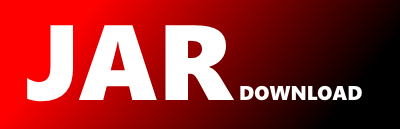
jedi.functors.DynamicFunctor Maven / Gradle / Ivy
The newest version!
package jedi.functors;
import jedi.JediException;
import jedi.functional.Functor;
import jedi.functional.Functor2;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Method;
import java.util.Collections;
import java.util.List;
/**
* This Functor reflectively executes a method on a type with zero or more arguments.
* Examples
*
DynamicFunctor<String, Integer> stringLength = new DynamicFunctor(String.class, "length");
*
stringLength.execute("hello") returns 5
*
*
DynamicFunctor<String, Character> stringCharAt = new DynamicFunctor(String.class, "charAt", Integer.TYPE);
*
stringCharAt.execute("hello", list(3)) returns 'l'
*/
public class DynamicFunctor implements Functor2, R>, Functor {
private final Method method;
public DynamicFunctor(Class clazz, String methodName, Class>... argTypes) {
try {
method = clazz.getMethod(methodName, argTypes);
if (method == null) throw new IllegalArgumentException("Method not found");
} catch (NoSuchMethodException e) {
throw new JediException(e);
}
}
public R execute(T t) {
return execute(t, Collections.
© 2015 - 2025 Weber Informatics LLC | Privacy Policy