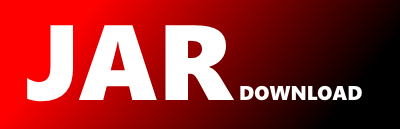
jedi.either.Either Maven / Gradle / Ivy
The newest version!
package jedi.either;
import java.io.Serializable;
import java.util.List;
import jedi.functional.Command;
import jedi.functional.Functor;
import jedi.functional.Functor0;
/**
* The Either
type represents a value of one of two possible types
* (a disjoint union) and was Inspired by Scala's Either.
*/
public abstract class Either implements Serializable {
private static final long serialVersionUID = 1L;
/**
* If the condition satisfies, return the given A in Left
,
* otherwise, return the given B in Right
.
*/
public static Either cond(boolean cond, A a, B b) {
return cond ? new Left(a) : new Right(b);
}
/**
* If the condition satisfies, return the result of executing a in Left
,
* otherwise, return the result of executing b in Right
.
*/
public static Either cond(boolean cond, Functor0 a, Functor0 b) {
return cond ? new Left(a.execute()) : new Right(b.execute());
}
public boolean isLeft() {
return false;
}
public boolean isRight() {
return false;
}
public LeftProjection left() {
return new LeftProjection(this);
}
public RightProjection right() {
return new RightProjection(this);
}
/**
* Deconstruction of the type.
*
* @param fa
* the functor to apply if this is a Left
* @param fb
* the functor to apply if this is a Left
* @return the result of applying the appropriate functor.
*/
public abstract X fold(Functor super A, X> fa, Functor super B, X> fb);
public abstract void execute(Command ca, Command cb);
public abstract Either swap();
/**
* @return Singleton list if Lift or empty list if Right.
*/
public abstract List asList();
/**
* Maps the function argument through Left
.
*/
public abstract Either map(Functor super A, X> f);
public abstract Either flatMap(Functor super A, Either> f);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy