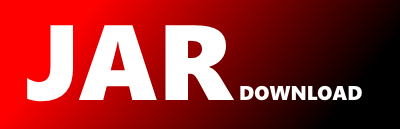
jedi.either.LeftProjection Maven / Gradle / Ivy
The newest version!
package jedi.either;
import jedi.functional.*;
import jedi.option.Option;
import jedi.option.Options;
import java.util.Iterator;
import java.util.NoSuchElementException;
/**
* Projects an Either
into a Left
.
*
*/
public final class LeftProjection implements Iterable {
private final Either either;
public LeftProjection(Either either) {
this.either = either;
}
/**
* Returns the value from this Left
or throws
* NoSuchElementException
if this is a
* Right
.
*/
public A get() {
return either.fold(new Functor() {
public A execute(A value) {
return value;
}
}, new Functor() {
public A execute(B value) {
throw new NoSuchElementException();
}
});
}
private B getRight() {
return either.fold(new Functor() {
public B execute(A value) {
throw new NoSuchElementException();
}
}, new Functor() {
public B execute(B value) {
return value;
}
});
}
/**
* Executes the given side-effect if this is a Left
.
*
* @param c
* The side-effect to execute.
*/
public void forEach(final Command c) {
either.execute(new Command() {
public void execute(A value) {
c.execute(value);
}
}, new NullCommand());
}
/**
* @deprecated please use {@link #forEach(jedi.functional.Command)}
* @see #forEach(jedi.functional.Command)
*/
public void foreach(final Command c) {
forEach(c);
}
/**
* Returns the value from this Left
or the given argument if
* this is a Right
.
*/
public A getOrElse(T or) {
return either.isLeft() ? get() : or;
}
/**
* @deprecated please use {@link #forAll(Functor)}
* @see #forAll(Functor)
*/
public Boolean forall(Functor super A, Boolean> f) {
return forAll(f);
}
/**
* Returns true
if Right
or returns the result of
* the application of the given function to the Left
value.
*/
public Boolean forAll(Functor super A, Boolean> f) {
return either.isLeft() ? f.execute(get()) : true;
}
/**
* Returns false
if Right
or returns the result of
* the application of the given function to the Left
value.
*/
public Boolean exists(Functor f) {
return either.isLeft() ? f.execute(get()) : false;
}
/**
* Binds the given function across Left
.
*
* @param f The function to bind across Left
.
*/
public Either flatMap(Functor super A, Either> f) {
return either.isLeft() ? f.execute(get()) : new Right(getRight());
}
/**
* Maps the function argument through Left
.
*/
public Either map(Functor super A, X> f) {
return either.isLeft() ? new Left(f.execute(get())) : new Right(getRight());
}
/**
* Returns None
if this is a Right
or if the given
* predicate p
does not hold for the left value, otherwise,
* returns a Left
.
*/
public Option extends Either> filter(Functor super A, Boolean> p) {
if (either.isLeft() && p.execute(get())) {
return Options.some(new Left(get()));
} else {
return Options.none();
}
}
/**
* Returns a Some
containing the Left
value if it
* exists or a None
if this is a Right
.
*/
public Option toOption() {
if (either.isLeft()) {
return Options.some(get());
} else {
return Options.none();
}
}
@SuppressWarnings("unchecked")
public Iterator iterator() {
if (either.isLeft()) {
return Coercions.list(get()).iterator();
} else {
return new EmptyIterator();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy