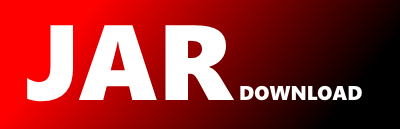
jedi.option.Option Maven / Gradle / Ivy
The newest version!
package jedi.option;
import java.io.Serializable;
import java.util.List;
import jedi.functional.Command;
import jedi.functional.Command0;
import jedi.functional.Filter;
import jedi.functional.Functor;
import jedi.functional.Functor0;
/**
* An optional value inspired by sensible languages like Scala.
*/
public interface Option extends Iterable, Serializable{
/**
* Accept a for this {@link Option}.
* In languages like Scala we can do this kind of thing:
*
* x match {
* case {@link Some}(s) => s
* case {@link None} => "?"
* }
*
*
* In Java, such elegance is not possible so this method is actually a
* Visitor implementation:
*
*
* x.match(new OptionMatcher() {
* public void caseSome(T value) { // excellent we can use the value }
* public void caseNone() { // no value, deal with it }
* });
*
*/
void match(OptionMatcher super T> matcher);
/**
* A match strategy based on Commands. Since annotated methods become
* commands in Jedi, this approach is a very simple solution to dealing with
* {@link Some} and {@link None}.
*
* @param someCommand
* a command that will receive the callback in the event that
* {@link Some} is matched
* @param noneCommand
* a command that will receive the callback in the event that
* {@link None} is matched
*/
void match(Command super T> someCommand, Command0 noneCommand);
/**
* A match strategy based on Functors.
*
* @param someFunctor
* the functor to execute if this Option is a Same
* @param noneFunctor
* the functor to execute if this Option is a {@link None}
* @return the result of executing the functor
*/
R1 match(Functor super T, R1> someFunctor, Functor0 noneFunctor);
/**
* An empty list for {@link None} or an immutable list with
* {@link Some#get}.
*/
List asList();
/**
* If the option is {@link Some} return its value, otherwise return the
* result of evaluating the generator expression.
*
* @param generator
* the default expression.
*/
T getOrElse(Functor0 extends T> generator);
/**
* If the option is {@link Some} return its value, otherwise return a
* default value.
*/
T getOrElse(T defaultValue);
/**
* If the option is {@link Some}, return a function applied to its
* value, wrapped in a {@link Some}: {@link Some}(f(this.get))
, otherwise
* return {@link None}.
*
* @param mappingFunction
* the function to apply
*/
Option map(Functor super T, R> mappingFunction);
Option flatMap(Functor super T, Option> mappingFunction);
/**
* Apply the given Command to the option's value if it is not {@link None}. Do
* nothing if it is {@link None}.
*
* @param command
* the Command to apply.
*/
void forEach(Command super T> command);
/**
* If this option is {@link Some} and the given functor f
* yields false
on its value, return {@link None}.
* Otherwise return this option.
*
* @param f
* the filter used for testing.
*/
Option filter(Filter super T> f);
/**
* Get the value returning null
for None.
*/
T unsafeGet();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy