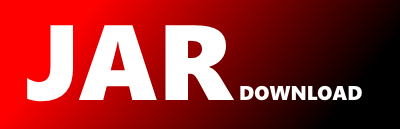
org.codehaus.jackson.map.introspect.AnnotatedConstructor Maven / Gradle / Ivy
Go to download
Data Mapper package is a high-performance data binding package
built on Jackson JSON processor
package org.codehaus.jackson.map.introspect;
import java.lang.annotation.Annotation;
import java.lang.reflect.Constructor;
import java.lang.reflect.Type;
import org.codehaus.jackson.map.util.ClassUtil;
public final class AnnotatedConstructor
extends Annotated
{
final Constructor> _constructor;
final AnnotationMap _classAnnotations;
final AnnotationMap[] _paramAnnotations;
/*
//////////////////////////////////////////////////////
// Life-cycle
//////////////////////////////////////////////////////
*/
public AnnotatedConstructor(Constructor> constructor, AnnotationMap annMap,
AnnotationMap[] paramAnnotations)
{
_constructor = constructor;
_classAnnotations = annMap;
_paramAnnotations = paramAnnotations;
}
/**
* Method called to override a constructor annotation, usually due to a mix-in
* annotation masking or overriding an annotation 'real' constructor
* has.
*/
public void addOrOverride(Annotation a)
{
_classAnnotations.add(a);
}
/**
* Method called to override a constructor parameter annotation,
* usually due to a mix-in
* annotation masking or overriding an annotation 'real' constructor
* has.
*/
public void addOrOverrideParam(int paramIndex, Annotation a)
{
AnnotationMap old = _paramAnnotations[paramIndex];
if (old == null) {
old = new AnnotationMap();
_paramAnnotations[paramIndex] = old;
}
old.add(a);
}
/*
//////////////////////////////////////////////////////
// Annotated impl
//////////////////////////////////////////////////////
*/
public Constructor> getAnnotated() { return _constructor; }
public int getModifiers() { return _constructor.getModifiers(); }
public String getName() { return _constructor.getName(); }
public A getAnnotation(Class acls)
{
return _classAnnotations.get(acls);
}
public Class> getType() {
return _constructor.getDeclaringClass();
}
/*
//////////////////////////////////////////////////////
// Extended API
//////////////////////////////////////////////////////
*/
public AnnotatedParameter getParameter(int index) {
return new AnnotatedParameter(getParameterType(index), _paramAnnotations[index]);
}
public int getParameterCount() {
return _constructor.getParameterTypes().length;
}
public Class> getParameterClass(int index)
{
Class>[] types = _constructor.getParameterTypes();
return (index >= types.length) ? null : types[index];
}
public Type getParameterType(int index)
{
Type[] types = _constructor.getGenericParameterTypes();
return (index >= types.length) ? null : types[index];
}
public AnnotationMap getParameterAnnotations(int index)
{
if (_paramAnnotations != null) {
if (index >= 0 && index <= _paramAnnotations.length) {
return _paramAnnotations[index];
}
}
return null;
}
/**
* Method that can be called to modify access rights, by calling
* {@link java.lang.reflect.AccessibleObject#setAccessible} on
* the underlying annotated element.
*/
public void fixAccess()
{
ClassUtil.checkAndFixAccess(_constructor);
}
/*
//////////////////////////////////////////////////////
// Extended API, specific annotations
//////////////////////////////////////////////////////
*/
public String toString()
{
return "[constructor for "+getName()+", annotations: "+_classAnnotations+"]";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy