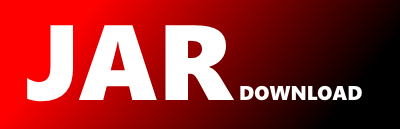
jedi.either.Left Maven / Gradle / Ivy
The newest version!
package jedi.either;
import jedi.functional.Command;
import jedi.functional.Functor;
import java.util.List;
import static java.util.Collections.singletonList;
/**
* Left typically represents a failure condition of Either - but not
* necessarily.
*/
public final class Left extends Either {
public static final long serialVersionUID = 1L;
private final A a;
public Left(A a) {
this.a = a;
}
@Override
public boolean isLeft() {
return true;
}
@Override
public X fold(Functor super A, X> fa, Functor super B, X> fb) {
return fa.execute(a);
}
@Override
public void execute(Command ca, Command cb) {
ca.execute(a);
}
@Override
public Either swap() {
return new Right(a);
}
@Override
public boolean equals(Object obj) {
if (obj == this) {
return true;
}
if (obj == null || !getClass().equals(obj.getClass())) {
return false;
}
Left, ?> other = (Left, ?>) obj;
return a.equals(other.a);
}
@Override
public int hashCode() {
return a.hashCode();
}
@Override
public String toString() {
return "Left:" + a;
}
@Override
public List asList() {
return singletonList(a);
}
@Override
public Either map(Functor super A, X> f) {
return new Left(f.execute(a));
}
@Override
public Either flatMap(Functor super A, Either> f) {
return f.execute(a);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy