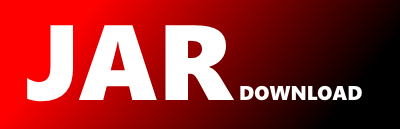
org.codehaus.jackson.map.deser.MapDeserializer Maven / Gradle / Ivy
package org.codehaus.jackson.map.deser;
import java.io.IOException;
import java.util.*;
import org.codehaus.jackson.JsonProcessingException;
import org.codehaus.jackson.JsonParser;
import org.codehaus.jackson.JsonToken;
import org.codehaus.jackson.map.JsonDeserializer;
import org.codehaus.jackson.map.DeserializationContext;
import org.codehaus.jackson.map.KeyDeserializer;
/**
* Basic serializer that can take Json "Object" structure and
* construct a {@link java.util.Map} instance, with typed contents.
*
* Note: for untyped content (one indicated by passing Object.class
* as the type), {@link UntypedObjectDeserializer} is used instead.
* It can also construct {@link java.util.Map}s, but not with specific
* POJO types, only other containers and primitives/wrappers.
*/
public class MapDeserializer
extends StdDeserializer
© 2015 - 2025 Weber Informatics LLC | Privacy Policy