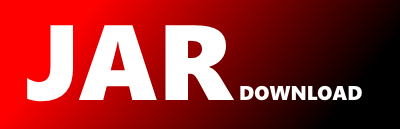
jedi.functional.Comparables Maven / Gradle / Ivy
The newest version!
package jedi.functional;
import static jedi.assertion.Assert.assertNotNull;
import static jedi.functional.Coercions.asList;
import static jedi.functional.FunctionalPrimitives.fold;
import static jedi.functional.FunctionalPrimitives.head;
import java.util.Collection;
import java.util.Collections;
import java.util.Comparator;
import java.util.List;
import jedi.functors.MaxFunctor;
import jedi.functors.MinFunctor;
public class Comparables {
public static Filter equal(final T item, final Comparator comparator) {
return new Filter() {
public Boolean execute(final T value) {
return comparator.compare(value, item) == 0;
}
};
}
@SuppressWarnings("unchecked")
public static Filter greaterThan(final T item, final Comparator comparator) {
return FirstOrderLogic.not(FirstOrderLogic.or(Comparables.lessThan(item, comparator), Comparables.equal(item, comparator)));
}
public static Filter lessThan(final T item, final Comparator comparator) {
return new Filter() {
public Boolean execute(final T value) {
return comparator.compare(value, item) < 0;
}
};
}
/**
* Find and return the maximum value from a collection of comparables.
* Requires at least one item in the collection.
*/
public static > T max(final Collection items) {
return fold(head(items), items, new MaxFunctor());
}
/**
* Find and return the maximum value from a collection of comparables, or a
* default value if the collection is empty.
*/
public static > T max(final T defaultValue, final Collection items) {
assertNotNull(items, "items must not be null");
return items.isEmpty() ? defaultValue : Comparables.max(items);
}
/**
* Find and return the minimum value from a collection of comparables.
* Requires at least one item in the collection.
*/
public static > T min(final Collection items) {
return fold(head(items), items, new MinFunctor());
}
/**
* Find and return the minimum value from a collection of comparables, or a
* default value if the collection is empty.
*/
public static > T min(final T defaultValue, final Collection items) {
assertNotNull(items, "items must not be null");
return items.isEmpty() ? defaultValue : Comparables.min(items);
}
/**
* Copy the items
into a new list, sort the new list using the
* natural ordering of the contained items, and return the new list.
*/
public static > List sort(final Collection items) {
return sortInPlace(asList(items));
}
/**
* Copy the items
into a new list, sort the new list using the
* given comparator
and return the new list.
*/
public static List sort(final Collection items, final Comparator super T> comparator) {
return sortInPlace(asList(items), comparator);
}
/**
* Copy the items
into a new list, sort the new list using the
* {@link java.lang.Comparable Comparable} values obtained by applying the
* given evaluator
to each item and return the new list.
*/
public static > List sort(final Collection items, final Functor super T, C> evaluator) {
return sortInPlace(asList(items), evaluator);
}
/**
* Sort items
in place using the natural ordering of the
* contained items. Note that this function returns the collection allowing
* functions to be composed easily.
*/
public static > List sortInPlace(final List items) {
assertNotNull(items, "items must not be null");
Collections.sort(items);
return items;
}
/**
* Sort items
in place using the given comparator
.
* Note that this function returns the collection allowing functions to be
* composed easily.
*/
public static List sortInPlace(final List items, final Comparator super T> comparator) {
assertNotNull(items, "items must not be null");
assertNotNull(comparator, "comparator must not be null");
Collections.sort(items, comparator);
return items;
}
/**
* Sort items
in place using the {@link java.lang.Comparable
* Comparable} values obtained by applying the given evaluator
* to each item. Note that this function returns the collection allowing
* functions to be composed easily.
*/
public static > List sortInPlace(final List items, final Functor super T, C> evaluator) {
assertNotNull(items, "items must not be null");
assertNotNull(evaluator, "evaluator must not be null");
return sortInPlace(items, new Comparator() {
public int compare(final T t1, final T t2) {
return evaluate(t1).compareTo(evaluate(t2));
}
private C evaluate(final T t) {
return evaluator.execute(t);
}
});
}
private Comparables() {
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy