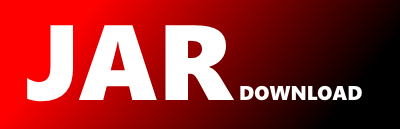
griffon.javafx.collections.GriffonFXCollections Maven / Gradle / Ivy
/*
* Copyright 2008-2017 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package griffon.javafx.collections;
import griffon.javafx.beans.binding.UIThreadAware;
import javafx.application.Platform;
import javafx.collections.ListChangeListener;
import javafx.collections.MapChangeListener;
import javafx.collections.ObservableList;
import javafx.collections.ObservableMap;
import javafx.collections.ObservableSet;
import javafx.collections.SetChangeListener;
import javax.annotation.Nonnull;
import static java.util.Objects.requireNonNull;
/**
* @author Andres Almiray
* @since 2.10.0
*/
public final class GriffonFXCollections {
private static final String ERROR_SOURCE_NULL = "Argument 'source' must not be null";
private GriffonFXCollections() {
// prevent instantiation
}
/**
* Wraps an ObservableList, publishing updates inside the UI thread.
*
* @param source the ObservableList to be wrapped
* @param the list's parameter type.
*
* @return a new ObservableList
*/
@Nonnull
public static ObservableList uiThreadAwareObservableList(@Nonnull ObservableList source) {
requireNonNull(source, ERROR_SOURCE_NULL);
return source instanceof UIThreadAware ? source : new UIThreadAwareObservableList<>(source);
}
private static class UIThreadAwareObservableList extends DelegatingObservableList implements UIThreadAware {
protected UIThreadAwareObservableList(ObservableList delegate) {
super(delegate);
}
@Override
protected void sourceChanged(@Nonnull final ListChangeListener.Change extends E> c) {
if (Platform.isFxApplicationThread()) {
fireChange(c);
} else {
Platform.runLater(() -> fireChange(c));
}
}
}
/**
* Wraps an ObservableSet, publishing updates inside the UI thread.
*
* @param source the ObservableSet to be wrapped
* @param the set's parameter type.
*
* @return a new ObservableSet
*/
@Nonnull
public static ObservableSet uiThreadAwareObservableSet(@Nonnull ObservableSet source) {
requireNonNull(source, ERROR_SOURCE_NULL);
return source instanceof UIThreadAware ? source : new UIThreadAwareObservableSet<>(source);
}
private static class UIThreadAwareObservableSet extends DelegatingObservableSet implements UIThreadAware {
protected UIThreadAwareObservableSet(ObservableSet delegate) {
super(delegate);
}
@Override
protected void sourceChanged(@Nonnull final SetChangeListener.Change extends E> c) {
if (Platform.isFxApplicationThread()) {
fireChange(c);
} else {
Platform.runLater(() -> fireChange(c));
}
}
}
/**
* Wraps an ObservableMap, publishing updates inside the UI thread.
*
* @param source the ObservableMap to be wrapped
* @param the type of keys maintained by the map
* @param the type of mapped values
*
* @return a new ObservableMap
*/
@Nonnull
public static ObservableMap uiThreadAwareObservableMap(@Nonnull ObservableMap source) {
requireNonNull(source, ERROR_SOURCE_NULL);
return source instanceof UIThreadAware ? source : new UIThreadAwareObservableMap<>(source);
}
private static class UIThreadAwareObservableMap extends DelegatingObservableMap implements UIThreadAware {
protected UIThreadAwareObservableMap(ObservableMap delegate) {
super(delegate);
}
@Override
protected void sourceChanged(@Nonnull final MapChangeListener.Change extends K, ? extends V> c) {
if (Platform.isFxApplicationThread()) {
fireChange(c);
} else {
Platform.runLater(() -> fireChange(c));
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy