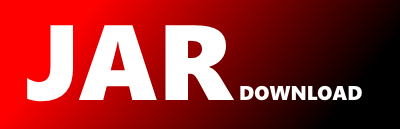
org.jfree.util.ClassComparator Maven / Gradle / Ivy
Show all versions of jtstand-common Show documentation
/*
* Copyright (c) 2009 Albert Kurucz.
*
* This file, ClassComparator.java is part of JTStand.
*
* JTStand is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* JTStand is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with GTStand. If not, see .
*/
package org.jfree.util;
import java.io.Serializable;
import java.util.Comparator;
/**
* The class comparator can be used to compare and sort classes and their
* superclasses. The comparator is not able to compare classes which have
* no relation...
*
* @author Thomas Morgner
*/
public class ClassComparator implements Comparator, Serializable {
/** For serialization. */
private static final long serialVersionUID = -5225335361837391120L;
/**
* Defaultconstructor.
*/
public ClassComparator() {
super();
}
/**
* Compares its two arguments for order. Returns a negative integer,
* zero, or a positive integer as the first argument is less than, equal
* to, or greater than the second.
*
* Note: throws ClassCastException if the arguments' types prevent them from
* being compared by this Comparator.
* And IllegalArgumentException if the classes share no relation.
*
* The implementor must ensure that sgn(compare(x, y)) ==
* -sgn(compare(y, x)) for all x and y. (This
* implies that compare(x, y) must throw an exception if and only
* if compare(y, x) throws an exception.)
*
* The implementor must also ensure that the relation is transitive:
* ((compare(x, y)>0) && (compare(y, z)>0)) implies
* compare(x, z)>0.
*
* Finally, the implementer must ensure that compare(x, y)==0
* implies that sgn(compare(x, z))==sgn(compare(y, z)) for all
* z.
*
* It is generally the case, but not strictly required that
* (compare(x, y)==0) == (x.equals(y)). Generally speaking,
* any comparator that violates this condition should clearly indicate
* this fact. The recommended language is "Note: this comparator
* imposes orderings that are inconsistent with equals."
*
* @param o1 the first object to be compared.
* @param o2 the second object to be compared.
* @return a negative integer, zero, or a positive integer as the
* first argument is less than, equal to, or greater than the
* second.
*/
public int compare(final Object o1, final Object o2) {
final Class c1 = (Class) o1;
final Class c2 = (Class) o2;
if (c1.equals(o2)) {
return 0;
}
if (c1.isAssignableFrom(c2)) {
return -1;
}
else {
if (!c2.isAssignableFrom(c2)) {
throw new IllegalArgumentException(
"The classes share no relation"
);
}
return 1;
}
}
/**
* Checks, whether the given classes are comparable. This method will
* return true, if one of the classes is assignable from the other class.
*
* @param c1 the first class to compare
* @param c2 the second class to compare
* @return true, if the classes share a direct relation, false otherwise.
*/
public boolean isComparable(final Class c1, final Class c2) {
return (c1.isAssignableFrom(c2) || c2.isAssignableFrom(c1));
}
}