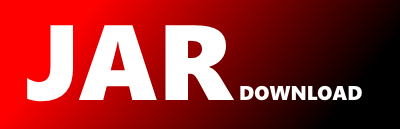
org.jfree.xml.factory.objects.Line2DObjectDescription Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jtstand-common Show documentation
Show all versions of jtstand-common Show documentation
jtstand-common is a library derived from jcommon, used by jtstand-chart, which is derived from jfreechart
/*
* Copyright (c) 2009 Albert Kurucz.
*
* This file, Line2DObjectDescription.java is part of JTStand.
*
* JTStand is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* JTStand is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with GTStand. If not, see .
*/
package org.jfree.xml.factory.objects;
import java.awt.geom.Line2D;
/**
* An object-description for a Line2D
object.
*
* @author Thomas Morgner
*/
public class Line2DObjectDescription extends AbstractObjectDescription {
/**
* Creates a new object description.
*/
public Line2DObjectDescription() {
super(Line2D.class);
setParameterDefinition("x1", Float.class);
setParameterDefinition("x2", Float.class);
setParameterDefinition("y1", Float.class);
setParameterDefinition("y2", Float.class);
}
/**
* Creates an object based on this description.
*
* @return The object.
*/
public Object createObject() {
final Line2D line = new Line2D.Float();
final float x1 = getFloatParameter("x1");
final float x2 = getFloatParameter("x2");
final float y1 = getFloatParameter("y1");
final float y2 = getFloatParameter("y2");
line.setLine(x1, y1, x2, y2);
return line;
}
/**
* Returns a parameter value as a float.
*
* @param param the parameter name.
*
* @return The float value.
*/
private float getFloatParameter(final String param) {
final Float p = (Float) getParameter(param);
if (p == null) {
return 0;
}
return p.floatValue();
}
/**
* Sets the parameters of this description object to match the supplied object.
*
* @param o the object (should be an instance of Line2D
).
*
* @throws ObjectFactoryException if the object is not an instance of Line2D
.
*/
public void setParameterFromObject(final Object o) throws ObjectFactoryException {
if (!(o instanceof Line2D)) {
throw new ObjectFactoryException("The given object is no java.awt.geom.Line2D.");
}
final Line2D line = (Line2D) o;
final float x1 = (float) line.getX1();
final float x2 = (float) line.getX2();
final float y1 = (float) line.getY1();
final float y2 = (float) line.getY2();
setParameter("x1", new Float(x1));
setParameter("x2", new Float(x2));
setParameter("y1", new Float(y1));
setParameter("y2", new Float(y2));
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy