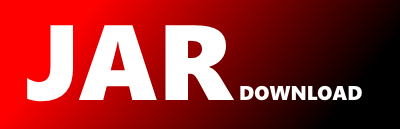
org.codehaus.mojo.weblogic.ClientGen9Mojo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of weblogic-maven-plugin Show documentation
Show all versions of weblogic-maven-plugin Show documentation
This plugin will support various tasks within the Weblogic 8.1,
9.x, 10.x and 12.x environment. This version starts to support weblogic 10 and
11. The mojo supports such tasks as deploy,
undeploy,clientgen,servicegen, and appc are supported as well as
many others. The plugin uses exposed API's that are subject to
change but have been tested in 8.1 SP 4-6 and 9.0 - 9.2 MP3, 10.x.
There are two versions of the plugin to support the two
environments based on differences in the JDK. The 9.x version is
currently being refactored to support the standard JSR supported
deployment interface. The code used in the plugin has been
contributed to the Cargo project however to date has not be
integrated into the codebase. Please feel free to suggest
improvements or help support this plugin effort.
The newest version!
package org.codehaus.mojo.weblogic;
/*
* Copyright 2008 The Apache Software Foundation.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
import org.apache.maven.plugin.MojoExecutionException;
import org.apache.tools.ant.BuildListener;
import org.apache.tools.ant.DefaultLogger;
import org.apache.tools.ant.Project;
import org.apache.tools.ant.types.FileSet;
import org.apache.tools.ant.types.Path;
import org.codehaus.mojo.weblogic.util.Bootstrapper;
import org.codehaus.mojo.weblogic.util.WeblogicMojoUtilities;
import weblogic.wsee.tools.anttasks.ClientGenTask;
import weblogic.wsee.tools.anttasks.JwsModule;
import weblogic.wsee.tools.anttasks.MultipleJwsModule;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.ObjectInputStream;
import java.io.ObjectOutputStream;
import java.io.Serializable;
import java.net.URI;
/**
* Runs Client Gen on a given WSDL. This client gen uses the BEA refactored client gen tool
* first appearing in weblogic 9. This is the preferred client gen tool for Weblogic 9.0 and
* newer.
*
* @author Jon Osborn
* @version $Id: ClientGen9Mojo.java 17525 2012-09-16 13:39:50Z jonnio $
* @description This mojo will run client gen on a given WSDL. This client gen uses the BEA refactored client gen tool
* first appearing in weblogic 9. This is the preferred client gen tool for Weblogic 9.0 and newer.
* @goal clientgen9
* @requiresDependencyResolution compile
*/
public class ClientGen9Mojo
extends AbstractWeblogicMojo
{
/**
* The filename of the war file to find the services. The file path is
* extracted from the artifact list.
*
* @parameter
*/
private String warFileName;
/**
* The wsdl to client gen from. If warFileName is specified, this parameter
* is the root relative file to use when creating the URI for the wsdl.
*
* @parameter
*/
private String inputWSDL;
/**
* The directory to output the generated code to.
*
* @parameter default-value="${basedir}/src/main/java"
*/
private String outputDir;
/**
* The package name of the output code.
*
* @parameter default-value="com.test.webservice"
*/
private String packageName;
/**
* The name of the service.
*
* @parameter
*/
private String serviceName;
/**
* Output verbose messages
*
* @parameter default-value="false"
*/
private boolean verbose;
/**
* Whether or not to use server types from the ear file in the client jar.
*
* @parameter default-value="false"
*/
private boolean useServerTypes;
/**
* Sets whether or not to create the type conversions for a web service in
* the client.
*
* @parameter default-value="true"
*/
private boolean autotype;
/**
* Sets whether or not to use the jaxRPCWrappedArrayStyle
*
* @parameter default-value="true"
*/
private boolean jaxRPCWrappedArrayStyle;
/**
* Sets whether or not to use the async method wrappers
*
* @parameter default-value="false"
*/
private boolean generateAsyncMethods;
/**
* The client handler chain configuration file.
*
* @parameter
*/
private String handlerChainFile;
/**
* Set this to skip JWSC compiling
*
* @parameter default-value="false" expression="${weblogic.clientgen.skip}"
*/
private boolean skipClientGen;
/**
* @param skipClientGen the skipJwsc to set
*/
public void setSkipClientGen( boolean skipClientGen )
{
this.skipClientGen = skipClientGen;
}
/**
* Set this to set the JWS tpe
*
* @parameter default-value="JAXRPC" expression="${weblogic.jwsc.type}"
*/
private String wsType;
/**
* Set the WS type 'JAXWS' or 'JAXRPC'
*
* @param wsType 'JAXWS' or 'JAXRPC'
*/
public void setWsType( String wsType )
{
this.wsType = wsType;
}
/**
* The debug setting
*
* @parameter default-value="false"
*/
private boolean debug;
/**
* The debug setter
*
* @param debug the setter
*/
public void setDebug( boolean debug )
{
this.debug = debug;
}
/**
* The binding files
*
* @parameter
*/
private String bindingFile;
/**
* The binding files setter
*
* @param bindingFile
*/
private void setBindingFile( String bindingFile )
{
this.bindingFile = bindingFile;
}
/**
* The getter
*
* @return if true
*/
public boolean isDebug()
{
return debug;
}
/**
* the binding file
*
* @return the binding file
*/
public String getBindingFile()
{
return bindingFile;
}
/**
* skip clientgen
*
* @return skip
*/
public boolean isSkipClientGen()
{
return skipClientGen;
}
/**
* The ws type
*
* @return
*/
public String getWsType()
{
return wsType;
}
/**
* This method will run client gen on the given WSDL.
*
* @throws MojoExecutionException Thrown if we fail to obtain the WSDL.
*/
public void execute()
throws MojoExecutionException
{
super.execute();
if ( this.skipClientGen )
{
getLog().info( "skipping client gen" );
return;
}
File tmpFile = null;
try
{
final String executeClass = ClientGenExecutor.class.getName();
final URI[] classPathUrls =
WeblogicMojoUtilities.getDependenciesAsUri( this.getArtifacts(), this.getPluginArtifacts() );
final StringBuffer path = new StringBuffer( 1024 );
//append weblogic.jar as the first thing on the classpath
path.append( getWeblogicJar().toURI() ).append( ' ' );
for ( URI url : classPathUrls )
{
path.append( url ).append( ' ' );
}
path.append( Bootstrapper.class.getProtectionDomain().getCodeSource().getLocation().toURI() );
final ClientGenParameters params = new ClientGenParameters();
params.setClassPath(
WeblogicMojoUtilities.getDependencies( this.getArtifacts(), this.getPluginArtifacts() ) );
params.setDebug( this.debug );
params.setDestDir( new File( this.outputDir ) );
params.setDestEncoding( "UTF-8" );
params.setNoWarn( false );
params.setSrcEncoding( "UTF-8" );
params.setVerbose( this.verbose );
params.setWsType( this.wsType != null && this.wsType.trim().length() > 0 ? this.wsType : null );
params.packageName = this.packageName;
params.useServerTypes = this.useServerTypes;
params.serviceName = this.serviceName;
params.handlerChainFile = this.handlerChainFile;
params.bindingFile = this.bindingFile;
params.srcDir = new File( this.outputDir );
//calculate the wsdl location
String wsdlUri = null;
if ( this.warFileName != null )
{
if ( getLog().isInfoEnabled() )
{
getLog().info(
" calculating wsdl URI from warFileName " + this.warFileName + " with wsdl " + this.inputWSDL );
}
wsdlUri =
"jar:file:" + WeblogicMojoUtilities.getWarFileName( this.getArtifacts(), this.warFileName ) + "!"
+ this.inputWSDL;
new File( this.inputWSDL ).toURI().toString();
if ( getLog().isInfoEnabled() )
{
getLog().info( " using " + wsdlUri + " for clientgen." );
}
}
else if ( this.inputWSDL.startsWith( "http" ) )
{
if ( getLog().isInfoEnabled() )
{
getLog().info( " using " + this.inputWSDL + " for clientgen." );
}
wsdlUri = this.inputWSDL;
}
else
{
wsdlUri = new File( this.inputWSDL ).toURI().toString();
if ( getLog().isInfoEnabled() )
{
getLog().info( " using " + wsdlUri + " for clientgen." );
}
}
params.inputWSDL = wsdlUri;
tmpFile = serialize( params );
Bootstrapper.exec( path.toString(),
new String[]{ executeClass, tmpFile != null ? tmpFile.getPath() : "" } );
}
catch ( Exception e )
{
throw new MojoExecutionException( "Exception while bootstrapping clientGen", e );
}
finally
{
if ( tmpFile != null )
{
tmpFile.delete();
}
}
}
/**
* Send the parameters to a temp file and return the file name
*
* @param params the params
* @return the file name
* @throws IOException the exceptions
*/
private static File serialize( ClientGenParameters params )
throws IOException
{
FileOutputStream file = null;
ObjectOutputStream ois = null;
File tmpFile = null;
try
{
tmpFile = File.createTempFile( "clientGenParams", ".bin" );
file = new FileOutputStream( tmpFile );
ois = new ObjectOutputStream( file );
ois.writeObject( params );
}
finally
{
if ( ois != null )
{
try
{
ois.close();
}
catch ( IOException e )
{
//eat
}
}
if ( file != null )
{
try
{
file.close();
}
catch ( IOException e )
{
//eat
}
}
}
return tmpFile;
}
/**
* Getter for property input WSDL.
*
* @return The value of input WSDL.
*/
public String getInputWSDL()
{
return this.inputWSDL;
}
/**
* Setter for the input WSDL.
*
* @param inInputWSDL The value of input WSDL.
*/
public void setInputWSDL( final String inInputWSDL )
{
this.inputWSDL = inInputWSDL;
}
/**
* Getter for property output dir.
*
* @return The value of output dir.
*/
public String getOutputDir()
{
return this.outputDir;
}
/**
* Setter for the output dir.
*
* @param inOutputDir The value of output dir.
*/
public void setOutputDir( final String inOutputDir )
{
this.outputDir = inOutputDir;
}
/**
* Getter for property package name.
*
* @return The value of package name.
*/
public String getPackageName()
{
return this.packageName;
}
/**
* Setter for the package name.
*
* @param inPackageName The value of package name.
*/
public void setPackageName( String inPackageName )
{
this.packageName = inPackageName;
}
/**
* Getter for property service name.
*
* @return The value of service name.
*/
public String getServiceName()
{
return this.serviceName;
}
/**
* Setter for the service name.
*
* @param inServiceName The value of service name.
*/
public void setServiceName( final String inServiceName )
{
this.serviceName = inServiceName;
}
/**
* toString method: creates a String representation of the object
*
* @return the String representation
*/
@Override
public String toString()
{
final StringBuilder sb = new StringBuilder();
sb.append( "ClientGen9Mojo" );
sb.append( "{warFileName='" ).append( warFileName ).append( '\'' );
sb.append( ", inputWSDL='" ).append( inputWSDL ).append( '\'' );
sb.append( ", outputDir='" ).append( outputDir ).append( '\'' );
sb.append( ", packageName='" ).append( packageName ).append( '\'' );
sb.append( ", serviceName='" ).append( serviceName ).append( '\'' );
sb.append( ", verbose=" ).append( verbose );
sb.append( ", useServerTypes=" ).append( useServerTypes );
sb.append( ", autotype=" ).append( autotype );
sb.append( ", jaxRPCWrappedArrayStyle=" ).append( jaxRPCWrappedArrayStyle );
sb.append( ", generateAsyncMethods=" ).append( generateAsyncMethods );
sb.append( ", handlerChainFile='" ).append( handlerChainFile ).append( '\'' );
sb.append( ", skipClientGen=" ).append( skipClientGen );
sb.append( ", wsType='" ).append( wsType ).append( '\'' );
sb.append( '}' );
return sb.toString();
}
/**
* Getter for server types
*
* @return true if the client gen should use server type information
*/
public boolean isUseServerTypes()
{
return useServerTypes;
}
/**
* Setter for server types
*
* @param useServerTypes - true if the client gen should use server types
*/
public void setUseServerTypes( boolean useServerTypes )
{
this.useServerTypes = useServerTypes;
}
/**
* Getter for verbose messages
*
* @return true if the client gen should use verbose output
*/
public boolean isVerbose()
{
return this.verbose;
}
/**
* Setter for verbose messages
*
* @param verbose - true of the clientgen should use verbose output
*/
public void setVerbose( boolean verbose )
{
this.verbose = verbose;
}
/**
* Getter for autoType
*
* @return true if clientgen shoud autotype from the wsdl
*/
public boolean isAutotype()
{
return this.autotype;
}
/**
* Setter for autoType
*
* @param autotype - true if the client should autotype
*/
public void setAutotype( boolean autotype )
{
this.autotype = autotype;
}
/**
* Getter for warFileName
*
* @return the warFileName
*/
public String getWarFileName()
{
return this.warFileName;
}
/**
* Setter for warFileName
*
* @param warFileName - the warFileName to set
*/
public void setWarFileName( String warFileName )
{
this.warFileName = warFileName;
}
/**
* Getter for jaxRPCWrappedArrayStyle
*
* @return the jaxRPCWrappedArrayStyle
*/
public boolean isJaxRPCWrappedArrayStyle()
{
return jaxRPCWrappedArrayStyle;
}
/**
* Setter for jaxRPCWrappedArrayStyle
*
* @param jaxRPCWrappedArrayStyle the jaxRPCWrappedArrayStyle to set
*/
public void setJaxRPCWrappedArrayStyle( boolean jaxRPCWrappedArrayStyle )
{
this.jaxRPCWrappedArrayStyle = jaxRPCWrappedArrayStyle;
}
public boolean isGenerateAsyncMethods()
{
return generateAsyncMethods;
}
public void setGenerateAsyncMethods( boolean generateAsyncMethods )
{
this.generateAsyncMethods = generateAsyncMethods;
}
public String getHandlerChainFile()
{
return handlerChainFile;
}
public void setHandlerChainFile( String handlerChainFile )
{
this.handlerChainFile = handlerChainFile;
}
/**
* Adapter class for bootstrapping the classpath.
* arg[0] must be the file name of the serialized parameters
*/
public static class ClientGenExecutor
{
public static void main( String[] args )
throws Exception
{
//the first argument is the serialized version of our jwsc parameters
ObjectInputStream ois = null;
try
{
ois = new ObjectInputStream( new FileInputStream( args[0] ) );
ClientGenParameters params = (ClientGenParameters) ois.readObject();
execute( params );
}
finally
{
if ( ois != null )
{
ois.close();
}
}
}
private static void execute( ClientGenParameters params )
throws MojoExecutionException
{
System.out.println( params );
try
{
if ( params.destDir != null && params.destDir.exists() )
{
boolean mk = params.destDir.mkdirs();
if ( !mk )
{
System.out.println( String.format( "Did not make the output dir %s", params.destDir ) );
}
}
ClientGenTask clientGen = new ClientGenTask();
// Set the classpath
Project project = new Project();
project.setName( "clientgen" );
String pathString = params.getClassPath();
Path path = new Path( project, pathString );
clientGen.setProject( project );
clientGen.setClasspath( path );
clientGen.setVerbose( params.verbose );
clientGen.setDebug( params.debug );
clientGen.setSrcdir( params.srcDir );
clientGen.setDestDir( params.destDir );
clientGen.setPackageName( params.packageName );
clientGen.setIncludeGlobalTypes( params.useServerTypes );
if ( params.bindingFile != null && params.bindingFile.length() > 0 )
{
final FileSet binding = new FileSet();
final File bindingFile = new File( params.bindingFile );
binding.setFile( bindingFile );
binding.setProject( project );
clientGen.addBinding( binding );
}
clientGen.setWsdl( params.inputWSDL );
if ( params.handlerChainFile != null )
{
clientGen.setHandlerChainFile( new File( params.handlerChainFile ) );
}
if ( params.serviceName != null )
{
clientGen.setServiceName( params.serviceName );
}
if ( params.wsType != null && params.wsType.trim().length() > 0 )
{
clientGen.setType( params.wsType );
}
clientGen.setListfiles( true );
clientGen.execute();
}
catch ( Exception ex )
{
throw new RuntimeException( "Exception encountered during clientGen", ex );
}
}
private static void setupDescriptors( ClientGenParameters params, MultipleJwsModule module )
{
if ( params.getDescriptors() != null )
{
final String[] files = params.getDescriptors().split( "\\," );
for ( int i = 0; i < files.length; i++ )
{
final String file = files[i];
if ( file != null && file.trim().length() > 0 )
{
final File ff = new File( file.trim() );
if ( ff.exists() )
{
final JwsModule.Descriptor dd = module.createDescriptor();
dd.setFile( ff );
}
}
}
}
}
/**
* Generate a logger by using the default settings.
*
* @param verbose set to true to enable more logging
* @return a default logger
*/
private static BuildListener getDefaultLogger( boolean verbose )
{
final DefaultLogger antLogger = new DefaultLogger();
antLogger.setOutputPrintStream( System.out );
antLogger.setErrorPrintStream( System.err );
antLogger.setMessageOutputLevel( verbose ? Project.MSG_DEBUG : Project.MSG_INFO );
return antLogger;
}
}
static class ClientGenParameters
implements Serializable
{
private static final long serialVersionUID = 1L;
private String classPath;
private boolean noWarn;
private File destDir;
private boolean verbose;
private boolean optimize;
private boolean debug;
private File srcDir;
private String destEncoding;
private String srcEncoding;
private boolean keepGenerated;
private File inputDir;
private String descriptors;
private String outtputName;
private boolean explode;
private String contextPath;
private String sourcePath;
private String wsType;
private String srcExcludes;
private String packageName;
private boolean useServerTypes;
private String serviceName;
private String handlerChainFile;
private String inputWSDL;
public String bindingFile;
public String getSourcePath()
{
return sourcePath;
}
public void setSourcePath( String sourcePath )
{
this.sourcePath = sourcePath;
}
public String getClassPath()
{
return classPath;
}
public void setClassPath( String classPath )
{
this.classPath = classPath;
}
public boolean isNoWarn()
{
return noWarn;
}
public void setNoWarn( boolean noWarn )
{
this.noWarn = noWarn;
}
public File getDestDir()
{
return destDir;
}
public void setDestDir( File destDir )
{
this.destDir = destDir;
}
public boolean isVerbose()
{
return verbose;
}
public void setVerbose( boolean verbose )
{
this.verbose = verbose;
}
public boolean isOptimize()
{
return optimize;
}
public void setOptimize( boolean optimize )
{
this.optimize = optimize;
}
public boolean isDebug()
{
return debug;
}
public void setDebug( boolean debug )
{
this.debug = debug;
}
public File getSrcDir()
{
return srcDir;
}
public void setSrcDir( File srcDir )
{
this.srcDir = srcDir;
}
public String getDestEncoding()
{
return destEncoding;
}
public void setDestEncoding( String destEncoding )
{
this.destEncoding = destEncoding;
}
public String getSrcEncoding()
{
return srcEncoding;
}
public void setSrcEncoding( String srcEncoding )
{
this.srcEncoding = srcEncoding;
}
public boolean isKeepGenerated()
{
return keepGenerated;
}
public void setKeepGenerated( boolean keepGenerated )
{
this.keepGenerated = keepGenerated;
}
public File getInputDir()
{
return inputDir;
}
public void setInputDir( File inputDir )
{
this.inputDir = inputDir;
}
public String getDescriptors()
{
return descriptors;
}
public void setDescriptors( String descriptors )
{
this.descriptors = descriptors;
}
public String getOuttputName()
{
return outtputName;
}
public void setOuttputName( String outtputName )
{
this.outtputName = outtputName;
}
public boolean isExplode()
{
return explode;
}
public void setExplode( boolean explode )
{
this.explode = explode;
}
public String getContextPath()
{
return contextPath;
}
public void setContextPath( String contextPath )
{
this.contextPath = contextPath;
}
public void setWsType( String wsType )
{
this.wsType = wsType;
}
public String getSrcExcludes()
{
return srcExcludes;
}
public void setSrcExcludes( String srcExcludes )
{
this.srcExcludes = srcExcludes;
}
public String getWsType()
{
return wsType;
}
@Override
public String toString()
{
final StringBuilder sb = new StringBuilder();
sb.append( "ClientGenParameters" );
sb.append( "{classPath='" ).append( classPath ).append( '\'' );
sb.append( ", noWarn=" ).append( noWarn );
sb.append( ", destDir=" ).append( destDir );
sb.append( ", verbose=" ).append( verbose );
sb.append( ", optimize=" ).append( optimize );
sb.append( ", debug=" ).append( debug );
sb.append( ", srcDir=" ).append( srcDir );
sb.append( ", destEncoding='" ).append( destEncoding ).append( '\'' );
sb.append( ", srcEncoding='" ).append( srcEncoding ).append( '\'' );
sb.append( ", keepGenerated=" ).append( keepGenerated );
sb.append( ", inputDir=" ).append( inputDir );
sb.append( ", descriptors='" ).append( descriptors ).append( '\'' );
sb.append( ", outtputName='" ).append( outtputName ).append( '\'' );
sb.append( ", explode=" ).append( explode );
sb.append( ", contextPath='" ).append( contextPath ).append( '\'' );
sb.append( ", sourcePath='" ).append( sourcePath ).append( '\'' );
sb.append( ", wsType='" ).append( wsType ).append( '\'' );
sb.append( ", srcExcludes='" ).append( srcExcludes ).append( '\'' );
sb.append( ", packageName='" ).append( packageName ).append( '\'' );
sb.append( ", useServerTypes=" ).append( useServerTypes );
sb.append( ", serviceName='" ).append( serviceName ).append( '\'' );
sb.append( ", handlerChainFile='" ).append( handlerChainFile ).append( '\'' );
sb.append( ", inputWSDL='" ).append( inputWSDL ).append( '\'' );
sb.append( ", bindingFile='" ).append( bindingFile ).append( '\'' );
sb.append( '}' );
return sb.toString();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy