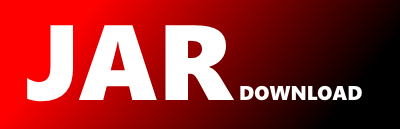
jedi.functional.FirstOrderLogic Maven / Gradle / Ivy
The newest version!
package jedi.functional;
import static jedi.assertion.Assert.assertNotNull;
import static jedi.functional.Coercions.asList;
import static jedi.functional.Coercions.asSet;
import static jedi.functional.FunctionalPrimitives.hasItems;
import static jedi.functional.FunctionalPrimitives.head;
import static jedi.functional.FunctionalPrimitives.select;
import static jedi.functional.FunctionalPrimitives.tail;
import java.util.Collection;
import java.util.HashSet;
import java.util.Set;
import jedi.filters.Conjunction;
import jedi.filters.Disjunction;
import jedi.filters.Inverter;
public class FirstOrderLogic {
/**
* Returns true
if a given predicate
returns
* true
for at least one of the items
in a given
* iterable. false
otherwise.
*/
public static boolean exists(Iterable items, Filter super T> predicate) {
assertNotNull(items, "items must not be null");
assertNotNull(predicate, "predicate must not be null");
for (T item : items) {
if (predicate.execute(item)) {
return true;
}
}
return false;
}
/**
* Returns true
if a given predicate
returns
* true
for all of the items
in a given iterable.
* false
otherwise.
*/
public static boolean all(Iterable items, Filter super T> predicate) {
return !exists(items, invert(predicate));
}
/**
* Returns true
if a given predicate
returns
* true
for zero or one of the items
in a given
* iterable. false
otherwise.
*/
public static boolean zeroOrOne(Iterable items, Filter super T> predicate) {
return select(items, predicate).size() <= 1;
}
/**
* Create a filter which is the logical inversion of a given filter
*/
public static Filter invert(final Filter filter) {
return Inverter.create(filter);
}
/**
* Synonymous with {@link #invert(Filter) invert(Filter)}
*/
public static Filter not(Filter filter) {
return invert(filter);
}
/**
* Create a short circuiting filter which is the conjunction of the given
* filters
*/
public static Filter and(final Filter... filters) {
return Conjunction.create(filters);
}
/**
* Create a short circuiting filter which is the disjunction of the given
* filters
*/
public static Filter or(final Filter... filters) {
return Disjunction.create(filters);
}
/**
* Calculate the intersection of all of the given collections.
*/
public static Set intersection(Collection... collections) {
return intersection(asList(collections));
}
/**
* Calculate the intersection of all of the given collections.
*/
public static Set intersection(Iterable> collections) {
assertNotNull(collections, "collections");
if (!hasItems(collections)) {
return new HashSet();
}
Set intersection = asSet(head(collections));
for (Collection collection : tail(collections)) {
intersection.retainAll(collection);
}
return intersection;
}
/**
* Calculate the union of all of the given collections.
*/
public static Set union(Collection... collections) {
return union(asList(collections));
}
/**
* Calculate the union of all of the given collections.
*/
public static Set union(Iterable> collections) {
assertNotNull(collections, "collections");
if (!hasItems(collections)) {
return new HashSet();
}
Set union = asSet(head(collections));
for (Collection collection : tail(collections)) {
union.addAll(collection);
}
return union;
}
/**
* Create a filter which is the exclusive or of the given filters
.
*/
@SuppressWarnings("unchecked")
public static Filter xor(Filter a, Filter b) {
return or(and(a, not(b)), and(not(a), b));
}
private FirstOrderLogic() {
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy