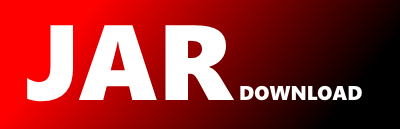
org.codehaus.jackson.map.SerializerProvider Maven / Gradle / Ivy
package org.codehaus.jackson.map;
import java.io.IOException;
import java.util.Date;
import org.codehaus.jackson.*;
import org.codehaus.jackson.schema.JsonSchema;
/**
* Abstract class that defines API used by {@link ObjectMapper} and
* {@link JsonSerializer}s to obtain serializers capable of serializing
* instances of specific types.
*
* Note about usage: for {@link JsonSerializer} instances, only accessors
* for locating other (sub-)serializers are to be used. {@link ObjectMapper},
* on the other hand, is to initialize recursive serialization process by
* calling {@link #serializeValue}.
*/
public abstract class SerializerProvider
{
protected final SerializationConfig _config;
protected SerializerProvider(SerializationConfig config)
{
_config = config;
}
/*
//////////////////////////////////////////////////////
// Methods that ObjectMapper will call
//////////////////////////////////////////////////////
*/
/**
* The method to be called by {@link ObjectMapper} to
* execute recursive serialization, using serializers that
* this provider has access to.
*
* @param jsf Underlying factory object used for creating serializers
* as needed
*/
public abstract void serializeValue(SerializationConfig cfg,
JsonGenerator jgen, Object value,
SerializerFactory jsf)
throws IOException, JsonGenerationException;
/**
* Generate the Json-schema.
*
* @param type The type.
* @param config The config.
* @param jsf The serializer factory.
* @return The config.
*/
public JsonSchema generateJsonSchema(Class> type, SerializationConfig config, SerializerFactory jsf)
throws JsonMappingException {
throw new UnsupportedOperationException();
}
/**
* Method that can be called to see if this serializer provider
* can find a serializer for an instance of given class.
*
* Note that no Exceptions are thrown, including unchecked ones:
* implementations are to swallow exceptions if necessary.
*/
public abstract boolean hasSerializerFor(SerializationConfig cfg,
Class> cls, SerializerFactory jsf);
/*
//////////////////////////////////////////////////////
// Access to configuration
//////////////////////////////////////////////////////
*/
public final SerializationConfig getConfig() { return _config; }
public final boolean isEnabled(SerializationConfig.Feature f) {
return _config.isEnabled(f);
}
/*
//////////////////////////////////////////////////////
// General serializer locating method
//////////////////////////////////////////////////////
*/
/**
* Method called to get hold of a serializer for a value of given type;
* or if no such serializer can be found, a default handler (which
* may do a best-effort generic serialization or just simply
* throw an exception when invoked).
*
* Note: this method is only called for non-null values; not for keys
* or null values. For these, check out other accessor methods.
*
* @throws JsonMappingException if there are fatal problems with
* accessing suitable serializer; including that of not
* finding any serializer
*/
public abstract JsonSerializer