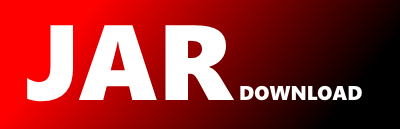
org.codehaus.mojo.cobertura.configuration.ConfigInstrumentation Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cobertura-maven-plugin Show documentation
Show all versions of cobertura-maven-plugin Show documentation
This is the Mojo's Maven plugin for Cobertura. Cobertura is a free Java tool that calculates the percentage of code accessed by
tests. It can be used to identify which parts of your Java program are lacking test coverage.
The newest version!
/*
* #%L
* Mojo's Maven plugin for Cobertura
* %%
* Copyright (C) 2005 - 2013 Codehaus
* %%
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* #L%
*/
package org.codehaus.mojo.cobertura.configuration;
import java.io.File;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
/**
* The Configuration for the Instrumentation.
*
* @author Joakim Erdfelt
*/
public class ConfigInstrumentation
{
private File basedir;
private List excludes;
private List ignores;
private List includes;
private String maxmem;
private boolean ignoreTrivial;
private List ignoreMethodAnnotations;
/**
* Construct a new ConfigInstrumentation object.
*/
public ConfigInstrumentation()
{
this.includes = new ArrayList();
this.excludes = new ArrayList();
this.ignores = new ArrayList();
this.ignoreTrivial = false;
this.ignoreMethodAnnotations = new ArrayList();
this.basedir = new File( System.getProperty( "user.dir" ) );
if ( MaxHeapSizeUtil.getInstance().envHasMavenMaxMemSetting() )
{
maxmem = MaxHeapSizeUtil.getInstance().getMavenMaxMemSetting();
}
else
{
this.maxmem = "64m";
}
}
/**
* Add an Exclude to the underlying list.
*
* @param exclude the exlude string.
*/
public void addExclude( String exclude )
{
this.excludes.add( exclude );
}
/**
* Add an Ignore to the underlying list.
*
* @param ignore the ignore string.
*/
public void addIgnore( String ignore )
{
this.ignores.add( ignore );
}
/**
* Add an IgnoreMethodAnnotation to the underlying list.
*
* @param ignoreMethodAnnotation the ignore string.
*/
public void addIgnoreMethodAnnotation( String ignoreMethodAnnotation )
{
this.ignoreMethodAnnotations.add( ignoreMethodAnnotation );
}
/**
* Add an Include ot the underlying list.
*
* @param include the include string.
*/
public void addInclude( String include )
{
this.includes.add( include );
}
/**
* @return Returns the basedir.
*/
public File getBasedir()
{
return basedir;
}
/**
* Get the Exclude List.
*
* @return the exlude list.
*/
public List getExcludes()
{
return excludes;
}
/**
* Get the Ignore List.
*
* @return the ignore list.
*/
public List getIgnores()
{
return ignores;
}
/**
* Get the Include List.
*
* @return the include list.
*/
public List getIncludes()
{
return includes;
}
/**
* @param basedir The basedir to set.
*/
public void setBasedir( File basedir )
{
this.basedir = basedir;
}
/**
* Get the maxmem setting.
*
* @return the maxmem setting.
*/
public String getMaxmem()
{
return maxmem;
}
/**
* Sets the max memory for the JVM used to run the InstrumentationTask
task.
* The format is "". Ex: "64m" where 64 is the value, and "m" denotes megabytes.
*
* @param maxmem the value to which maxmem will be set
*/
public void setMaxmem( String maxmem )
{
this.maxmem = maxmem;
}
/**
* Get the ignoreTrivial setting.
*
* @return the ignoreTrivial setting.
*/
public boolean getIgnoreTrivial()
{
return ignoreTrivial;
}
/**
* IgnoreTrivial switch that tells Cobertura to ignore the following in the coverage report:
* Getter methods that simply read a class field; Setter methods that set a class field;
* Constructors that only set class fields and call a super class constructor.
*
* @param ignoreTrivial enable ignoreTrivial
*/
public void setIgnoreTrivial( boolean ignoreTrivial )
{
this.ignoreTrivial = ignoreTrivial;
}
/**
* Get the ignoreMethodAnnotations setting.
*
* @return the ignoreMethodAnnotations setting.
*/
public List getIgnoreMethodAnnotations()
{
return ignoreMethodAnnotations;
}
/**
* IgnoreMethodAnnotation switch used to specify an annotation that, when present on a method,
* will cause Cobertura to ignore the method in the coverage report.
*
* @param ignoreMethodAnnotations
*/
public void setIgnoreMethodAnnotations( List ignoreMethodAnnotations )
{
this.ignoreMethodAnnotations = ignoreMethodAnnotations;
}
/**
* {@inheritDoc}
*/
public String toString()
{
StringBuffer sb = new StringBuffer();
sb.append( " it = this.includes.iterator();
while ( it.hasNext() )
{
String include = (String) it.next();
sb.append( include );
if ( it.hasNext() )
{
sb.append( " " );
}
}
sb.append( "\"" );
}
if ( !this.excludes.isEmpty() )
{
sb.append( " excludes=\"" );
Iterator it = this.excludes.iterator();
while ( it.hasNext() )
{
String exclude = (String) it.next();
sb.append( exclude );
if ( it.hasNext() )
{
sb.append( " " );
}
}
sb.append( "\"" );
}
if ( !this.ignores.isEmpty() )
{
sb.append( " ignores=\"" );
Iterator it = this.ignores.iterator();
while ( it.hasNext() )
{
String ignore = (String) it.next();
sb.append( ignore );
if ( it.hasNext() )
{
sb.append( " " );
}
}
sb.append( "\"" );
}
if ( 0 != getMaxmem().length() )
{
sb.append( " maxmem=\"" );
sb.append( getMaxmem() );
sb.append( "\"" );
}
sb.append( " ignoreTrivial=\"" );
sb.append( getIgnoreTrivial() );
sb.append( "\"" );
if ( !this.ignoreMethodAnnotations.isEmpty() )
{
sb.append( " ignoreMethodAnnotations=\"" );
Iterator it = this.ignoreMethodAnnotations.iterator();
while ( it.hasNext() )
{
String ignore = (String) it.next();
sb.append( ignore );
if ( it.hasNext() )
{
sb.append( " " );
}
}
sb.append( "\"" );
}
return sb.append( " />" ).toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy