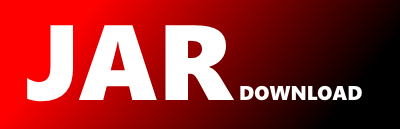
org.jfree.date.DayAndMonthRule Maven / Gradle / Ivy
Show all versions of jtstand-common Show documentation
/*
* Copyright (c) 2009 Albert Kurucz.
*
* This file, DayAndMonthRule.java is part of JTStand.
*
* JTStand is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* JTStand is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with GTStand. If not, see .
*/
package org.jfree.date;
/**
* An annual date rule where the generated date always falls on the same day
* and month each year.
*
* An example is ANZAC Day in Australia and New Zealand: it is observed on
* 25 April of every year.
*
* @author David Gilbert
*/
public class DayAndMonthRule extends AnnualDateRule {
/** The day of the month. */
private int dayOfMonth;
/** The month (uses 1 to 12 in the obvious way). */
private int month;
/**
* Default constructor: builds a DayAndMonthRule for 1 January.
*/
public DayAndMonthRule() {
this(1, MonthConstants.JANUARY);
}
/**
* Standard constructor: builds a DayAndMonthRule for the given
* day-of-the-month and month.
*
* For the month parameter, use SerialDate.JANUARY, etc. Note that there
* are no checks to prevent you from entering an invalid combination (such
* as 31 February).
*
* @param dayOfMonth the day of the month (in the range 1 to 31).
* @param month the month (use SerialDate.JANUARY, SerialDate.FEBRUARY etc.);
*/
public DayAndMonthRule(final int dayOfMonth, final int month) {
// check arguments delegated to setter methods...
setMonth(month);
setDayOfMonth(dayOfMonth);
}
/**
* Returns the day of the month.
*
* @return the day of the month.
*/
public int getDayOfMonth() {
return this.dayOfMonth;
}
/**
* Sets the day-of-the-month for this rule.
*
* @param dayOfMonth the day-of-the-month.
*/
public void setDayOfMonth(final int dayOfMonth) {
// check arguments...
if ((dayOfMonth < 1) || (dayOfMonth > SerialDate.LAST_DAY_OF_MONTH[this.month])) {
throw new IllegalArgumentException(
"DayAndMonthRule(): dayOfMonth outside valid range.");
}
// make the change...
this.dayOfMonth = dayOfMonth;
}
/**
* Returns an integer code representing the month.
*
* The codes JANUARY, FEBRUARY, MARCH, APRIL, MAY, JUNE, JULY, AUGUST,
* SEPTEMBER, OCTOBER, NOVEMBER and DECEMBER are defined in the SerialDate
* class.
*
* @return an integer code representing the month.
*/
public int getMonth() {
return this.month;
}
/**
* Sets the month for this rule.
*
* @param month the month for this rule.
*/
public void setMonth(final int month) {
// check arguments...
if (!SerialDate.isValidMonthCode(month)) {
throw new IllegalArgumentException("DayAndMonthRule(): month code not valid.");
}
// make the change...
this.month = month;
}
/**
* Returns the date, given the year.
*
* @param yyyy the year.
*
* @return the date generated by this rule for the specified year (null permitted).
*/
public SerialDate getDate(final int yyyy) {
return SerialDate.createInstance(this.dayOfMonth, this.month, yyyy);
}
}