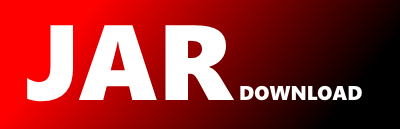
jedi.example.CommandExampleClosureFactory Maven / Gradle / Ivy
package jedi.example;
public class CommandExampleClosureFactory implements ICommandExampleClosureFactory {
/**
* @see jedi.example.CommandExample#doX
*/
public jedi.functional.Command doXCommand(final java.lang.String arg) {
class Closure implements java.io.Serializable, jedi.functional.Command {
public void execute(final jedi.example.CommandExample $receiver) {
try {
$receiver.doX(arg);
}
catch (RuntimeException ex) {
throw ex;
}
catch (Exception ex) {
throw new jedi.JediException(ex);
}
}
public int hashCode() {
return (17) * 37 + (arg == null ? 0 : arg.hashCode());
}
public boolean equals(Object obj) {
if (obj == this) { return true; }
if (!(obj instanceof Closure)) { return false; }
return ((Closure) obj).equalsParameters(arg);
}
private boolean equalsParameters(final java.lang.String $arg) {
return ($arg == null ? arg == null : $arg.equals(arg));
}
}
return new Closure();
}
/**
* @see jedi.example.CommandExample#doX
*/
public jedi.functional.Command doXProxyCommand(final jedi.example.CommandExample $receiver) {
class Closure implements java.io.Serializable, jedi.functional.Command {
public void execute(final java.lang.String arg) {
try {
$receiver.doX(arg);
}
catch (RuntimeException ex) {
throw ex;
}
catch (Exception ex) {
throw new jedi.JediException(ex);
}
}
public int hashCode() {
return (17) * 37 + ($receiver == null ? 0 : $receiver.hashCode());
}
public boolean equals(Object obj) {
if (obj == this) { return true; }
if (!(obj instanceof Closure)) { return false; }
return ((Closure) obj).equalsParameters($receiver);
}
private boolean equalsParameters(final jedi.example.CommandExample $$receiver) {
return ($$receiver == null ? $receiver == null : $$receiver.equals($receiver));
}
}
return new Closure();
}
/**
* @see jedi.example.CommandExample#doY
*/
public jedi.functional.Command doYCommand() {
class Closure implements java.io.Serializable, jedi.functional.Command {
public void execute(final jedi.example.CommandExample $receiver) {
try {
$receiver.doY();
}
catch (RuntimeException ex) {
throw ex;
}
catch (Exception ex) {
throw new jedi.JediException(ex);
}
}
public int hashCode() {
return 17;
}
public boolean equals(Object obj) {
if (obj == this) { return true; }
if (!(obj instanceof Closure)) { return false; }
return true;
}
}
return new Closure();
}
/**
* @see jedi.example.CommandExample#doY
*/
public jedi.functional.Command0 doYProxyCommand0(final jedi.example.CommandExample $receiver) {
class Closure implements java.io.Serializable, jedi.functional.Command0 {
public void execute() {
try {
$receiver.doY();
}
catch (RuntimeException ex) {
throw ex;
}
catch (Exception ex) {
throw new jedi.JediException(ex);
}
}
public int hashCode() {
return (17) * 37 + ($receiver == null ? 0 : $receiver.hashCode());
}
public boolean equals(Object obj) {
if (obj == this) { return true; }
if (!(obj instanceof Closure)) { return false; }
return ((Closure) obj).equalsParameters($receiver);
}
private boolean equalsParameters(final jedi.example.CommandExample $$receiver) {
return ($$receiver == null ? $receiver == null : $$receiver.equals($receiver));
}
}
return new Closure();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy