue observable, @Nonnull Function super T, ? extends R> mapper) {
MappingBindings.mapObject(observable, mapper)
}
@Nonnull
static ObjectBinding mapObject(
@Nonnull ObservableValue observable,
@Nonnull Function super T, ? extends R> mapper, @Nullable R defaultValue) {
MappingBindings.mapObject(observable, mapper, defaultValue)
}
@Nonnull
static ObjectBinding mapObject(
@Nonnull ObservableValue observable,
@Nonnull Function super T, ? extends R> mapper, @Nonnull Supplier supplier) {
MappingBindings.mapObject(observable, mapper, supplier)
}
@Nonnull
static ObjectBinding mapObject(
@Nonnull ObservableValue observable, @Nonnull ObservableValue> mapper) {
MappingBindings.mapObject(observable, mapper)
}
@Nonnull
static ObjectBinding mapObject(
@Nonnull ObservableValue observable,
@Nonnull ObservableValue> mapper, @Nullable R defaultValue) {
MappingBindings.mapObject(observable, mapper, defaultValue)
}
@Nonnull
static ObjectBinding mapObject(
@Nonnull ObservableValue observable,
@Nonnull ObservableValue> mapper, @Nonnull Supplier supplier) {
MappingBindings.mapObject(observable, mapper, supplier)
}
@Nonnull
static BooleanBinding mapBoolean(
@Nonnull ObservableValue observable, @Nonnull Function mapper) {
MappingBindings.mapBoolean(observable, mapper)
}
@Nonnull
static BooleanBinding mapBoolean(
@Nonnull ObservableValue observable,
@Nonnull Function mapper, @Nonnull Boolean defaultValue) {
MappingBindings.mapBoolean(observable, mapper, defaultValue)
}
@Nonnull
static BooleanBinding mapBoolean(
@Nonnull ObservableValue observable,
@Nonnull Function mapper, @Nonnull Supplier supplier) {
MappingBindings.mapBoolean(observable, mapper, supplier)
}
@Nonnull
static BooleanBinding mapBoolean(
@Nonnull ObservableValue observable, @Nonnull ObservableValue> mapper) {
MappingBindings.mapBoolean(observable, mapper)
}
@Nonnull
static BooleanBinding mapBoolean(
@Nonnull ObservableValue observable,
@Nonnull ObservableValue> mapper, @Nonnull Boolean defaultValue) {
MappingBindings.mapBoolean(observable, mapper, defaultValue)
}
@Nonnull
static BooleanBinding mapBoolean(
@Nonnull ObservableValue observable,
@Nonnull ObservableValue> mapper, @Nonnull Supplier supplier) {
MappingBindings.mapBoolean(observable, mapper, supplier)
}
@Nonnull
static IntegerBinding mapInteger(
@Nonnull ObservableValue observable, @Nonnull Function mapper) {
MappingBindings.mapInteger(observable, mapper)
}
@Nonnull
static IntegerBinding mapInteger(
@Nonnull ObservableValue observable,
@Nonnull Function mapper, @Nonnull Integer defaultValue) {
MappingBindings.mapInteger(observable, mapper, defaultValue)
}
@Nonnull
static IntegerBinding mapInteger(
@Nonnull ObservableValue observable,
@Nonnull Function mapper, @Nonnull Supplier supplier) {
MappingBindings.mapInteger(observable, mapper, supplier)
}
@Nonnull
static IntegerBinding mapInteger(
@Nonnull ObservableValue observable, @Nonnull ObservableValue> mapper) {
MappingBindings.mapInteger(observable, mapper)
}
@Nonnull
static IntegerBinding mapInteger(
@Nonnull ObservableValue observable,
@Nonnull ObservableValue> mapper, @Nonnull Integer defaultValue) {
MappingBindings.mapInteger(observable, mapper, defaultValue)
}
@Nonnull
static IntegerBinding mapInteger(
@Nonnull ObservableValue observable,
@Nonnull ObservableValue> mapper, @Nonnull Supplier supplier) {
MappingBindings.mapInteger(observable, mapper, supplier)
}
@Nonnull
static LongBinding mapLong(@Nonnull ObservableValue observable, @Nonnull Function mapper) {
MappingBindings.mapLong(observable, mapper)
}
@Nonnull
static LongBinding mapLong(
@Nonnull ObservableValue observable, @Nonnull Function mapper, @Nonnull Long defaultValue) {
MappingBindings.mapLong(observable, mapper, defaultValue)
}
@Nonnull
static LongBinding mapLong(
@Nonnull ObservableValue observable,
@Nonnull Function mapper, @Nonnull Supplier supplier) {
MappingBindings.mapLong(observable, mapper, supplier)
}
@Nonnull
static LongBinding mapLong(
@Nonnull ObservableValue observable, @Nonnull ObservableValue> mapper) {
MappingBindings.mapLong(observable, mapper)
}
@Nonnull
static LongBinding mapLong(
@Nonnull ObservableValue observable,
@Nonnull ObservableValue> mapper, @Nonnull Long defaultValue) {
MappingBindings.mapLong(observable, mapper, defaultValue)
}
@Nonnull
static LongBinding mapLong(
@Nonnull ObservableValue observable,
@Nonnull ObservableValue> mapper, @Nonnull Supplier supplier) {
MappingBindings.mapLong(observable, mapper, supplier)
}
@Nonnull
static FloatBinding mapFloat(@Nonnull ObservableValue observable, @Nonnull Function mapper) {
MappingBindings.mapFloat(observable, mapper)
}
@Nonnull
static FloatBinding mapFloat(
@Nonnull ObservableValue observable,
@Nonnull Function mapper, @Nonnull Float defaultValue) {
MappingBindings.mapFloat(observable, mapper, defaultValue)
}
@Nonnull
static FloatBinding mapFloat(
@Nonnull ObservableValue observable,
@Nonnull Function mapper, @Nonnull Supplier supplier) {
MappingBindings.mapFloat(observable, mapper, supplier)
}
@Nonnull
static FloatBinding mapFloat(
@Nonnull ObservableValue observable, @Nonnull ObservableValue> mapper) {
MappingBindings.mapFloat(observable, mapper)
}
@Nonnull
static FloatBinding mapFloat(
@Nonnull ObservableValue observable,
@Nonnull ObservableValue> mapper, @Nullable Float defaultValue) {
MappingBindings.mapFloat(observable, mapper, defaultValue)
}
@Nonnull
static FloatBinding mapFloat(
@Nonnull ObservableValue observable,
@Nonnull ObservableValue> mapper, @Nonnull Supplier supplier) {
MappingBindings.mapFloat(observable, mapper, supplier)
}
@Nonnull
static DoubleBinding mapDouble(
@Nonnull ObservableValue observable, @Nonnull Function mapper) {
MappingBindings.mapDouble(observable, mapper)
}
@Nonnull
static DoubleBinding mapDouble(
@Nonnull ObservableValue observable,
@Nonnull Function mapper, @Nullable Double defaultValue) {
MappingBindings.mapDouble(observable, mapper, defaultValue)
}
@Nonnull
static DoubleBinding mapDouble(
@Nonnull ObservableValue observable,
@Nonnull Function mapper, @Nonnull Supplier supplier) {
MappingBindings.mapDouble(observable, mapper, supplier)
}
@Nonnull
static DoubleBinding mapDouble(
@Nonnull ObservableValue observable, @Nonnull ObservableValue> mapper) {
MappingBindings.mapDouble(observable, mapper)
}
@Nonnull
static DoubleBinding mapDouble(
@Nonnull ObservableValue observable,
@Nonnull ObservableValue> mapper, @Nonnull Double defaultValue) {
MappingBindings.mapDouble(observable, mapper, defaultValue)
}
@Nonnull
static DoubleBinding mapDouble(
@Nonnull ObservableValue observable,
@Nonnull ObservableValue> mapper, @Nonnull Supplier supplier) {
MappingBindings.mapDouble(observable, mapper, supplier)
}
@Nonnull
static StringBinding mapString(
@Nonnull ObservableValue observable, @Nonnull Function mapper) {
MappingBindings.mapString(observable, mapper)
}
@Nonnull
static StringBinding mapString(
@Nonnull ObservableValue observable,
@Nonnull Function mapper, @Nonnull String defaultValue) {
MappingBindings.mapString(observable, mapper, defaultValue)
}
@Nonnull
static StringBinding mapString(
@Nonnull ObservableValue observable,
@Nonnull Function mapper, @Nonnull Supplier supplier) {
MappingBindings.mapString(observable, mapper, supplier)
}
@Nonnull
static StringBinding mapString(
@Nonnull ObservableValue observable, @Nonnull ObservableValue> mapper) {
MappingBindings.mapString(observable, mapper)
}
@Nonnull
static StringBinding mapString(
@Nonnull ObservableValue observable,
@Nonnull ObservableValue> mapper, @Nonnull String defaultValue) {
MappingBindings.mapString(observable, mapper, defaultValue)
}
@Nonnull
static StringBinding mapString(
@Nonnull ObservableValue observable,
@Nonnull ObservableValue> mapper, @Nonnull Supplier supplier) {
MappingBindings.mapString(observable, mapper, supplier)
}
@Nonnull
static BooleanBinding mapBooleans(
@Nonnull ObservableValue observable1,
@Nonnull ObservableValue observable2,
@Nonnull Boolean defaultValue, @Nonnull BiFunction mapper) {
MappingBindings.mapBooleans(observable1, observable2, defaultValue, mapper)
}
@Nonnull
static BooleanBinding mapBooleans(
@Nonnull ObservableValue observable1,
@Nonnull ObservableValue observable2,
@Nonnull Supplier supplier, @Nonnull BiFunction mapper) {
MappingBindings.mapBooleans(observable1, observable2, supplier, mapper)
}
@Nonnull
static BooleanBinding mapBooleans(
@Nonnull ObservableValue observable1,
@Nonnull ObservableValue observable2,
@Nonnull Boolean defaultValue, @Nonnull ObservableValue> mapper) {
MappingBindings.mapBooleans(observable1, observable2, defaultValue, mapper)
}
@Nonnull
static BooleanBinding mapBooleans(
@Nonnull ObservableValue observable1,
@Nonnull ObservableValue observable2,
@Nonnull Supplier supplier, @Nonnull ObservableValue> mapper) {
MappingBindings.mapBooleans(observable1, observable2, supplier, mapper)
}
@Nonnull
static IntegerBinding mapIntegers(
@Nonnull ObservableValue extends Number> observable1,
@Nonnull ObservableValue extends Number> observable2,
@Nonnull Integer defaultValue, @Nonnull BiFunction super Number, ? super Number, Integer> mapper) {
MappingBindings.mapIntegers(observable1, observable2, defaultValue, mapper)
}
@Nonnull
static IntegerBinding mapIntegers(
@Nonnull ObservableValue extends Number> observable1,
@Nonnull ObservableValue extends Number> observable2,
@Nonnull Supplier supplier, @Nonnull BiFunction super Number, ? super Number, Integer> mapper) {
MappingBindings.mapIntegers(observable1, observable2, supplier, mapper)
}
@Nonnull
static IntegerBinding mapIntegers(
@Nonnull ObservableValue extends Number> observable1,
@Nonnull ObservableValue extends Number> observable2,
@Nonnull Integer defaultValue,
@Nonnull ObservableValue> mapper) {
MappingBindings.mapIntegers(observable1, observable2, defaultValue, mapper)
}
@Nonnull
static IntegerBinding mapIntegers(
@Nonnull ObservableValue extends Number> observable1,
@Nonnull ObservableValue extends Number> observable2,
@Nonnull Supplier supplier,
@Nonnull ObservableValue> mapper) {
MappingBindings.mapIntegers(observable1, observable2, supplier, mapper)
}
@Nonnull
static LongBinding mapLongs(
@Nonnull ObservableValue extends Number> observable1,
@Nonnull ObservableValue extends Number> observable2,
@Nonnull Long defaultValue, @Nonnull BiFunction super Number, ? super Number, Long> mapper) {
MappingBindings.mapLongs(observable1, observable2, defaultValue, mapper)
}
@Nonnull
static LongBinding mapLongs(
@Nonnull ObservableValue extends Number> observable1,
@Nonnull ObservableValue extends Number> observable2,
@Nonnull Supplier supplier, @Nonnull BiFunction super Number, ? super Number, Long> mapper) {
MappingBindings.mapLongs(observable1, observable2, supplier, mapper)
}
@Nonnull
static LongBinding mapLongs(
@Nonnull ObservableValue extends Number> observable1,
@Nonnull ObservableValue extends Number> observable2,
@Nonnull Long defaultValue, @Nonnull ObservableValue> mapper) {
MappingBindings.mapLongs(observable1, observable2, defaultValue, mapper)
}
@Nonnull
static LongBinding mapLongs(
@Nonnull ObservableValue extends Number> observable1,
@Nonnull ObservableValue extends Number> observable2,
@Nonnull Supplier supplier,
@Nonnull ObservableValue> mapper) {
MappingBindings.mapLongs(observable1, observable2, supplier, mapper)
}
@Nonnull
static FloatBinding mapFloats(
@Nonnull ObservableValue extends Number> observable1,
@Nonnull ObservableValue extends Number> observable2,
@Nonnull Float defaultValue, @Nonnull BiFunction super Number, ? super Number, Float> mapper) {
MappingBindings.mapFloats(observable1, observable2, defaultValue, mapper)
}
@Nonnull
static FloatBinding mapFloats(
@Nonnull ObservableValue extends Number> observable1,
@Nonnull ObservableValue extends Number> observable2,
@Nonnull Supplier supplier, @Nonnull BiFunction super Number, ? super Number, Float> mapper) {
MappingBindings.mapFloats(observable1, observable2, supplier, mapper)
}
@Nonnull
static FloatBinding mapFloats(
@Nonnull ObservableValue extends Number> observable1,
@Nonnull ObservableValue extends Number> observable2,
@Nonnull Float defaultValue,
@Nonnull ObservableValue> mapper) {
MappingBindings.mapFloats(observable1, observable2, defaultValue, mapper)
}
@Nonnull
static FloatBinding mapFloats(
@Nonnull ObservableValue extends Number> observable1,
@Nonnull ObservableValue extends Number> observable2,
@Nonnull Supplier supplier,
@Nonnull ObservableValue> mapper) {
MappingBindings.mapFloats(observable1, observable2, supplier, mapper)
}
@Nonnull
static DoubleBinding mapDoubles(
@Nonnull ObservableValue extends Number> observable1,
@Nonnull ObservableValue extends Number> observable2,
@Nonnull Double defaultValue, @Nonnull BiFunction super Number, ? super Number, Double> mapper) {
MappingBindings.mapDoubles(observable1, observable2, defaultValue, mapper)
}
@Nonnull
static DoubleBinding mapDoubles(
@Nonnull ObservableValue extends Number> observable1,
@Nonnull ObservableValue extends Number> observable2,
@Nonnull Supplier supplier, @Nonnull BiFunction super Number, ? super Number, Double> mapper) {
MappingBindings.mapDoubles(observable1, observable2, supplier, mapper)
}
@Nonnull
static DoubleBinding mapDoubles(
@Nonnull ObservableValue extends Number> observable1,
@Nonnull ObservableValue extends Number> observable2,
@Nonnull Double defaultValue,
@Nonnull ObservableValue> mapper) {
MappingBindings.mapDoubles(observable1, observable2, defaultValue, mapper)
}
@Nonnull
static DoubleBinding mapDoubles(
@Nonnull ObservableValue extends Number> observable1,
@Nonnull ObservableValue extends Number> observable2,
@Nonnull Supplier supplier,
@Nonnull ObservableValue> mapper) {
MappingBindings.mapDoubles(observable1, observable2, supplier, mapper)
}
@Nonnull
static ObjectBinding mapObjects(
@Nonnull ObservableValue observable1,
@Nonnull ObservableValue observable2,
@Nullable R defaultValue, @Nonnull BiFunction super A, ? super B, R> mapper) {
MappingBindings.mapObjects(observable1, observable2, defaultValue, mapper)
}
@Nonnull
static ObjectBinding mapObjects(
@Nonnull ObservableValue observable1,
@Nonnull ObservableValue observable2,
@Nonnull Supplier supplier, @Nonnull BiFunction super A, ? super B, R> mapper) {
MappingBindings.mapObjects(observable1, observable2, supplier, mapper)
}
@Nonnull
static ObjectBinding mapObjects(
@Nonnull ObservableValue observable1,
@Nonnull ObservableValue observable2,
@Nullable R defaultValue, @Nonnull ObservableValue> mapper) {
MappingBindings.mapObjects(observable1, observable2, defaultValue, mapper)
}
@Nonnull
static ObjectBinding mapObjects(
@Nonnull ObservableValue observable1,
@Nonnull ObservableValue observable2,
@Nonnull Supplier supplier, @Nonnull ObservableValue> mapper) {
MappingBindings.mapObjects(observable1, observable2, supplier, mapper)
}
@Nonnull
static StringBinding mapStrings(
@Nonnull ObservableValue observable1,
@Nonnull ObservableValue observable2,
@Nullable String defaultValue, @Nonnull BiFunction mapper) {
MappingBindings.mapStrings(observable1, observable2, defaultValue, mapper)
}
@Nonnull
static StringBinding mapStrings(
@Nonnull ObservableValue observable1,
@Nonnull ObservableValue observable2,
@Nonnull Supplier supplier, @Nonnull BiFunction mapper) {
MappingBindings.mapStrings(observable1, observable2, supplier, mapper)
}
@Nonnull
static StringBinding mapStrings(
@Nonnull ObservableValue observable1,
@Nonnull ObservableValue observable2,
@Nullable String defaultValue, @Nonnull ObservableValue> mapper) {
MappingBindings.mapStrings(observable1, observable2, defaultValue, mapper)
}
@Nonnull
static StringBinding mapStrings(
@Nonnull ObservableValue observable1,
@Nonnull ObservableValue observable2,
@Nonnull Supplier supplier, @Nonnull ObservableValue> mapper) {
MappingBindings.mapStrings(observable1, observable2, supplier, mapper)
}
@Nonnull
static IntegerBinding mapInteger(@Nonnull ObservableValue extends Number> observable) {
MappingBindings.mapInteger(observable)
}
@Nonnull
static IntegerBinding mapInteger(
@Nonnull ObservableValue extends Number> observable, @Nullable Integer defaultValue) {
MappingBindings.mapInteger(observable, defaultValue)
}
@Nonnull
static IntegerBinding mapInteger(
@Nonnull ObservableValue extends Number> observable, @Nullable Supplier supplier) {
MappingBindings.mapInteger(observable, supplier)
}
@Nonnull
static LongBinding mapLong(@Nonnull ObservableValue extends Number> observable) {
MappingBindings.mapLong(observable)
}
@Nonnull
static LongBinding mapLong(@Nonnull ObservableValue extends Number> observable, @Nullable Long defaultValue) {
MappingBindings.mapLong(observable, defaultValue)
}
@Nonnull
static LongBinding mapLong(
@Nonnull ObservableValue extends Number> observable, @Nullable Supplier supplier) {
MappingBindings.mapLong(observable, supplier)
}
@Nonnull
static FloatBinding mapFloat(@Nonnull ObservableValue extends Number> observable) {
MappingBindings.mapFloat(observable)
}
@Nonnull
static FloatBinding mapFloat(@Nonnull ObservableValue extends Number> observable, @Nullable Float defaultValue) {
MappingBindings.mapFloat(observable, defaultValue)
}
@Nonnull
static FloatBinding mapFloat(
@Nonnull ObservableValue extends Number> observable, @Nullable Supplier supplier) {
MappingBindings.mapFloat(observable, supplier)
}
@Nonnull
static DoubleBinding mapDouble(@Nonnull ObservableValue extends Number> observable) {
MappingBindings.mapDouble(observable)
}
@Nonnull
static DoubleBinding mapDouble(
@Nonnull ObservableValue extends Number> observable, @Nullable Double defaultValue) {
MappingBindings.mapDouble(observable, defaultValue)
}
@Nonnull
static DoubleBinding mapDouble(
@Nonnull ObservableValue extends Number> observable, @Nullable Supplier supplier) {
MappingBindings.mapDouble(observable, supplier)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy