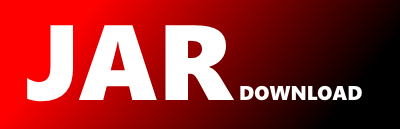
jedi.option.Some Maven / Gradle / Ivy
The newest version!
package jedi.option;
import jedi.functional.*;
import java.util.Iterator;
import java.util.List;
import static java.util.Collections.singletonList;
import static jedi.assertion.Assert.assertNotNull;
import static jedi.option.Options.some;
/**
* Some represents a value of type T
that exists.
*/
public final class Some implements Option {
public static final long serialVersionUID = 1L;
private final T value;
public Some(T value) {
assertNotNull(value, "Some value cannot be null, use None instead");
this.value = value;
}
public List asList() {
return singletonList(get());
}
public T getOrElse(Functor0 extends T> generator) {
return get();
}
public T getOrElse(T defaultValue) {
return get();
}
/**
* Get the value this Some contains.
*
* @return the value, guaranteed to not be null.
*/
public T get() {
return value;
}
public void match(OptionMatcher super T> matcher) {
assertNotNull(matcher, "OptionMatcher must not be null");
matcher.caseSome(value);
}
public void match(Command super T> someCommand, Command0 noneCommand) {
someCommand.execute(get());
}
public R1 match(Functor super T, R1> someFunctor, Functor0 noneFunctor) {
return someFunctor.execute(get());
}
public Option map(Functor super T, R> mappingFunction) {
R result = mappingFunction.execute(get());
return result == null ? Options.none() : some(result);
}
public Option flatMap(Functor super T, Option> mappingFunction) {
return mappingFunction.execute(get());
}
@Override
public boolean equals(Object obj) {
return obj instanceof Some && get().equals(((Some>) obj).get());
}
@Override
public int hashCode() {
return get().hashCode();
}
@Override
public String toString() {
return "Some: " + get();
}
public void forEach(Command super T> command) {
command.execute(get());
}
public Option filter(Filter super T> f) {
return f.execute(get()) ? this : Options. none();
}
public Iterator iterator() {
return asList().iterator();
}
public T unsafeGet() {
return value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy