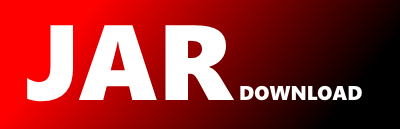
org.codehaus.jackson.map.deser.CollectionDeserializer Maven / Gradle / Ivy
Go to download
Data Mapper package is a high-performance data binding package
built on Jackson JSON processor
package org.codehaus.jackson.map.deser;
import java.io.IOException;
import java.util.*;
import org.codehaus.jackson.JsonProcessingException;
import org.codehaus.jackson.JsonParser;
import org.codehaus.jackson.JsonToken;
import org.codehaus.jackson.map.JsonDeserializer;
import org.codehaus.jackson.map.DeserializationContext;
/**
* Basic serializer that can take Json "Array" structure and
* construct a {@link java.util.Collection} instance, with typed contents.
*
* Note: for untyped content (one indicated by passing Object.class
* as the type), {@link UntypedObjectDeserializer} is used instead.
* It can also construct {@link java.util.List}s, but not with specific
* POJO types, only other containers and primitives/wrappers.
*/
public class CollectionDeserializer
extends StdDeserializer>
{
// // Configuration
final Class> _collectionClass;
/**
* Value deserializer.
*/
final JsonDeserializer
© 2015 - 2025 Weber Informatics LLC | Privacy Policy