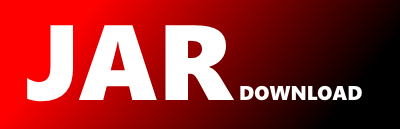
org.codehaus.mojo.cobertura.AbstractCoberturaMojo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cobertura-maven-plugin Show documentation
Show all versions of cobertura-maven-plugin Show documentation
This is the Mojo's Maven plugin for Cobertura. Cobertura is a free Java tool that calculates the percentage of code accessed by
tests. It can be used to identify which parts of your Java program are lacking test coverage.
package org.codehaus.mojo.cobertura;
/*
* Copyright 2011
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
import java.io.File;
import java.util.List;
import org.apache.maven.artifact.Artifact;
import org.apache.maven.plugin.AbstractMojo;
import org.apache.maven.plugin.MojoExecution;
import org.apache.maven.project.MavenProject;
import org.codehaus.mojo.cobertura.configuration.MaxHeapSizeUtil;
import org.codehaus.mojo.cobertura.tasks.AbstractTask;
/**
* Abstract Base for Cobertura Mojos.
*
* @author Joakim Erdfelt
*/
public abstract class AbstractCoberturaMojo
extends AbstractMojo
{
/**
* Maven Internal: Project to interact with.
*
* @parameter default-value="${project}"
* @required
* @readonly
*/
private MavenProject project;
/**
* Maximum memory to pass JVM as -Xmx of Cobertura processes.
*
* @parameter expression="${cobertura.maxmem}" default-value="64m"
*/
// Duplicate def to please MojoTestCase.
// Use only default-value once tests have been refactored to IT's
private String maxmem = "64m";
/**
*
* The Datafile Location.
*
*
* @parameter expression="${cobertura.datafile}" default-value="${project.build.directory}/cobertura/cobertura.ser"
* @required
* @readonly
*/
private File dataFile;
/**
* @parameter default-value="${mojoExecution}"
* @required
* @readonly
*/
private MojoExecution mojoExecution;
/**
* Only output coberura errors, avoid info messages.
*
* @parameter expression="${quiet}" default-value="false"
*/
private boolean quiet;
/**
* Maven Internal: List of artifacts for the plugin.
*
* @parameter default-value="${plugin.artifacts}"
* @required
* @readonly
*/
protected List pluginClasspathList;
/**
* When true
, skip the execution.
* @since 2.5
* @parameter expression="${cobertura.skip}"
* default-value="false"
*/
private boolean skip;
/**
* Usually most of out cobertura mojos will not get executed on parent poms.
* Setting this parameter to true
will force
* the execution of this mojo, even if it would usually get skipped in this case.
*
* @since 2.5
* @parameter expression="${cobertura.force}"
* default-value=false
* @required
*/
private boolean forceMojoExecution;
/**
* Setup the Task defaults.
*
* @param task the task to setup.
*/
protected void setTaskDefaults( AbstractTask task )
{
task.setLog( getLog() );
task.setPluginClasspathList( pluginClasspathList );
if ( MaxHeapSizeUtil.getInstance().envHasMavenMaxMemSetting() )
{
maxmem = MaxHeapSizeUtil.getInstance().getMavenMaxMemSetting();
}
else
{
task.setMaxmem( maxmem );
}
task.setQuiet( quiet );
}
/**
* Determine if the mojo execution should get skipped.
* This is the case if:
*
* - {@link #skip} is
true
* - if the mojo gets executed on a project with packaging type 'pom' and
* {@link #forceMojoExecution} is
false
*
*
* @return true
if the mojo execution should be skipped.
*/
protected boolean skipMojo()
{
if ( skip )
{
getLog().info( "Skipping cobertura execution" );
return true;
}
if ( !forceMojoExecution && "pom".equals( this.project.getPackaging() ) )
{
getLog().info( "Skipping cobertura mojo for project with packaging type 'pom'" );
return true;
}
return false;
}
/**
* Get the data file which is or will be generated by Cobertura, never null
.
*
* @return the data file
*/
protected File getDataFile()
{
return CoberturaMojoUtils.getDataFile( new File( this.project.getBuild().getDirectory() ), mojoExecution );
}
/**
* @return the project
*/
protected final MavenProject getProject()
{
return project;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy