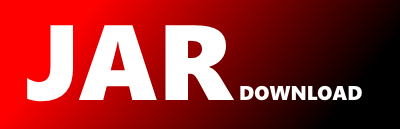
jedi.functional.Coercions Maven / Gradle / Ivy
The newest version!
package jedi.functional;
import static jedi.assertion.Assert.assertEqual;
import static jedi.assertion.Assert.assertNotNull;
import static jedi.assertion.Assert.assertTrue;
import static jedi.functional.FunctionalPrimitives.head;
import static jedi.functional.FunctionalPrimitives.select;
import java.lang.reflect.Array;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Set;
import jedi.filters.NotNullFilter;
import jedi.tuple.Tuple2;
public class Coercions {
/**
* Create an array from the given parameter list
*/
public static T[] array(final T... items) {
assertNotNull(items, "items must not be null");
return items;
}
/**
* Produce an array from a collection. All of the items
must be
* of the same class, otherwise an {@link ArrayStoreException} will be
* thrown. If you want to specify the type use {@link #asArray(Class, Collection)}.
*/
@SuppressWarnings("unchecked")
public static T[] asArray(final Collection items) {
assertNotNull(items, "items must not be null");
return items.toArray((T[]) Array.newInstance(head(select(items, new NotNullFilter())).getClass(), items.size()));
}
/**
* Produce an array from a collection.
* @param clazz the type you want the array to be
* @param items to convert to array
* @return an array
*/
@SuppressWarnings("unchecked")
public static T[] asArray(Class clazz, Collection items) {
assertNotNull(items, "items must not be null");
return items.toArray((T[]) Array.newInstance(clazz, items.size()));
}
/**
* Create a {@link jedi.functional.Filter Filter} which uses the given
* functor
to map from one domain (T) to another (R) and then
* applies the given filter
to R. i.e. the result of
* executing
the returned filter with a value t
is
* equivalent to filter.execute(functor.execute(t))
.
*/
public static Filter asFilter(final Functor functor, final Filter super R> filter) {
assertNotNull(functor, "functor must not be null");
assertNotNull(filter, "filter must not be null");
return new Filter() {
public Boolean execute(final T t) {
return filter.execute(functor.execute(t));
}
};
}
/**
* Create a {@link jedi.functional.Functor Functor} which uses the given
* map
as the transform between argument (key) and result
* (value). The returned functor can optionally deal with an argument which
* does not exist as a key in the map
by (a) throwing an
* {@link jedi.assertion.AssertionError AssertionError} or (b) returning
* null
.
*/
public static Functor asFunctor(final Map map, final boolean allowUncontainedKeys) {
assertNotNull(map, "map must not be null");
return new Functor() {
public R execute(final T key) {
assertTrue(allowUncontainedKeys || map.containsKey(key), "Unknown key", key);
return map.get(key);
}
};
}
/**
* Copy the given collection into a new list
*/
public static List asList(final Iterable items) {
assertNotNull(items, "items must not be null");
ArrayList list = new ArrayList();
for (T t : items) {
list.add(t);
}
return list;
}
/**
* Copy the elements in the given array to a new list
*/
public static List asList(final T[] items) {
assertNotNull(items, "items");
return new ArrayList(Arrays.asList(items));
}
public static Map asMap(final Tuple2[] tuples) {
assertNotNull(tuples, "tuples must not be null");
return asMap(asList(tuples));
}
/**
* Make a Map from the given tuples using the {@link Tuple2#a()} values as keys
and
* the {@link Tuple2#b()} values as values
.
*/
public static Map map(final Tuple2... tuples) {
return asMap(tuples);
}
/**
* Create a Map from the given tuples using the {@link Tuple2#a()} values as keys
and
* the {@link Tuple2#b()} values as values
.
*/
public static Map asMap(final Iterable> tuples) {
assertNotNull(tuples, "tuples must not be null");
final Map map = new HashMap();
for (Tuple2 tuple : tuples) {
map.put(tuple.a(), tuple.b());
}
return map;
}
/**
* Create a Map from the given keys
and the given
* values
.
*/
public static Map asMap(final Iterable keys, final Iterable values) {
assertNotNull(keys, "keys must not be null");
assertNotNull(values, "values must not be null");
final Map map = new HashMap();
final Iterator valueIterator = values.iterator();
final Iterator keyIterator = keys.iterator();
while (keyIterator.hasNext() && valueIterator.hasNext()) {
map.put(keyIterator.next(), valueIterator.next());
}
assertTrue(!keyIterator.hasNext() && !valueIterator.hasNext(), "keys and vaues must be the same size");
return map;
}
/**
* Create a map whose values are the given items
each of which
* is keyed on the result of applying the given keyFunctor
to
* the item.
*/
public static Map asMap(final Iterable items, final Functor super T, K> keyFunctor) {
assertNotNull(items, "items must not be null");
assertNotNull(keyFunctor, "keyFunctor must not be null");
final Map map = new HashMap();
int count = 0;
for (final T item : items) {
map.put(keyFunctor.execute(item), item);
count++;
}
assertEqual(count, map.size(), "items and map should be the same size but are not");
return map;
}
/**
* Copy the given collection into a new set
*/
public static Set asSet(final Collection items) {
assertNotNull(items, "items must not be null");
return new HashSet(items);
}
/**
* Copy the elements in the given array to a new set
*/
public static Set asSet(final T[] items) {
return new HashSet(asList(items));
}
/**
* Produce a collection with a different instantiated generic type from a
* given collection.
*/
public static List cast(final Class returnType, final Iterable items) {
assertNotNull(returnType, "returnType must not be null");
return FunctionalPrimitives.collect(items, new Functor() {
@SuppressWarnings("unchecked")
public R execute(final T value) {
return (R) value;
}
});
}
/**
* Make a list from the given parameters
*/
public static List list(final T... items) {
return asList(items);
}
/**
* Make a set from the given items
*/
public static Set set(final T... items) {
return asSet(items);
}
private Coercions() {
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy