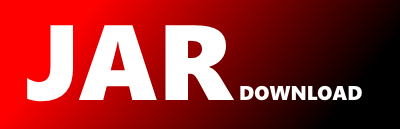
org.codehaus.jackson.map.ser.WritableBeanProperty Maven / Gradle / Ivy
Go to download
Data Mapper package is a high-performance data binding package
built on Jackson JSON processor
package org.codehaus.jackson.map.ser;
import java.io.IOException;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Method;
import org.codehaus.jackson.JsonGenerationException;
import org.codehaus.jackson.JsonGenerator;
import org.codehaus.jackson.map.JsonSerializer;
import org.codehaus.jackson.map.SerializerProvider;
/**
* Simple container class, used to store information about a single
* property, matching to a single accessor method, and to be serializer
* as a field value in json output.
*/
public final class WritableBeanProperty
{
/**
* Logical name of the property; will be used as the field name
* under which value for the property is written.
*/
final String _name;
/**
* Accessor method used to get property value
*/
final Method _accessorMethod;
/**
* Serializer to use for writing out the value; if known statically.
* Will be null when property's static type is non-final, since
* then the dynamic type may be different.
*/
JsonSerializer
© 2015 - 2025 Weber Informatics LLC | Privacy Policy