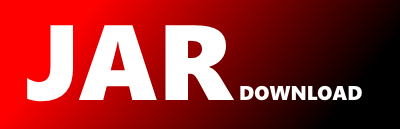
org.codehaus.mojo.flatten.PomProperty Maven / Gradle / Ivy
package org.codehaus.mojo.flatten;
import java.io.File;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Properties;
import org.apache.maven.model.Build;
import org.apache.maven.model.CiManagement;
import org.apache.maven.model.Contributor;
import org.apache.maven.model.Dependency;
import org.apache.maven.model.DependencyManagement;
import org.apache.maven.model.Developer;
import org.apache.maven.model.DistributionManagement;
import org.apache.maven.model.IssueManagement;
import org.apache.maven.model.License;
import org.apache.maven.model.MailingList;
import org.apache.maven.model.Model;
import org.apache.maven.model.Organization;
import org.apache.maven.model.Parent;
import org.apache.maven.model.PluginManagement;
import org.apache.maven.model.Prerequisites;
import org.apache.maven.model.Profile;
import org.apache.maven.model.Reporting;
import org.apache.maven.model.Repository;
import org.apache.maven.model.Scm;
/**
* This class reflects a property of a {@link Model POM}. It contains {@link #getPomProperties() all available
* properties} as constants and allows generic access to {@link #get(Model) read} and {@link #set(Model, Object) write}
* the property via a {@link Model}.
*
* @param is the generic type of the {@link #get(Model) property value}.
* @author Joerg Hohwiller (hohwille at users.sourceforge.net)
* @since 1.0.0-beta-4
*/
public abstract class PomProperty
{
/** @see Model#getArtifactId() */
public static final PomProperty ARTIFACT_ID = new PomProperty( "artifactId", String.class)
{
@Override
public String get( Model model )
{
return model.getArtifactId();
}
@Override
public void set( Model model, String value )
{
model.setArtifactId( value );
};
};
/** @see Model#getBuild() */
public static final PomProperty BUILD = new PomProperty( "build", Build.class)
{
@Override
public Build get( Model model )
{
Build result = model.getBuild();
return result;
}
@Override
public void set( Model model, Build value )
{
model.setBuild( value );
};
};
/** @see Model#getCiManagement() */
public static final PomProperty CI_MANAGEMENT =
new PomProperty( "ciManagement", CiManagement.class)
{
@Override
public CiManagement get( Model model )
{
return model.getCiManagement();
}
@Override
public void set( Model model, CiManagement value )
{
model.setCiManagement( value );
};
};
/** @see Model#getContributors() */
@SuppressWarnings( { "rawtypes", "unchecked" } )
public static final PomProperty> CONTRIBUTORS =
new PomProperty>( "contributors", (Class) List.class)
{
@Override
public List get( Model model )
{
return model.getContributors();
}
@Override
public void set( Model model, List value )
{
model.setContributors( value );
};
};
/** @see Model#getDependencies() */
@SuppressWarnings( { "rawtypes", "unchecked" } )
public static final PomProperty> DEPENDENCIES =
new PomProperty>( "dependencies", (Class) List.class)
{
@Override
public List get( Model model )
{
return model.getDependencies();
}
@Override
public void set( Model model, List value )
{
model.setDependencies( value );
};
};
/** @see Model#getDependencyManagement() */
public static final PomProperty DEPENDENCY_MANAGEMENT =
new PomProperty( "dependencyManagement", DependencyManagement.class)
{
@Override
public DependencyManagement get( Model model )
{
return model.getDependencyManagement();
}
@Override
public void set( Model model, DependencyManagement value )
{
model.setDependencyManagement( value );
};
};
/** @see Model#getDescription() */
public static final PomProperty DESCRIPTION = new PomProperty( "description", String.class)
{
@Override
public String get( Model model )
{
return model.getDescription();
}
@Override
public void set( Model model, String value )
{
model.setDescription( value );
};
};
/** @see Model#getDevelopers() */
@SuppressWarnings( { "rawtypes", "unchecked" } )
public static final PomProperty> DEVELOPERS =
new PomProperty>( "developers", (Class) List.class)
{
@Override
public List get( Model model )
{
return model.getDevelopers();
}
@Override
public void set( Model model, List value )
{
model.setDevelopers( value );
};
};
/** @see Model#getDistributionManagement() */
public static final PomProperty DISTRIBUTION_MANAGEMENT =
new PomProperty( "distributionManagement", DistributionManagement.class)
{
@Override
public DistributionManagement get( Model model )
{
return model.getDistributionManagement();
}
@Override
public void set( Model model, DistributionManagement value )
{
model.setDistributionManagement( value );
};
};
/** @see Model#getGroupId() */
public static final PomProperty GROUP_ID = new PomProperty( "groupId", String.class)
{
@Override
public String get( Model model )
{
return model.getGroupId();
}
@Override
public void set( Model model, String value )
{
model.setGroupId( value );
};
};
/** @see Model#getInceptionYear() */
public static final PomProperty INCEPTION_YEAR = new PomProperty( "inceptionYear", String.class)
{
@Override
public String get( Model model )
{
return model.getInceptionYear();
}
@Override
public void set( Model model, String value )
{
model.setInceptionYear( value );
};
};
/** @see Model#getIssueManagement() */
public static final PomProperty ISSUE_MANAGEMENT =
new PomProperty( "issueManagement", IssueManagement.class)
{
@Override
public IssueManagement get( Model model )
{
return model.getIssueManagement();
}
@Override
public void set( Model model, IssueManagement value )
{
model.setIssueManagement( value );
};
};
/** @see Model#getLicenses() */
@SuppressWarnings( { "rawtypes", "unchecked" } )
public static final PomProperty> LICENSES =
new PomProperty>( "licenses", (Class) List.class)
{
@Override
public List get( Model model )
{
return model.getLicenses();
}
@Override
public void set( Model model, List value )
{
model.setLicenses( value );
};
};
/** @see Model#getMailingLists() */
@SuppressWarnings( { "rawtypes", "unchecked" } )
public static final PomProperty> MAILING_LISTS =
new PomProperty>( "mailingLists", (Class) List.class)
{
@Override
public List get( Model model )
{
return model.getMailingLists();
}
@Override
public void set( Model model, List value )
{
model.setMailingLists( value );
};
};
/** @see Model#getModelEncoding() */
public static final PomProperty MODEL_ENCODING = new PomProperty( "modelEncoding", String.class)
{
@Override
public String get( Model model )
{
return model.getModelEncoding();
}
@Override
public void set( Model model, String value )
{
model.setModelEncoding( value );
};
};
/** @see Model#getModelVersion() */
public static final PomProperty MODEL_VERSION = new PomProperty( "modelVersion", String.class)
{
@Override
public String get( Model model )
{
return model.getModelVersion();
}
@Override
public void set( Model model, String value )
{
model.setModelVersion( value );
};
};
/** @see Model#getModules() */
@SuppressWarnings( { "rawtypes", "unchecked" } )
public static final PomProperty> MODULES =
new PomProperty>( "modules", (Class) List.class)
{
@Override
public List get( Model model )
{
return model.getModules();
}
@Override
public void set( Model model, List value )
{
model.setModules( value );
};
};
/** @see Model#getName() */
public static final PomProperty NAME = new PomProperty( "name", String.class)
{
@Override
public String get( Model model )
{
return model.getName();
}
@Override
public void set( Model model, String value )
{
model.setName( value );
};
};
/** @see Model#getOrganization() */
public static final PomProperty ORGANIZATION =
new PomProperty( "organization", Organization.class)
{
@Override
public Organization get( Model model )
{
return model.getOrganization();
}
@Override
public void set( Model model, Organization value )
{
model.setOrganization( value );
};
};
/** @see Model#getPackaging() */
public static final PomProperty PACKAGING = new PomProperty( "packaging", String.class)
{
@Override
public String get( Model model )
{
return model.getPackaging();
}
@Override
public void set( Model model, String value )
{
model.setPackaging( value );
};
};
/** @see Model#getParent() */
public static final PomProperty PARENT = new PomProperty( "parent", Parent.class)
{
@Override
public Parent get( Model model )
{
return model.getParent();
}
@Override
public void set( Model model, Parent value )
{
model.setParent( value );
};
};
/** @see Build#getPluginManagement() */
public static final PomProperty PLUGIN_MANAGEMENT =
new PomProperty( "pluginManagement", PluginManagement.class)
{
@Override
public PluginManagement get( Model model )
{
if ( model.getBuild() == null )
return null;
return model.getBuild().getPluginManagement();
}
@Override
public void set( Model model, PluginManagement value )
{
if ( model.getBuild() == null )
model.setBuild( new Build() );
model.getBuild().setPluginManagement( value );
};
};
/** @see Model#getPluginRepositories() */
@SuppressWarnings( { "rawtypes", "unchecked" } )
public static final PomProperty> PLUGIN_REPOSITORIES =
new PomProperty>( "pluginRepositories", (Class) List.class)
{
@Override
public List get( Model model )
{
return model.getPluginRepositories();
}
@Override
public void set( Model model, List value )
{
model.setPluginRepositories( value );
};
};
/** @see Model#getPomFile() */
public static final PomProperty POM_FILE = new PomProperty( "pomFile", File.class)
{
@Override
public File get( Model model )
{
return model.getPomFile();
}
@Override
public void set( Model model, File value )
{
model.setPomFile( value );
};
};
/** @see Model#getPrerequisites() */
public static final PomProperty PREREQUISITES =
new PomProperty( "prerequisites", Prerequisites.class)
{
@Override
public Prerequisites get( Model model )
{
return model.getPrerequisites();
}
@Override
public void set( Model model, Prerequisites value )
{
model.setPrerequisites( value );
};
};
/** @see Model#getProfiles() */
@SuppressWarnings( { "rawtypes", "unchecked" } )
public static final PomProperty> PROFILES =
new PomProperty>( "profiles", (Class) List.class)
{
@Override
public List get( Model model )
{
return model.getProfiles();
}
@Override
public void set( Model model, List value )
{
model.setProfiles( value );
};
};
/** @see Model#getProperties() */
public static final PomProperty PROPERTIES =
new PomProperty( "properties", Properties.class)
{
@Override
public Properties get( Model model )
{
return model.getProperties();
}
@Override
public void set( Model model, Properties value )
{
model.setProperties( value );
};
};
/** @see Model#getReporting() */
public static final PomProperty REPORTING = new PomProperty( "reporting", Reporting.class)
{
@Override
public Reporting get( Model model )
{
return model.getReporting();
}
@Override
public void set( Model model, Reporting value )
{
model.setReporting( value );
};
};
/** @see Model#getPluginRepositories() */
@SuppressWarnings( { "rawtypes", "unchecked" } )
public static final PomProperty> REPOSITORIES =
new PomProperty>( "repositories", (Class) List.class)
{
@Override
public List get( Model model )
{
return model.getRepositories();
}
@Override
public void set( Model model, List value )
{
model.setRepositories( value );
};
};
/** @see Model#getScm() */
public static final PomProperty SCM = new PomProperty( "scm", Scm.class)
{
@Override
public Scm get( Model model )
{
return model.getScm();
}
@Override
public void set( Model model, Scm value )
{
model.setScm( value );
};
};
/** @see Model#getUrl() */
public static final PomProperty URL = new PomProperty( "url", String.class)
{
@Override
public String get( Model model )
{
return model.getUrl();
}
@Override
public void set( Model model, String value )
{
model.setUrl( value );
};
};
/** @see Model#getVersion() */
public static final PomProperty VERSION = new PomProperty( "version", String.class)
{
@Override
public String get( Model model )
{
return model.getVersion();
}
@Override
public void set( Model model, String value )
{
model.setVersion( value );
};
};
private static final PomProperty>[] POM_PROPERTIES_ARRAY = new PomProperty>[] { ARTIFACT_ID, BUILD,
CI_MANAGEMENT, CONTRIBUTORS, DEPENDENCIES, DEPENDENCY_MANAGEMENT, DESCRIPTION, DEVELOPERS,
DISTRIBUTION_MANAGEMENT, GROUP_ID, INCEPTION_YEAR, ISSUE_MANAGEMENT, LICENSES, MAILING_LISTS, MODEL_ENCODING,
MODEL_VERSION, MODULES, NAME, ORGANIZATION, PACKAGING, PARENT, PLUGIN_MANAGEMENT, PLUGIN_REPOSITORIES, POM_FILE,
PREREQUISITES, PROFILES, PROPERTIES, REPORTING, REPOSITORIES, SCM, URL, VERSION };
private static final List> POM_PROPERTIES =
Collections.unmodifiableList( Arrays.asList( POM_PROPERTIES_ARRAY ) );
private final String name;
private final Class valueType;
/**
* The constructor.
*
* @param name - see {@link #getName()}.
* @param valueType - see {@link #getValueType()}.
*/
public PomProperty( String name, Class valueType )
{
super();
this.name = name;
this.valueType = valueType;
}
/**
* @return name
*/
public String getName()
{
return this.name;
}
/**
* @return {@link Class} reflecting the type of the {@link #get(Model) property value}.
*/
public Class getValueType()
{
return this.valueType;
}
/**
* @return true
if required for flattened POM, false
otherwise.
*/
public boolean isRequired()
{
if ( ( this == GROUP_ID ) || ( this == ARTIFACT_ID ) || ( this == VERSION ) )
{
return true;
}
return false;
}
/**
* @return true
if this property represents an XML element of the POM representation,
* false
otherwise (if an internal property such as {@link Model#getPomFile()}).
*/
public boolean isElement()
{
if ( this == POM_FILE )
{
return false;
}
if ( this == MODEL_ENCODING )
{
return false;
}
return true;
}
/**
* Generic getter for reading a {@link PomProperty} from a {@link Model}.
*
* @param model is the {@link Model} to read from.
* @return the value of the property to read identified by this {@link PomProperty}.
*/
public abstract V get( Model model );
/**
* Generic setter for writing a {@link PomProperty} in a {@link Model}.
*
* @param model is the {@link Model} to write to.
* @param value is the value of the property to write identified by this {@link PomProperty}.
*/
public abstract void set( Model model, V value );
/**
* Copies the value identified by this {@link PomProperty} from the given source
{@link Model} to the
* given target
{@link Model}.
*
* @param source is the {@link Model} to copy from (read).
* @param target is the {@link Model} to copy to (write).
*/
public void copy( Model source, Model target )
{
V value = get( source );
if ( value != null )
{
set( target, value );
}
}
/**
* @return an {@link Collections#unmodifiableList(List) unmodifiable} {@link List} with all {@link PomProperty
* properties}.
*/
public static List> getPomProperties()
{
return POM_PROPERTIES;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy