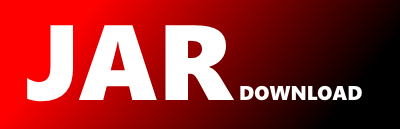
org.codehaus.mojo.weblogic.HelpMojo Maven / Gradle / Ivy
package org.codehaus.mojo.weblogic;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
import org.apache.maven.plugin.AbstractMojo;
import org.apache.maven.plugin.MojoExecutionException;
/**
* Display help information on weblogic-maven-plugin.
Call mvn weblogic:help -Ddetail=true -Dgoal=<goal-name>
to display parameter details.
*
* @version generated on Mon Sep 17 12:03:19 EDT 2012
* @author org.apache.maven.tools.plugin.generator.PluginHelpGenerator (version 2.7)
* @goal help
* @requiresProject false
* @threadSafe
*/
public class HelpMojo
extends AbstractMojo
{
/**
* If true
, display all settable properties for each goal.
*
* @parameter expression="${detail}" default-value="false"
*/
private boolean detail;
/**
* The name of the goal for which to show help. If unspecified, all goals will be displayed.
*
* @parameter expression="${goal}"
*/
private java.lang.String goal;
/**
* The maximum length of a display line, should be positive.
*
* @parameter expression="${lineLength}" default-value="80"
*/
private int lineLength;
/**
* The number of spaces per indentation level, should be positive.
*
* @parameter expression="${indentSize}" default-value="2"
*/
private int indentSize;
/** {@inheritDoc} */
public void execute()
throws MojoExecutionException
{
if ( lineLength <= 0 )
{
getLog().warn( "The parameter 'lineLength' should be positive, using '80' as default." );
lineLength = 80;
}
if ( indentSize <= 0 )
{
getLog().warn( "The parameter 'indentSize' should be positive, using '2' as default." );
indentSize = 2;
}
StringBuffer sb = new StringBuffer();
append( sb, "org.codehaus.mojo:weblogic-maven-plugin:2.9.5", 0 );
append( sb, "", 0 );
append( sb, "Mojo\'s Maven plugin for WebLogic", 0 );
append( sb, "This plugin will support various tasks within the Weblogic 8.1, 9.x, 10.x and 12.x environment. This version starts to support weblogic 10 and 11. The mojo supports such tasks as deploy, undeploy,clientgen,servicegen, and appc are supported as well as many others. The plugin uses exposed API\'s that are subject to change but have been tested in 8.1 SP 4-6 and 9.0 - 9.2 MP3, 10.x. There are two versions of the plugin to support the two environments based on differences in the JDK. The 9.x version is currently being refactored to support the standard JSR supported deployment interface. The code used in the plugin has been contributed to the Cargo project however to date has not be integrated into the codebase. Please feel free to suggest improvements or help support this plugin effort.", 1 );
append( sb, "", 0 );
if ( goal == null || goal.length() <= 0 )
{
append( sb, "This plugin has 12 goals:", 0 );
append( sb, "", 0 );
}
if ( goal == null || goal.length() <= 0 || "appc".equals( goal ) )
{
append( sb, "weblogic:appc", 0 );
append( sb, "Run the weblogic appc compiler against an artifact.", 1 );
append( sb, "", 0 );
if ( detail )
{
append( sb, "Available parameters:", 1 );
append( sb, "", 0 );
append( sb, "basicClientJar (Default: false)", 2 );
append( sb, "If set to true the basic client jar will be created without descriptors, etc.", 3 );
append( sb, "", 0 );
append( sb, "clientJarOutputDir (Default: ${project.build.directory})", 2 );
append( sb, "The full path to create the generated client jar file.", 3 );
append( sb, "", 0 );
append( sb, "continueCompilation (Default: true)", 2 );
append( sb, "If set to false the plugin stops after if compilation of a JSP file fails.", 3 );
append( sb, "", 0 );
append( sb, "forceGeneration (Default: true)", 2 );
append( sb, "If set to true the generation of JSP and EJB s will be forced to happen.", 3 );
append( sb, "", 0 );
append( sb, "inputArtifactPath", 2 );
append( sb, "The full path to the artifact to be compiled. It can be an EAR, War or Jar. If the project packaging is ejb then the .ejb suffix will be replaced with .jar if needed.", 3 );
append( sb, "Expression: ${project.build.directory}/${project.build.finalName}.${project.packaging}", 3 );
append( sb, "", 0 );
append( sb, "keepGenerated (Default: true)", 2 );
append( sb, "If set to true the generated source files will be kept.", 3 );
append( sb, "", 0 );
append( sb, "lineNumbers (Default: false)", 2 );
append( sb, "If set to true then line numbers will be added to classes for debugging.", 3 );
append( sb, "", 0 );
append( sb, "localRepository", 2 );
append( sb, "The local repository", 3 );
append( sb, "Expression: ${localRepository}", 3 );
append( sb, "", 0 );
append( sb, "outputArtifactPath", 2 );
append( sb, "The full path to the output artifact. By default it is not used and defaults to be the same as the input.", 3 );
append( sb, "", 0 );
append( sb, "outputDirectory", 2 );
append( sb, "This is the output directory for the artifacts. It defaults to $project.build.directory.", 3 );
append( sb, "Expression: ${project.build.directory}", 3 );
append( sb, "", 0 );
append( sb, "pluginArtifacts", 2 );
append( sb, "These are the plugin artifacts for the weblogic mojo", 3 );
append( sb, "Expression: ${plugin.artifacts}", 3 );
append( sb, "", 0 );
append( sb, "projectPackaging", 2 );
append( sb, "The project packaging used to check the suffix on the artifact.", 3 );
append( sb, "Expression: ${project.packaging}", 3 );
append( sb, "", 0 );
append( sb, "remoteRepositories", 2 );
append( sb, "The list of remote repositories", 3 );
append( sb, "Expression: ${project.remoteArtifactRepositories}", 3 );
append( sb, "", 0 );
append( sb, "toolsJar", 2 );
append( sb, "The the location of tools.jar file. this file must be dynamically added to the classloader due to some issues with the way maven works and a bug in jdk 1.6\nNote that the java.home location should be to the jre not the jdk", 3 );
append( sb, "Expression: ${java.home}/../lib/tools.jar", 3 );
append( sb, "", 0 );
append( sb, "verbose (Default: false)", 2 );
append( sb, "If this is set to true then verbose output for the process will be generated.", 3 );
append( sb, "", 0 );
append( sb, "weblogicHome (Default: ${weblogic.home})", 2 );
append( sb, "Property to set the weblogic home", 3 );
append( sb, "", 0 );
}
}
if ( goal == null || goal.length() <= 0 || "clientgen9".equals( goal ) )
{
append( sb, "weblogic:clientgen9", 0 );
append( sb, "Runs Client Gen on a given WSDL. This client gen uses the BEA refactored client gen tool first appearing in weblogic 9. This is the preferred client gen tool for Weblogic 9.0 and newer.", 1 );
append( sb, "", 0 );
if ( detail )
{
append( sb, "Available parameters:", 1 );
append( sb, "", 0 );
append( sb, "autotype (Default: true)", 2 );
append( sb, "Sets whether or not to create the type conversions for a web service in the client.", 3 );
append( sb, "", 0 );
append( sb, "bindingFile", 2 );
append( sb, "The binding files", 3 );
append( sb, "", 0 );
append( sb, "debug (Default: false)", 2 );
append( sb, "The debug setting", 3 );
append( sb, "", 0 );
append( sb, "generateAsyncMethods (Default: false)", 2 );
append( sb, "Sets whether or not to use the async method wrappers", 3 );
append( sb, "", 0 );
append( sb, "handlerChainFile", 2 );
append( sb, "The client handler chain configuration file.", 3 );
append( sb, "", 0 );
append( sb, "inputWSDL", 2 );
append( sb, "The wsdl to client gen from. If warFileName is specified, this parameter is the root relative file to use when creating the URI for the wsdl.", 3 );
append( sb, "", 0 );
append( sb, "jaxRPCWrappedArrayStyle (Default: true)", 2 );
append( sb, "Sets whether or not to use the jaxRPCWrappedArrayStyle", 3 );
append( sb, "", 0 );
append( sb, "localRepository", 2 );
append( sb, "The local repository", 3 );
append( sb, "Expression: ${localRepository}", 3 );
append( sb, "", 0 );
append( sb, "outputDir (Default: ${basedir}/src/main/java)", 2 );
append( sb, "The directory to output the generated code to.", 3 );
append( sb, "", 0 );
append( sb, "outputDirectory", 2 );
append( sb, "This is the output directory for the artifacts. It defaults to $project.build.directory.", 3 );
append( sb, "Expression: ${project.build.directory}", 3 );
append( sb, "", 0 );
append( sb, "packageName (Default: com.test.webservice)", 2 );
append( sb, "The package name of the output code.", 3 );
append( sb, "", 0 );
append( sb, "pluginArtifacts", 2 );
append( sb, "These are the plugin artifacts for the weblogic mojo", 3 );
append( sb, "Expression: ${plugin.artifacts}", 3 );
append( sb, "", 0 );
append( sb, "remoteRepositories", 2 );
append( sb, "The list of remote repositories", 3 );
append( sb, "Expression: ${project.remoteArtifactRepositories}", 3 );
append( sb, "", 0 );
append( sb, "serviceName", 2 );
append( sb, "The name of the service.", 3 );
append( sb, "", 0 );
append( sb, "skipClientGen (Default: false)", 2 );
append( sb, "Set this to skip JWSC compiling", 3 );
append( sb, "Expression: ${weblogic.clientgen.skip}", 3 );
append( sb, "", 0 );
append( sb, "toolsJar", 2 );
append( sb, "The the location of tools.jar file. this file must be dynamically added to the classloader due to some issues with the way maven works and a bug in jdk 1.6\nNote that the java.home location should be to the jre not the jdk", 3 );
append( sb, "Expression: ${java.home}/../lib/tools.jar", 3 );
append( sb, "", 0 );
append( sb, "useServerTypes (Default: false)", 2 );
append( sb, "Whether or not to use server types from the ear file in the client jar.", 3 );
append( sb, "", 0 );
append( sb, "verbose (Default: false)", 2 );
append( sb, "Output verbose messages", 3 );
append( sb, "", 0 );
append( sb, "warFileName", 2 );
append( sb, "The filename of the war file to find the services. The file path is extracted from the artifact list.", 3 );
append( sb, "", 0 );
append( sb, "weblogicHome (Default: ${weblogic.home})", 2 );
append( sb, "Property to set the weblogic home", 3 );
append( sb, "", 0 );
append( sb, "wsType (Default: JAXRPC)", 2 );
append( sb, "Set this to set the JWS tpe", 3 );
append( sb, "Expression: ${weblogic.jwsc.type}", 3 );
append( sb, "", 0 );
}
}
if ( goal == null || goal.length() <= 0 || "deploy".equals( goal ) )
{
append( sb, "weblogic:deploy", 0 );
append( sb, "Deploy an artifact to Weblogic servers(s) or cluster(s).", 1 );
append( sb, "", 0 );
if ( detail )
{
append( sb, "Available parameters:", 1 );
append( sb, "", 0 );
append( sb, "adminServerHostName (Default: localhost)", 2 );
append( sb, "The dns hostname of the Weblogic Admin server.", 3 );
append( sb, "Expression: ${weblogic.adminServer.hostName}", 3 );
append( sb, "", 0 );
append( sb, "adminServerPort (Default: 7001)", 2 );
append( sb, "The admin port of the Weblogic Admin Server.", 3 );
append( sb, "Expression: ${weblogic.adminServer.port}", 3 );
append( sb, "", 0 );
append( sb, "adminServerProtocol (Default: t3)", 2 );
append( sb, "The protocol to use to access the Weblogic Admin server for deployment.", 3 );
append( sb, "Expression: ${weblogic.adminServer.protocol}", 3 );
append( sb, "", 0 );
append( sb, "artifactPath", 2 );
append( sb, "The full path to artifact to be deployed.", 3 );
append( sb, "Expression: ${project.build.directory}/${project.build.finalName}", 3 );
append( sb, "", 0 );
append( sb, "continueOnFailure (Default: false)", 2 );
append( sb, "If set to true, the deployer will not stop if a failure is detected.", 3 );
append( sb, "", 0 );
append( sb, "customTrustKeyStoreFileName", 2 );
append( sb, "Set fully qualified name to the key store file", 3 );
append( sb, "Expression: ${weblogic.customTrustKeyStoreFileName}", 3 );
append( sb, "", 0 );
append( sb, "customTrustKeyStorePassPhrase", 2 );
append( sb, "Set passphrase for the keystore", 3 );
append( sb, "Expression: ${weblogic.customTrustKeyStorePassPhrase}", 3 );
append( sb, "", 0 );
append( sb, "customTrustKeyStoreType (Default: JKS)", 2 );
append( sb, "Set keystore type. Usually JKS", 3 );
append( sb, "Expression: ${weblogic.customTrustKeyStoreType}", 3 );
append( sb, "", 0 );
append( sb, "debug (Default: false)", 2 );
append( sb, "True to turn on debugging", 3 );
append( sb, "", 0 );
append( sb, "demoTrustKeyStore (Default: false)", 2 );
append( sb, "Set to true for the demo trust key store", 3 );
append( sb, "Expression: ${weblogic.demoTrustKeyStore}", 3 );
append( sb, "", 0 );
append( sb, "deployEjb (Default: false)", 2 );
append( sb, "The dns hostname of the Weblogic Admin server.", 3 );
append( sb, "Expression: ${weblogic.deployEjb}", 3 );
append( sb, "", 0 );
append( sb, "deploymentPlanPath", 2 );
append( sb, "Deploy the target with the referenced deployment plan", 3 );
append( sb, "Expression: ${weblogic.deploymentPlanPath}", 3 );
append( sb, "", 0 );
append( sb, "exploded (Default: false)", 2 );
append( sb, "Set to true to do exploded deployments", 3 );
append( sb, "Expression: ${weblogic.exploded}", 3 );
append( sb, "", 0 );
append( sb, "ignoreHostnameVerification (Default: true)", 2 );
append( sb, "Set to true for the demo trust key store", 3 );
append( sb, "Expression: ${weblogic.ignoreHostnameVerification}", 3 );
append( sb, "", 0 );
append( sb, "localRepository", 2 );
append( sb, "The local repository", 3 );
append( sb, "Expression: ${localRepository}", 3 );
append( sb, "", 0 );
append( sb, "name", 2 );
append( sb, "The name to use when deploying the object.", 3 );
append( sb, "Expression: ${project.artifactId}", 3 );
append( sb, "", 0 );
append( sb, "noExit (Default: false)", 2 );
append( sb, "tell weblogic to not exit if there is a deployment failure.", 3 );
append( sb, "", 0 );
append( sb, "noVersion (Default: false)", 2 );
append( sb, "Tell weblogic to ignore the versions an apply the task to all versions.", 3 );
append( sb, "", 0 );
append( sb, "noWait (Default: false)", 2 );
append( sb, "Set this value to true so weblogic will return immediately", 3 );
append( sb, "", 0 );
append( sb, "outputDirectory", 2 );
append( sb, "This is the output directory for the artifacts. It defaults to $project.build.directory.", 3 );
append( sb, "Expression: ${project.build.directory}", 3 );
append( sb, "", 0 );
append( sb, "password (Default: weblogic)", 2 );
append( sb, "The admin password to access the Weblogic Admin server for deployment.", 3 );
append( sb, "Expression: ${weblogic.password}", 3 );
append( sb, "", 0 );
append( sb, "pluginArtifacts", 2 );
append( sb, "These are the plugin artifacts for the weblogic mojo", 3 );
append( sb, "Expression: ${plugin.artifacts}", 3 );
append( sb, "", 0 );
append( sb, "projectPackaging", 2 );
append( sb, "The project packaging used to check the suffix on the artifact.", 3 );
append( sb, "Expression: ${project.packaging}", 3 );
append( sb, "", 0 );
append( sb, "remote (Default: false)", 2 );
append( sb, "True if you are running on a machine that is remote to the admin server. If this is a remote deployment and this is set to false then it is assumed that all source paths are valid paths on the admin server.", 3 );
append( sb, "Expression: ${weblogic.remote}", 3 );
append( sb, "", 0 );
append( sb, "remoteRepositories", 2 );
append( sb, "The list of remote repositories", 3 );
append( sb, "Expression: ${project.remoteArtifactRepositories}", 3 );
append( sb, "", 0 );
append( sb, "skip (Default: false)", 2 );
append( sb, "The dns hostname of the Weblogic Admin server.", 3 );
append( sb, "Expression: ${weblogic.deploy.skip}", 3 );
append( sb, "", 0 );
append( sb, "stage (Default: true)", 2 );
append( sb, "True if you want to turn off staging", 3 );
append( sb, "Expression: ${weblogic.stage}", 3 );
append( sb, "", 0 );
append( sb, "targetNames (Default: AdminServer)", 2 );
append( sb, "A comma seperated list of names of servers or clusters to deploy the artifact onto.", 3 );
append( sb, "Expression: ${weblogic.targetNames}", 3 );
append( sb, "", 0 );
append( sb, "timeout (Default: -1)", 2 );
append( sb, "Set this value to the timeout seconds. Set to <=0 to disable", 3 );
append( sb, "", 0 );
append( sb, "toolsJar", 2 );
append( sb, "The the location of tools.jar file. this file must be dynamically added to the classloader due to some issues with the way maven works and a bug in jdk 1.6\nNote that the java.home location should be to the jre not the jdk", 3 );
append( sb, "Expression: ${java.home}/../lib/tools.jar", 3 );
append( sb, "", 0 );
append( sb, "upload (Default: false)", 2 );
append( sb, "Set to true to upload the code.", 3 );
append( sb, "Expression: ${weblogic.upload}", 3 );
append( sb, "", 0 );
append( sb, "userConfigFile", 2 );
append( sb, "The user config file to use", 3 );
append( sb, "Expression: ${weblogic.userConfigFile}", 3 );
append( sb, "", 0 );
append( sb, "userId (Default: weblogic)", 2 );
append( sb, "The Admin UserId to access the Weblogic Admin server for deployment.", 3 );
append( sb, "Expression: ${weblogic.user}", 3 );
append( sb, "", 0 );
append( sb, "userKeyFile", 2 );
append( sb, "The default user key file to use", 3 );
append( sb, "Expression: ${weblogic.userKeyFile}", 3 );
append( sb, "", 0 );
append( sb, "verbose (Default: true)", 2 );
append( sb, "True to turn on debugging", 3 );
append( sb, "", 0 );
append( sb, "version (Default: false)", 2 );
append( sb, "Print the version for weblogic", 3 );
append( sb, "", 0 );
append( sb, "weblogicHome (Default: ${weblogic.home})", 2 );
append( sb, "Property to set the weblogic home", 3 );
append( sb, "", 0 );
}
}
if ( goal == null || goal.length() <= 0 || "help".equals( goal ) )
{
append( sb, "weblogic:help", 0 );
append( sb, "Display help information on weblogic-maven-plugin.\nCall\n\u00a0\u00a0mvn\u00a0weblogic:help\u00a0-Ddetail=true\u00a0-Dgoal=\nto display parameter details.", 1 );
append( sb, "", 0 );
if ( detail )
{
append( sb, "Available parameters:", 1 );
append( sb, "", 0 );
append( sb, "detail (Default: false)", 2 );
append( sb, "If true, display all settable properties for each goal.", 3 );
append( sb, "Expression: ${detail}", 3 );
append( sb, "", 0 );
append( sb, "goal", 2 );
append( sb, "The name of the goal for which to show help. If unspecified, all goals will be displayed.", 3 );
append( sb, "Expression: ${goal}", 3 );
append( sb, "", 0 );
append( sb, "indentSize (Default: 2)", 2 );
append( sb, "The number of spaces per indentation level, should be positive.", 3 );
append( sb, "Expression: ${indentSize}", 3 );
append( sb, "", 0 );
append( sb, "lineLength (Default: 80)", 2 );
append( sb, "The maximum length of a display line, should be positive.", 3 );
append( sb, "Expression: ${lineLength}", 3 );
append( sb, "", 0 );
}
}
if ( goal == null || goal.length() <= 0 || "jwsc".equals( goal ) )
{
append( sb, "weblogic:jwsc", 0 );
append( sb, "Runs the JWSC compiler task for web service enabled code.", 1 );
append( sb, "", 0 );
if ( detail )
{
append( sb, "Available parameters:", 1 );
append( sb, "", 0 );
append( sb, "contextPath", 2 );
append( sb, "The deployed context of the web service.", 3 );
append( sb, "Required: Yes", 3 );
append( sb, "", 0 );
append( sb, "debug (Default: false)", 2 );
append( sb, "The flag to set when debugging the process", 3 );
append( sb, "", 0 );
append( sb, "descriptor", 2 );
append( sb, "The web.xml descriptor to use if a new one should not be generated. The path should be fully qualified.\nIf there is more than one descriptor, use a comma \',\' separated list.\n", 3 );
append( sb, "", 0 );
append( sb, "explode (Default: true)", 2 );
append( sb, "The flag to set when wanting exploded output. Defaults to true.", 3 );
append( sb, "", 0 );
append( sb, "inputDir (Default: ${basedir}/src/main/java)", 2 );
append( sb, "The directory to find the jwsc enabled files.", 3 );
append( sb, "", 0 );
append( sb, "jwscTaskType (Default: JAXRPC)", 2 );
append( sb, "The task type JAXRPC JAXWS", 3 );
append( sb, "Expression: ${weblogic.jwsc.type}", 3 );
append( sb, "", 0 );
append( sb, "keepGenerated (Default: false)", 2 );
append( sb, "The keep generated flag for the mojo", 3 );
append( sb, "Expression: ${weblogic.jwsc.keepGenerated}", 3 );
append( sb, "", 0 );
append( sb, "localRepository", 2 );
append( sb, "The local repository", 3 );
append( sb, "Expression: ${localRepository}", 3 );
append( sb, "", 0 );
append( sb, "optimize (Default: true)", 2 );
append( sb, "The flag to set when requiring optimization. Defaults to true.", 3 );
append( sb, "", 0 );
append( sb, "outputDir (Default: ${project.build.directory})", 2 );
append( sb, "The directory to output the generated code to.", 3 );
append( sb, "", 0 );
append( sb, "outputDirectory", 2 );
append( sb, "This is the output directory for the artifacts. It defaults to $project.build.directory.", 3 );
append( sb, "Expression: ${project.build.directory}", 3 );
append( sb, "", 0 );
append( sb, "outputName (Default: ${project.artifactId}-${project.version})", 2 );
append( sb, "The name of the war to use when evaluating the ear file.", 3 );
append( sb, "Required: Yes", 3 );
append( sb, "", 0 );
append( sb, "pluginArtifacts", 2 );
append( sb, "These are the plugin artifacts for the weblogic mojo", 3 );
append( sb, "Expression: ${plugin.artifacts}", 3 );
append( sb, "", 0 );
append( sb, "remoteRepositories", 2 );
append( sb, "The list of remote repositories", 3 );
append( sb, "Expression: ${project.remoteArtifactRepositories}", 3 );
append( sb, "", 0 );
append( sb, "sourceExcludes", 2 );
append( sb, "The explicit includes list for the file set", 3 );
append( sb, "Expression: ${weblogic.jwsc.excludes}", 3 );
append( sb, "", 0 );
append( sb, "sourceIncludes (Default: **/*.java)", 2 );
append( sb, "The explicit includes list for the file set", 3 );
append( sb, "Expression: ${weblogic.jwsc.includes}", 3 );
append( sb, "", 0 );
append( sb, "toolsJar", 2 );
append( sb, "The the location of tools.jar file. this file must be dynamically added to the classloader due to some issues with the way maven works and a bug in jdk 1.6\nNote that the java.home location should be to the jre not the jdk", 3 );
append( sb, "Expression: ${java.home}/../lib/tools.jar", 3 );
append( sb, "", 0 );
append( sb, "verbose (Default: false)", 2 );
append( sb, "The flag to set when desiring verbose output", 3 );
append( sb, "", 0 );
append( sb, "weblogicHome (Default: ${weblogic.home})", 2 );
append( sb, "Property to set the weblogic home", 3 );
append( sb, "", 0 );
}
}
if ( goal == null || goal.length() <= 0 || "listapps".equals( goal ) )
{
append( sb, "weblogic:listapps", 0 );
append( sb, "List the atifacts on Weblogic server(s) or cluster(s).", 1 );
append( sb, "", 0 );
if ( detail )
{
append( sb, "Available parameters:", 1 );
append( sb, "", 0 );
append( sb, "adminServerHostName (Default: localhost)", 2 );
append( sb, "The dns hostname of the Weblogic Admin server.", 3 );
append( sb, "Expression: ${weblogic.adminServer.hostName}", 3 );
append( sb, "", 0 );
append( sb, "adminServerPort (Default: 7001)", 2 );
append( sb, "The admin port of the Weblogic Admin Server.", 3 );
append( sb, "Expression: ${weblogic.adminServer.port}", 3 );
append( sb, "", 0 );
append( sb, "adminServerProtocol (Default: t3)", 2 );
append( sb, "The protocol to use to access the Weblogic Admin server for deployment.", 3 );
append( sb, "Expression: ${weblogic.adminServer.protocol}", 3 );
append( sb, "", 0 );
append( sb, "artifactPath", 2 );
append( sb, "The full path to artifact to be deployed.", 3 );
append( sb, "Expression: ${project.build.directory}/${project.build.finalName}", 3 );
append( sb, "", 0 );
append( sb, "continueOnFailure (Default: false)", 2 );
append( sb, "If set to true, the deployer will not stop if a failure is detected.", 3 );
append( sb, "", 0 );
append( sb, "customTrustKeyStoreFileName", 2 );
append( sb, "Set fully qualified name to the key store file", 3 );
append( sb, "Expression: ${weblogic.customTrustKeyStoreFileName}", 3 );
append( sb, "", 0 );
append( sb, "customTrustKeyStorePassPhrase", 2 );
append( sb, "Set passphrase for the keystore", 3 );
append( sb, "Expression: ${weblogic.customTrustKeyStorePassPhrase}", 3 );
append( sb, "", 0 );
append( sb, "customTrustKeyStoreType (Default: JKS)", 2 );
append( sb, "Set keystore type. Usually JKS", 3 );
append( sb, "Expression: ${weblogic.customTrustKeyStoreType}", 3 );
append( sb, "", 0 );
append( sb, "debug (Default: false)", 2 );
append( sb, "True to turn on debugging", 3 );
append( sb, "", 0 );
append( sb, "demoTrustKeyStore (Default: false)", 2 );
append( sb, "Set to true for the demo trust key store", 3 );
append( sb, "Expression: ${weblogic.demoTrustKeyStore}", 3 );
append( sb, "", 0 );
append( sb, "deployEjb (Default: false)", 2 );
append( sb, "The dns hostname of the Weblogic Admin server.", 3 );
append( sb, "Expression: ${weblogic.deployEjb}", 3 );
append( sb, "", 0 );
append( sb, "deploymentPlanPath", 2 );
append( sb, "Deploy the target with the referenced deployment plan", 3 );
append( sb, "Expression: ${weblogic.deploymentPlanPath}", 3 );
append( sb, "", 0 );
append( sb, "exploded (Default: false)", 2 );
append( sb, "Set to true to do exploded deployments", 3 );
append( sb, "Expression: ${weblogic.exploded}", 3 );
append( sb, "", 0 );
append( sb, "ignoreHostnameVerification (Default: true)", 2 );
append( sb, "Set to true for the demo trust key store", 3 );
append( sb, "Expression: ${weblogic.ignoreHostnameVerification}", 3 );
append( sb, "", 0 );
append( sb, "localRepository", 2 );
append( sb, "The local repository", 3 );
append( sb, "Expression: ${localRepository}", 3 );
append( sb, "", 0 );
append( sb, "name", 2 );
append( sb, "The name to use when deploying the object.", 3 );
append( sb, "Expression: ${project.artifactId}", 3 );
append( sb, "", 0 );
append( sb, "noExit (Default: false)", 2 );
append( sb, "tell weblogic to not exit if there is a deployment failure.", 3 );
append( sb, "", 0 );
append( sb, "noVersion (Default: false)", 2 );
append( sb, "Tell weblogic to ignore the versions an apply the task to all versions.", 3 );
append( sb, "", 0 );
append( sb, "noWait (Default: false)", 2 );
append( sb, "Set this value to true so weblogic will return immediately", 3 );
append( sb, "", 0 );
append( sb, "outputDirectory", 2 );
append( sb, "This is the output directory for the artifacts. It defaults to $project.build.directory.", 3 );
append( sb, "Expression: ${project.build.directory}", 3 );
append( sb, "", 0 );
append( sb, "password (Default: weblogic)", 2 );
append( sb, "The admin password to access the Weblogic Admin server for deployment.", 3 );
append( sb, "Expression: ${weblogic.password}", 3 );
append( sb, "", 0 );
append( sb, "pluginArtifacts", 2 );
append( sb, "These are the plugin artifacts for the weblogic mojo", 3 );
append( sb, "Expression: ${plugin.artifacts}", 3 );
append( sb, "", 0 );
append( sb, "projectPackaging", 2 );
append( sb, "The project packaging used to check the suffix on the artifact.", 3 );
append( sb, "Expression: ${project.packaging}", 3 );
append( sb, "", 0 );
append( sb, "remote (Default: false)", 2 );
append( sb, "True if you are running on a machine that is remote to the admin server. If this is a remote deployment and this is set to false then it is assumed that all source paths are valid paths on the admin server.", 3 );
append( sb, "Expression: ${weblogic.remote}", 3 );
append( sb, "", 0 );
append( sb, "remoteRepositories", 2 );
append( sb, "The list of remote repositories", 3 );
append( sb, "Expression: ${project.remoteArtifactRepositories}", 3 );
append( sb, "", 0 );
append( sb, "skip (Default: false)", 2 );
append( sb, "The dns hostname of the Weblogic Admin server.", 3 );
append( sb, "Expression: ${weblogic.deploy.skip}", 3 );
append( sb, "", 0 );
append( sb, "stage (Default: true)", 2 );
append( sb, "True if you want to turn off staging", 3 );
append( sb, "Expression: ${weblogic.stage}", 3 );
append( sb, "", 0 );
append( sb, "targetNames (Default: AdminServer)", 2 );
append( sb, "A comma seperated list of names of servers or clusters to deploy the artifact onto.", 3 );
append( sb, "Expression: ${weblogic.targetNames}", 3 );
append( sb, "", 0 );
append( sb, "timeout (Default: -1)", 2 );
append( sb, "Set this value to the timeout seconds. Set to <=0 to disable", 3 );
append( sb, "", 0 );
append( sb, "toolsJar", 2 );
append( sb, "The the location of tools.jar file. this file must be dynamically added to the classloader due to some issues with the way maven works and a bug in jdk 1.6\nNote that the java.home location should be to the jre not the jdk", 3 );
append( sb, "Expression: ${java.home}/../lib/tools.jar", 3 );
append( sb, "", 0 );
append( sb, "upload (Default: false)", 2 );
append( sb, "Set to true to upload the code.", 3 );
append( sb, "Expression: ${weblogic.upload}", 3 );
append( sb, "", 0 );
append( sb, "userConfigFile", 2 );
append( sb, "The user config file to use", 3 );
append( sb, "Expression: ${weblogic.userConfigFile}", 3 );
append( sb, "", 0 );
append( sb, "userId (Default: weblogic)", 2 );
append( sb, "The Admin UserId to access the Weblogic Admin server for deployment.", 3 );
append( sb, "Expression: ${weblogic.user}", 3 );
append( sb, "", 0 );
append( sb, "userKeyFile", 2 );
append( sb, "The default user key file to use", 3 );
append( sb, "Expression: ${weblogic.userKeyFile}", 3 );
append( sb, "", 0 );
append( sb, "verbose (Default: true)", 2 );
append( sb, "True to turn on debugging", 3 );
append( sb, "", 0 );
append( sb, "version (Default: false)", 2 );
append( sb, "Print the version for weblogic", 3 );
append( sb, "", 0 );
append( sb, "weblogicHome (Default: ${weblogic.home})", 2 );
append( sb, "Property to set the weblogic home", 3 );
append( sb, "", 0 );
}
}
if ( goal == null || goal.length() <= 0 || "redeploy".equals( goal ) )
{
append( sb, "weblogic:redeploy", 0 );
append( sb, "Redeploy artifact on Weblogic server(s) or cluster(s).", 1 );
append( sb, "", 0 );
if ( detail )
{
append( sb, "Available parameters:", 1 );
append( sb, "", 0 );
append( sb, "adminServerHostName (Default: localhost)", 2 );
append( sb, "The dns hostname of the Weblogic Admin server.", 3 );
append( sb, "Expression: ${weblogic.adminServer.hostName}", 3 );
append( sb, "", 0 );
append( sb, "adminServerPort (Default: 7001)", 2 );
append( sb, "The admin port of the Weblogic Admin Server.", 3 );
append( sb, "Expression: ${weblogic.adminServer.port}", 3 );
append( sb, "", 0 );
append( sb, "adminServerProtocol (Default: t3)", 2 );
append( sb, "The protocol to use to access the Weblogic Admin server for deployment.", 3 );
append( sb, "Expression: ${weblogic.adminServer.protocol}", 3 );
append( sb, "", 0 );
append( sb, "artifactPath", 2 );
append( sb, "The full path to artifact to be deployed.", 3 );
append( sb, "Expression: ${project.build.directory}/${project.build.finalName}", 3 );
append( sb, "", 0 );
append( sb, "continueOnFailure (Default: false)", 2 );
append( sb, "If set to true, the deployer will not stop if a failure is detected.", 3 );
append( sb, "", 0 );
append( sb, "customTrustKeyStoreFileName", 2 );
append( sb, "Set fully qualified name to the key store file", 3 );
append( sb, "Expression: ${weblogic.customTrustKeyStoreFileName}", 3 );
append( sb, "", 0 );
append( sb, "customTrustKeyStorePassPhrase", 2 );
append( sb, "Set passphrase for the keystore", 3 );
append( sb, "Expression: ${weblogic.customTrustKeyStorePassPhrase}", 3 );
append( sb, "", 0 );
append( sb, "customTrustKeyStoreType (Default: JKS)", 2 );
append( sb, "Set keystore type. Usually JKS", 3 );
append( sb, "Expression: ${weblogic.customTrustKeyStoreType}", 3 );
append( sb, "", 0 );
append( sb, "debug (Default: false)", 2 );
append( sb, "True to turn on debugging", 3 );
append( sb, "", 0 );
append( sb, "demoTrustKeyStore (Default: false)", 2 );
append( sb, "Set to true for the demo trust key store", 3 );
append( sb, "Expression: ${weblogic.demoTrustKeyStore}", 3 );
append( sb, "", 0 );
append( sb, "deployEjb (Default: false)", 2 );
append( sb, "The dns hostname of the Weblogic Admin server.", 3 );
append( sb, "Expression: ${weblogic.deployEjb}", 3 );
append( sb, "", 0 );
append( sb, "deploymentPlanPath", 2 );
append( sb, "Deploy the target with the referenced deployment plan", 3 );
append( sb, "Expression: ${weblogic.deploymentPlanPath}", 3 );
append( sb, "", 0 );
append( sb, "exploded (Default: false)", 2 );
append( sb, "Set to true to do exploded deployments", 3 );
append( sb, "Expression: ${weblogic.exploded}", 3 );
append( sb, "", 0 );
append( sb, "ignoreHostnameVerification (Default: true)", 2 );
append( sb, "Set to true for the demo trust key store", 3 );
append( sb, "Expression: ${weblogic.ignoreHostnameVerification}", 3 );
append( sb, "", 0 );
append( sb, "localRepository", 2 );
append( sb, "The local repository", 3 );
append( sb, "Expression: ${localRepository}", 3 );
append( sb, "", 0 );
append( sb, "name", 2 );
append( sb, "The name to use when deploying the object.", 3 );
append( sb, "Expression: ${project.artifactId}", 3 );
append( sb, "", 0 );
append( sb, "noExit (Default: false)", 2 );
append( sb, "tell weblogic to not exit if there is a deployment failure.", 3 );
append( sb, "", 0 );
append( sb, "noVersion (Default: false)", 2 );
append( sb, "Tell weblogic to ignore the versions an apply the task to all versions.", 3 );
append( sb, "", 0 );
append( sb, "noWait (Default: false)", 2 );
append( sb, "Set this value to true so weblogic will return immediately", 3 );
append( sb, "", 0 );
append( sb, "outputDirectory", 2 );
append( sb, "This is the output directory for the artifacts. It defaults to $project.build.directory.", 3 );
append( sb, "Expression: ${project.build.directory}", 3 );
append( sb, "", 0 );
append( sb, "password (Default: weblogic)", 2 );
append( sb, "The admin password to access the Weblogic Admin server for deployment.", 3 );
append( sb, "Expression: ${weblogic.password}", 3 );
append( sb, "", 0 );
append( sb, "pluginArtifacts", 2 );
append( sb, "These are the plugin artifacts for the weblogic mojo", 3 );
append( sb, "Expression: ${plugin.artifacts}", 3 );
append( sb, "", 0 );
append( sb, "projectPackaging", 2 );
append( sb, "The project packaging used to check the suffix on the artifact.", 3 );
append( sb, "Expression: ${project.packaging}", 3 );
append( sb, "", 0 );
append( sb, "remote (Default: false)", 2 );
append( sb, "True if you are running on a machine that is remote to the admin server. If this is a remote deployment and this is set to false then it is assumed that all source paths are valid paths on the admin server.", 3 );
append( sb, "Expression: ${weblogic.remote}", 3 );
append( sb, "", 0 );
append( sb, "remoteRepositories", 2 );
append( sb, "The list of remote repositories", 3 );
append( sb, "Expression: ${project.remoteArtifactRepositories}", 3 );
append( sb, "", 0 );
append( sb, "skip (Default: false)", 2 );
append( sb, "The dns hostname of the Weblogic Admin server.", 3 );
append( sb, "Expression: ${weblogic.deploy.skip}", 3 );
append( sb, "", 0 );
append( sb, "stage (Default: true)", 2 );
append( sb, "True if you want to turn off staging", 3 );
append( sb, "Expression: ${weblogic.stage}", 3 );
append( sb, "", 0 );
append( sb, "targetNames (Default: AdminServer)", 2 );
append( sb, "A comma seperated list of names of servers or clusters to deploy the artifact onto.", 3 );
append( sb, "Expression: ${weblogic.targetNames}", 3 );
append( sb, "", 0 );
append( sb, "timeout (Default: -1)", 2 );
append( sb, "Set this value to the timeout seconds. Set to <=0 to disable", 3 );
append( sb, "", 0 );
append( sb, "toolsJar", 2 );
append( sb, "The the location of tools.jar file. this file must be dynamically added to the classloader due to some issues with the way maven works and a bug in jdk 1.6\nNote that the java.home location should be to the jre not the jdk", 3 );
append( sb, "Expression: ${java.home}/../lib/tools.jar", 3 );
append( sb, "", 0 );
append( sb, "upload (Default: false)", 2 );
append( sb, "Set to true to upload the code.", 3 );
append( sb, "Expression: ${weblogic.upload}", 3 );
append( sb, "", 0 );
append( sb, "userConfigFile", 2 );
append( sb, "The user config file to use", 3 );
append( sb, "Expression: ${weblogic.userConfigFile}", 3 );
append( sb, "", 0 );
append( sb, "userId (Default: weblogic)", 2 );
append( sb, "The Admin UserId to access the Weblogic Admin server for deployment.", 3 );
append( sb, "Expression: ${weblogic.user}", 3 );
append( sb, "", 0 );
append( sb, "userKeyFile", 2 );
append( sb, "The default user key file to use", 3 );
append( sb, "Expression: ${weblogic.userKeyFile}", 3 );
append( sb, "", 0 );
append( sb, "verbose (Default: true)", 2 );
append( sb, "True to turn on debugging", 3 );
append( sb, "", 0 );
append( sb, "version (Default: false)", 2 );
append( sb, "Print the version for weblogic", 3 );
append( sb, "", 0 );
append( sb, "weblogicHome (Default: ${weblogic.home})", 2 );
append( sb, "Property to set the weblogic home", 3 );
append( sb, "", 0 );
}
}
if ( goal == null || goal.length() <= 0 || "servicegen".equals( goal ) )
{
append( sb, "weblogic:servicegen", 0 );
append( sb, "Runs Service Gen on a given WSDL.", 1 );
append( sb, "", 0 );
if ( detail )
{
append( sb, "Available parameters:", 1 );
append( sb, "", 0 );
append( sb, "contextUri", 2 );
append( sb, "The context uri for the service", 3 );
append( sb, "Required: Yes", 3 );
append( sb, "", 0 );
append( sb, "inputArtifactPath", 2 );
append( sb, "The full path to the artifact to be compiled. It can be an EAR, War or Jar. If the project packaging is ejb then the .ejb suffix will be replaced with .jar if needed.", 3 );
append( sb, "Expression: ${project.build.directory}/${project.build.finalName}.${project.packaging}", 3 );
append( sb, "", 0 );
append( sb, "localRepository", 2 );
append( sb, "The local repository", 3 );
append( sb, "Expression: ${localRepository}", 3 );
append( sb, "", 0 );
append( sb, "outputDir (Default: ${basedir}/src/main/java)", 2 );
append( sb, "The directory to output the geneated code to.", 3 );
append( sb, "", 0 );
append( sb, "outputDirectory", 2 );
append( sb, "This is the output directory for the artifacts. It defaults to $project.build.directory.", 3 );
append( sb, "Expression: ${project.build.directory}", 3 );
append( sb, "", 0 );
append( sb, "packageName (Default: com.test.webservice)", 2 );
append( sb, "The package name of the output code.", 3 );
append( sb, "", 0 );
append( sb, "pluginArtifacts", 2 );
append( sb, "These are the plugin artifacts for the weblogic mojo", 3 );
append( sb, "Expression: ${plugin.artifacts}", 3 );
append( sb, "", 0 );
append( sb, "remoteRepositories", 2 );
append( sb, "The list of remote repositories", 3 );
append( sb, "Expression: ${project.remoteArtifactRepositories}", 3 );
append( sb, "", 0 );
append( sb, "services", 2 );
append( sb, "The service configurations to generate", 3 );
append( sb, "", 0 );
append( sb, "toolsJar", 2 );
append( sb, "The the location of tools.jar file. this file must be dynamically added to the classloader due to some issues with the way maven works and a bug in jdk 1.6\nNote that the java.home location should be to the jre not the jdk", 3 );
append( sb, "Expression: ${java.home}/../lib/tools.jar", 3 );
append( sb, "", 0 );
append( sb, "warName", 2 );
append( sb, "this is the name of the war to execute the service gen against.", 3 );
append( sb, "Required: Yes", 3 );
append( sb, "", 0 );
append( sb, "weblogicHome (Default: ${weblogic.home})", 2 );
append( sb, "Property to set the weblogic home", 3 );
append( sb, "", 0 );
}
}
if ( goal == null || goal.length() <= 0 || "start".equals( goal ) )
{
append( sb, "weblogic:start", 0 );
append( sb, "Start an artifact on Weblogic server(s) or cluster(s).", 1 );
append( sb, "", 0 );
if ( detail )
{
append( sb, "Available parameters:", 1 );
append( sb, "", 0 );
append( sb, "adminServerHostName (Default: localhost)", 2 );
append( sb, "The dns hostname of the Weblogic Admin server.", 3 );
append( sb, "Expression: ${weblogic.adminServer.hostName}", 3 );
append( sb, "", 0 );
append( sb, "adminServerPort (Default: 7001)", 2 );
append( sb, "The admin port of the Weblogic Admin Server.", 3 );
append( sb, "Expression: ${weblogic.adminServer.port}", 3 );
append( sb, "", 0 );
append( sb, "adminServerProtocol (Default: t3)", 2 );
append( sb, "The protocol to use to access the Weblogic Admin server for deployment.", 3 );
append( sb, "Expression: ${weblogic.adminServer.protocol}", 3 );
append( sb, "", 0 );
append( sb, "artifactPath", 2 );
append( sb, "The full path to artifact to be deployed.", 3 );
append( sb, "Expression: ${project.build.directory}/${project.build.finalName}", 3 );
append( sb, "", 0 );
append( sb, "continueOnFailure (Default: false)", 2 );
append( sb, "If set to true, the deployer will not stop if a failure is detected.", 3 );
append( sb, "", 0 );
append( sb, "customTrustKeyStoreFileName", 2 );
append( sb, "Set fully qualified name to the key store file", 3 );
append( sb, "Expression: ${weblogic.customTrustKeyStoreFileName}", 3 );
append( sb, "", 0 );
append( sb, "customTrustKeyStorePassPhrase", 2 );
append( sb, "Set passphrase for the keystore", 3 );
append( sb, "Expression: ${weblogic.customTrustKeyStorePassPhrase}", 3 );
append( sb, "", 0 );
append( sb, "customTrustKeyStoreType (Default: JKS)", 2 );
append( sb, "Set keystore type. Usually JKS", 3 );
append( sb, "Expression: ${weblogic.customTrustKeyStoreType}", 3 );
append( sb, "", 0 );
append( sb, "debug (Default: false)", 2 );
append( sb, "True to turn on debugging", 3 );
append( sb, "", 0 );
append( sb, "demoTrustKeyStore (Default: false)", 2 );
append( sb, "Set to true for the demo trust key store", 3 );
append( sb, "Expression: ${weblogic.demoTrustKeyStore}", 3 );
append( sb, "", 0 );
append( sb, "deployEjb (Default: false)", 2 );
append( sb, "The dns hostname of the Weblogic Admin server.", 3 );
append( sb, "Expression: ${weblogic.deployEjb}", 3 );
append( sb, "", 0 );
append( sb, "deploymentPlanPath", 2 );
append( sb, "Deploy the target with the referenced deployment plan", 3 );
append( sb, "Expression: ${weblogic.deploymentPlanPath}", 3 );
append( sb, "", 0 );
append( sb, "exploded (Default: false)", 2 );
append( sb, "Set to true to do exploded deployments", 3 );
append( sb, "Expression: ${weblogic.exploded}", 3 );
append( sb, "", 0 );
append( sb, "ignoreHostnameVerification (Default: true)", 2 );
append( sb, "Set to true for the demo trust key store", 3 );
append( sb, "Expression: ${weblogic.ignoreHostnameVerification}", 3 );
append( sb, "", 0 );
append( sb, "localRepository", 2 );
append( sb, "The local repository", 3 );
append( sb, "Expression: ${localRepository}", 3 );
append( sb, "", 0 );
append( sb, "name", 2 );
append( sb, "The name to use when deploying the object.", 3 );
append( sb, "Expression: ${project.artifactId}", 3 );
append( sb, "", 0 );
append( sb, "noExit (Default: false)", 2 );
append( sb, "tell weblogic to not exit if there is a deployment failure.", 3 );
append( sb, "", 0 );
append( sb, "noVersion (Default: false)", 2 );
append( sb, "Tell weblogic to ignore the versions an apply the task to all versions.", 3 );
append( sb, "", 0 );
append( sb, "noWait (Default: false)", 2 );
append( sb, "Set this value to true so weblogic will return immediately", 3 );
append( sb, "", 0 );
append( sb, "outputDirectory", 2 );
append( sb, "This is the output directory for the artifacts. It defaults to $project.build.directory.", 3 );
append( sb, "Expression: ${project.build.directory}", 3 );
append( sb, "", 0 );
append( sb, "password (Default: weblogic)", 2 );
append( sb, "The admin password to access the Weblogic Admin server for deployment.", 3 );
append( sb, "Expression: ${weblogic.password}", 3 );
append( sb, "", 0 );
append( sb, "pluginArtifacts", 2 );
append( sb, "These are the plugin artifacts for the weblogic mojo", 3 );
append( sb, "Expression: ${plugin.artifacts}", 3 );
append( sb, "", 0 );
append( sb, "projectPackaging", 2 );
append( sb, "The project packaging used to check the suffix on the artifact.", 3 );
append( sb, "Expression: ${project.packaging}", 3 );
append( sb, "", 0 );
append( sb, "remote (Default: false)", 2 );
append( sb, "True if you are running on a machine that is remote to the admin server. If this is a remote deployment and this is set to false then it is assumed that all source paths are valid paths on the admin server.", 3 );
append( sb, "Expression: ${weblogic.remote}", 3 );
append( sb, "", 0 );
append( sb, "remoteRepositories", 2 );
append( sb, "The list of remote repositories", 3 );
append( sb, "Expression: ${project.remoteArtifactRepositories}", 3 );
append( sb, "", 0 );
append( sb, "skip (Default: false)", 2 );
append( sb, "The dns hostname of the Weblogic Admin server.", 3 );
append( sb, "Expression: ${weblogic.deploy.skip}", 3 );
append( sb, "", 0 );
append( sb, "stage (Default: true)", 2 );
append( sb, "True if you want to turn off staging", 3 );
append( sb, "Expression: ${weblogic.stage}", 3 );
append( sb, "", 0 );
append( sb, "targetNames (Default: AdminServer)", 2 );
append( sb, "A comma seperated list of names of servers or clusters to deploy the artifact onto.", 3 );
append( sb, "Expression: ${weblogic.targetNames}", 3 );
append( sb, "", 0 );
append( sb, "timeout (Default: -1)", 2 );
append( sb, "Set this value to the timeout seconds. Set to <=0 to disable", 3 );
append( sb, "", 0 );
append( sb, "toolsJar", 2 );
append( sb, "The the location of tools.jar file. this file must be dynamically added to the classloader due to some issues with the way maven works and a bug in jdk 1.6\nNote that the java.home location should be to the jre not the jdk", 3 );
append( sb, "Expression: ${java.home}/../lib/tools.jar", 3 );
append( sb, "", 0 );
append( sb, "upload (Default: false)", 2 );
append( sb, "Set to true to upload the code.", 3 );
append( sb, "Expression: ${weblogic.upload}", 3 );
append( sb, "", 0 );
append( sb, "userConfigFile", 2 );
append( sb, "The user config file to use", 3 );
append( sb, "Expression: ${weblogic.userConfigFile}", 3 );
append( sb, "", 0 );
append( sb, "userId (Default: weblogic)", 2 );
append( sb, "The Admin UserId to access the Weblogic Admin server for deployment.", 3 );
append( sb, "Expression: ${weblogic.user}", 3 );
append( sb, "", 0 );
append( sb, "userKeyFile", 2 );
append( sb, "The default user key file to use", 3 );
append( sb, "Expression: ${weblogic.userKeyFile}", 3 );
append( sb, "", 0 );
append( sb, "verbose (Default: true)", 2 );
append( sb, "True to turn on debugging", 3 );
append( sb, "", 0 );
append( sb, "version (Default: false)", 2 );
append( sb, "Print the version for weblogic", 3 );
append( sb, "", 0 );
append( sb, "weblogicHome (Default: ${weblogic.home})", 2 );
append( sb, "Property to set the weblogic home", 3 );
append( sb, "", 0 );
}
}
if ( goal == null || goal.length() <= 0 || "stop".equals( goal ) )
{
append( sb, "weblogic:stop", 0 );
append( sb, "Stop an artifact on Weblogic server(s) or cluster(s).", 1 );
append( sb, "", 0 );
if ( detail )
{
append( sb, "Available parameters:", 1 );
append( sb, "", 0 );
append( sb, "adminServerHostName (Default: localhost)", 2 );
append( sb, "The dns hostname of the Weblogic Admin server.", 3 );
append( sb, "Expression: ${weblogic.adminServer.hostName}", 3 );
append( sb, "", 0 );
append( sb, "adminServerPort (Default: 7001)", 2 );
append( sb, "The admin port of the Weblogic Admin Server.", 3 );
append( sb, "Expression: ${weblogic.adminServer.port}", 3 );
append( sb, "", 0 );
append( sb, "adminServerProtocol (Default: t3)", 2 );
append( sb, "The protocol to use to access the Weblogic Admin server for deployment.", 3 );
append( sb, "Expression: ${weblogic.adminServer.protocol}", 3 );
append( sb, "", 0 );
append( sb, "artifactPath", 2 );
append( sb, "The full path to artifact to be deployed.", 3 );
append( sb, "Expression: ${project.build.directory}/${project.build.finalName}", 3 );
append( sb, "", 0 );
append( sb, "continueOnFailure (Default: false)", 2 );
append( sb, "If set to true, the deployer will not stop if a failure is detected.", 3 );
append( sb, "", 0 );
append( sb, "customTrustKeyStoreFileName", 2 );
append( sb, "Set fully qualified name to the key store file", 3 );
append( sb, "Expression: ${weblogic.customTrustKeyStoreFileName}", 3 );
append( sb, "", 0 );
append( sb, "customTrustKeyStorePassPhrase", 2 );
append( sb, "Set passphrase for the keystore", 3 );
append( sb, "Expression: ${weblogic.customTrustKeyStorePassPhrase}", 3 );
append( sb, "", 0 );
append( sb, "customTrustKeyStoreType (Default: JKS)", 2 );
append( sb, "Set keystore type. Usually JKS", 3 );
append( sb, "Expression: ${weblogic.customTrustKeyStoreType}", 3 );
append( sb, "", 0 );
append( sb, "debug (Default: false)", 2 );
append( sb, "True to turn on debugging", 3 );
append( sb, "", 0 );
append( sb, "demoTrustKeyStore (Default: false)", 2 );
append( sb, "Set to true for the demo trust key store", 3 );
append( sb, "Expression: ${weblogic.demoTrustKeyStore}", 3 );
append( sb, "", 0 );
append( sb, "deployEjb (Default: false)", 2 );
append( sb, "The dns hostname of the Weblogic Admin server.", 3 );
append( sb, "Expression: ${weblogic.deployEjb}", 3 );
append( sb, "", 0 );
append( sb, "deploymentPlanPath", 2 );
append( sb, "Deploy the target with the referenced deployment plan", 3 );
append( sb, "Expression: ${weblogic.deploymentPlanPath}", 3 );
append( sb, "", 0 );
append( sb, "exploded (Default: false)", 2 );
append( sb, "Set to true to do exploded deployments", 3 );
append( sb, "Expression: ${weblogic.exploded}", 3 );
append( sb, "", 0 );
append( sb, "ignoreHostnameVerification (Default: true)", 2 );
append( sb, "Set to true for the demo trust key store", 3 );
append( sb, "Expression: ${weblogic.ignoreHostnameVerification}", 3 );
append( sb, "", 0 );
append( sb, "localRepository", 2 );
append( sb, "The local repository", 3 );
append( sb, "Expression: ${localRepository}", 3 );
append( sb, "", 0 );
append( sb, "name", 2 );
append( sb, "The name to use when deploying the object.", 3 );
append( sb, "Expression: ${project.artifactId}", 3 );
append( sb, "", 0 );
append( sb, "noExit (Default: false)", 2 );
append( sb, "tell weblogic to not exit if there is a deployment failure.", 3 );
append( sb, "", 0 );
append( sb, "noVersion (Default: false)", 2 );
append( sb, "Tell weblogic to ignore the versions an apply the task to all versions.", 3 );
append( sb, "", 0 );
append( sb, "noWait (Default: false)", 2 );
append( sb, "Set this value to true so weblogic will return immediately", 3 );
append( sb, "", 0 );
append( sb, "outputDirectory", 2 );
append( sb, "This is the output directory for the artifacts. It defaults to $project.build.directory.", 3 );
append( sb, "Expression: ${project.build.directory}", 3 );
append( sb, "", 0 );
append( sb, "password (Default: weblogic)", 2 );
append( sb, "The admin password to access the Weblogic Admin server for deployment.", 3 );
append( sb, "Expression: ${weblogic.password}", 3 );
append( sb, "", 0 );
append( sb, "pluginArtifacts", 2 );
append( sb, "These are the plugin artifacts for the weblogic mojo", 3 );
append( sb, "Expression: ${plugin.artifacts}", 3 );
append( sb, "", 0 );
append( sb, "projectPackaging", 2 );
append( sb, "The project packaging used to check the suffix on the artifact.", 3 );
append( sb, "Expression: ${project.packaging}", 3 );
append( sb, "", 0 );
append( sb, "remote (Default: false)", 2 );
append( sb, "True if you are running on a machine that is remote to the admin server. If this is a remote deployment and this is set to false then it is assumed that all source paths are valid paths on the admin server.", 3 );
append( sb, "Expression: ${weblogic.remote}", 3 );
append( sb, "", 0 );
append( sb, "remoteRepositories", 2 );
append( sb, "The list of remote repositories", 3 );
append( sb, "Expression: ${project.remoteArtifactRepositories}", 3 );
append( sb, "", 0 );
append( sb, "skip (Default: false)", 2 );
append( sb, "The dns hostname of the Weblogic Admin server.", 3 );
append( sb, "Expression: ${weblogic.deploy.skip}", 3 );
append( sb, "", 0 );
append( sb, "stage (Default: true)", 2 );
append( sb, "True if you want to turn off staging", 3 );
append( sb, "Expression: ${weblogic.stage}", 3 );
append( sb, "", 0 );
append( sb, "targetNames (Default: AdminServer)", 2 );
append( sb, "A comma seperated list of names of servers or clusters to deploy the artifact onto.", 3 );
append( sb, "Expression: ${weblogic.targetNames}", 3 );
append( sb, "", 0 );
append( sb, "timeout (Default: -1)", 2 );
append( sb, "Set this value to the timeout seconds. Set to <=0 to disable", 3 );
append( sb, "", 0 );
append( sb, "toolsJar", 2 );
append( sb, "The the location of tools.jar file. this file must be dynamically added to the classloader due to some issues with the way maven works and a bug in jdk 1.6\nNote that the java.home location should be to the jre not the jdk", 3 );
append( sb, "Expression: ${java.home}/../lib/tools.jar", 3 );
append( sb, "", 0 );
append( sb, "upload (Default: false)", 2 );
append( sb, "Set to true to upload the code.", 3 );
append( sb, "Expression: ${weblogic.upload}", 3 );
append( sb, "", 0 );
append( sb, "userConfigFile", 2 );
append( sb, "The user config file to use", 3 );
append( sb, "Expression: ${weblogic.userConfigFile}", 3 );
append( sb, "", 0 );
append( sb, "userId (Default: weblogic)", 2 );
append( sb, "The Admin UserId to access the Weblogic Admin server for deployment.", 3 );
append( sb, "Expression: ${weblogic.user}", 3 );
append( sb, "", 0 );
append( sb, "userKeyFile", 2 );
append( sb, "The default user key file to use", 3 );
append( sb, "Expression: ${weblogic.userKeyFile}", 3 );
append( sb, "", 0 );
append( sb, "verbose (Default: true)", 2 );
append( sb, "True to turn on debugging", 3 );
append( sb, "", 0 );
append( sb, "version (Default: false)", 2 );
append( sb, "Print the version for weblogic", 3 );
append( sb, "", 0 );
append( sb, "weblogicHome (Default: ${weblogic.home})", 2 );
append( sb, "Property to set the weblogic home", 3 );
append( sb, "", 0 );
}
}
if ( goal == null || goal.length() <= 0 || "undeploy".equals( goal ) )
{
append( sb, "weblogic:undeploy", 0 );
append( sb, "Undeploy artifacts from Weblogic server(s) or cluster(s).", 1 );
append( sb, "", 0 );
if ( detail )
{
append( sb, "Available parameters:", 1 );
append( sb, "", 0 );
append( sb, "adminServerHostName (Default: localhost)", 2 );
append( sb, "The dns hostname of the Weblogic Admin server.", 3 );
append( sb, "Expression: ${weblogic.adminServer.hostName}", 3 );
append( sb, "", 0 );
append( sb, "adminServerPort (Default: 7001)", 2 );
append( sb, "The admin port of the Weblogic Admin Server.", 3 );
append( sb, "Expression: ${weblogic.adminServer.port}", 3 );
append( sb, "", 0 );
append( sb, "adminServerProtocol (Default: t3)", 2 );
append( sb, "The protocol to use to access the Weblogic Admin server for deployment.", 3 );
append( sb, "Expression: ${weblogic.adminServer.protocol}", 3 );
append( sb, "", 0 );
append( sb, "artifactPath", 2 );
append( sb, "The full path to artifact to be deployed.", 3 );
append( sb, "Expression: ${project.build.directory}/${project.build.finalName}", 3 );
append( sb, "", 0 );
append( sb, "continueOnFailure (Default: false)", 2 );
append( sb, "If set to true, the deployer will not stop if a failure is detected.", 3 );
append( sb, "", 0 );
append( sb, "customTrustKeyStoreFileName", 2 );
append( sb, "Set fully qualified name to the key store file", 3 );
append( sb, "Expression: ${weblogic.customTrustKeyStoreFileName}", 3 );
append( sb, "", 0 );
append( sb, "customTrustKeyStorePassPhrase", 2 );
append( sb, "Set passphrase for the keystore", 3 );
append( sb, "Expression: ${weblogic.customTrustKeyStorePassPhrase}", 3 );
append( sb, "", 0 );
append( sb, "customTrustKeyStoreType (Default: JKS)", 2 );
append( sb, "Set keystore type. Usually JKS", 3 );
append( sb, "Expression: ${weblogic.customTrustKeyStoreType}", 3 );
append( sb, "", 0 );
append( sb, "debug (Default: false)", 2 );
append( sb, "True to turn on debugging", 3 );
append( sb, "", 0 );
append( sb, "demoTrustKeyStore (Default: false)", 2 );
append( sb, "Set to true for the demo trust key store", 3 );
append( sb, "Expression: ${weblogic.demoTrustKeyStore}", 3 );
append( sb, "", 0 );
append( sb, "deployEjb (Default: false)", 2 );
append( sb, "The dns hostname of the Weblogic Admin server.", 3 );
append( sb, "Expression: ${weblogic.deployEjb}", 3 );
append( sb, "", 0 );
append( sb, "deploymentPlanPath", 2 );
append( sb, "Deploy the target with the referenced deployment plan", 3 );
append( sb, "Expression: ${weblogic.deploymentPlanPath}", 3 );
append( sb, "", 0 );
append( sb, "exploded (Default: false)", 2 );
append( sb, "Set to true to do exploded deployments", 3 );
append( sb, "Expression: ${weblogic.exploded}", 3 );
append( sb, "", 0 );
append( sb, "ignoreHostnameVerification (Default: true)", 2 );
append( sb, "Set to true for the demo trust key store", 3 );
append( sb, "Expression: ${weblogic.ignoreHostnameVerification}", 3 );
append( sb, "", 0 );
append( sb, "localRepository", 2 );
append( sb, "The local repository", 3 );
append( sb, "Expression: ${localRepository}", 3 );
append( sb, "", 0 );
append( sb, "name", 2 );
append( sb, "The name to use when deploying the object.", 3 );
append( sb, "Expression: ${project.artifactId}", 3 );
append( sb, "", 0 );
append( sb, "noExit (Default: false)", 2 );
append( sb, "tell weblogic to not exit if there is a deployment failure.", 3 );
append( sb, "", 0 );
append( sb, "noVersion (Default: false)", 2 );
append( sb, "Tell weblogic to ignore the versions an apply the task to all versions.", 3 );
append( sb, "", 0 );
append( sb, "noWait (Default: false)", 2 );
append( sb, "Set this value to true so weblogic will return immediately", 3 );
append( sb, "", 0 );
append( sb, "outputDirectory", 2 );
append( sb, "This is the output directory for the artifacts. It defaults to $project.build.directory.", 3 );
append( sb, "Expression: ${project.build.directory}", 3 );
append( sb, "", 0 );
append( sb, "password (Default: weblogic)", 2 );
append( sb, "The admin password to access the Weblogic Admin server for deployment.", 3 );
append( sb, "Expression: ${weblogic.password}", 3 );
append( sb, "", 0 );
append( sb, "pluginArtifacts", 2 );
append( sb, "These are the plugin artifacts for the weblogic mojo", 3 );
append( sb, "Expression: ${plugin.artifacts}", 3 );
append( sb, "", 0 );
append( sb, "projectPackaging", 2 );
append( sb, "The project packaging used to check the suffix on the artifact.", 3 );
append( sb, "Expression: ${project.packaging}", 3 );
append( sb, "", 0 );
append( sb, "remote (Default: false)", 2 );
append( sb, "True if you are running on a machine that is remote to the admin server. If this is a remote deployment and this is set to false then it is assumed that all source paths are valid paths on the admin server.", 3 );
append( sb, "Expression: ${weblogic.remote}", 3 );
append( sb, "", 0 );
append( sb, "remoteRepositories", 2 );
append( sb, "The list of remote repositories", 3 );
append( sb, "Expression: ${project.remoteArtifactRepositories}", 3 );
append( sb, "", 0 );
append( sb, "skip (Default: false)", 2 );
append( sb, "The dns hostname of the Weblogic Admin server.", 3 );
append( sb, "Expression: ${weblogic.deploy.skip}", 3 );
append( sb, "", 0 );
append( sb, "stage (Default: true)", 2 );
append( sb, "True if you want to turn off staging", 3 );
append( sb, "Expression: ${weblogic.stage}", 3 );
append( sb, "", 0 );
append( sb, "targetNames (Default: AdminServer)", 2 );
append( sb, "A comma seperated list of names of servers or clusters to deploy the artifact onto.", 3 );
append( sb, "Expression: ${weblogic.targetNames}", 3 );
append( sb, "", 0 );
append( sb, "timeout (Default: -1)", 2 );
append( sb, "Set this value to the timeout seconds. Set to <=0 to disable", 3 );
append( sb, "", 0 );
append( sb, "toolsJar", 2 );
append( sb, "The the location of tools.jar file. this file must be dynamically added to the classloader due to some issues with the way maven works and a bug in jdk 1.6\nNote that the java.home location should be to the jre not the jdk", 3 );
append( sb, "Expression: ${java.home}/../lib/tools.jar", 3 );
append( sb, "", 0 );
append( sb, "upload (Default: false)", 2 );
append( sb, "Set to true to upload the code.", 3 );
append( sb, "Expression: ${weblogic.upload}", 3 );
append( sb, "", 0 );
append( sb, "userConfigFile", 2 );
append( sb, "The user config file to use", 3 );
append( sb, "Expression: ${weblogic.userConfigFile}", 3 );
append( sb, "", 0 );
append( sb, "userId (Default: weblogic)", 2 );
append( sb, "The Admin UserId to access the Weblogic Admin server for deployment.", 3 );
append( sb, "Expression: ${weblogic.user}", 3 );
append( sb, "", 0 );
append( sb, "userKeyFile", 2 );
append( sb, "The default user key file to use", 3 );
append( sb, "Expression: ${weblogic.userKeyFile}", 3 );
append( sb, "", 0 );
append( sb, "verbose (Default: true)", 2 );
append( sb, "True to turn on debugging", 3 );
append( sb, "", 0 );
append( sb, "version (Default: false)", 2 );
append( sb, "Print the version for weblogic", 3 );
append( sb, "", 0 );
append( sb, "weblogicHome (Default: ${weblogic.home})", 2 );
append( sb, "Property to set the weblogic home", 3 );
append( sb, "", 0 );
}
}
if ( goal == null || goal.length() <= 0 || "wsdlgen".equals( goal ) )
{
append( sb, "weblogic:wsdlgen", 0 );
append( sb, "This class generates wsdl from ear/war package", 1 );
append( sb, "", 0 );
if ( detail )
{
append( sb, "Available parameters:", 1 );
append( sb, "", 0 );
append( sb, "defaultEndpoint (Default: http://localhost:7001/)", 2 );
append( sb, "The default endpoint address", 3 );
append( sb, "", 0 );
append( sb, "localRepository", 2 );
append( sb, "The local repository", 3 );
append( sb, "Expression: ${localRepository}", 3 );
append( sb, "", 0 );
append( sb, "outputDirectory", 2 );
append( sb, "This is the output directory for the artifacts. It defaults to $project.build.directory.", 3 );
append( sb, "Expression: ${project.build.directory}", 3 );
append( sb, "", 0 );
append( sb, "overwrite (Default: true)", 2 );
append( sb, "Set to false to not overwrite existing resources.", 3 );
append( sb, "", 0 );
append( sb, "pluginArtifacts", 2 );
append( sb, "These are the plugin artifacts for the weblogic mojo", 3 );
append( sb, "Expression: ${plugin.artifacts}", 3 );
append( sb, "", 0 );
append( sb, "remoteRepositories", 2 );
append( sb, "The list of remote repositories", 3 );
append( sb, "Expression: ${project.remoteArtifactRepositories}", 3 );
append( sb, "", 0 );
append( sb, "serviceName", 2 );
append( sb, "The service name to generate the wsdl for", 3 );
append( sb, "Required: Yes", 3 );
append( sb, "", 0 );
append( sb, "toolsJar", 2 );
append( sb, "The the location of tools.jar file. this file must be dynamically added to the classloader due to some issues with the way maven works and a bug in jdk 1.6\nNote that the java.home location should be to the jre not the jdk", 3 );
append( sb, "Expression: ${java.home}/../lib/tools.jar", 3 );
append( sb, "", 0 );
append( sb, "warName", 2 );
append( sb, "The war name inside of the ear that contains the services", 3 );
append( sb, "Required: Yes", 3 );
append( sb, "", 0 );
append( sb, "weblogicHome (Default: ${weblogic.home})", 2 );
append( sb, "Property to set the weblogic home", 3 );
append( sb, "", 0 );
append( sb, "wsdlFile", 2 );
append( sb, "The wsdl file to output when complete.", 3 );
append( sb, "Expression: ${project.build.directory}/${project.build.finalName}.wsdl", 3 );
append( sb, "", 0 );
}
}
if ( getLog().isInfoEnabled() )
{
getLog().info( sb.toString() );
}
}
/**
* Repeat a String n
times to form a new string.
*
* @param str String to repeat
* @param repeat number of times to repeat str
* @return String with repeated String
* @throws NegativeArraySizeException if repeat < 0
* @throws NullPointerException if str is null
*/
private static String repeat( String str, int repeat )
{
StringBuffer buffer = new StringBuffer( repeat * str.length() );
for ( int i = 0; i < repeat; i++ )
{
buffer.append( str );
}
return buffer.toString();
}
/**
* Append a description to the buffer by respecting the indentSize and lineLength parameters.
* Note: The last character is always a new line.
*
* @param sb The buffer to append the description, not null
.
* @param description The description, not null
.
* @param indent The base indentation level of each line, must not be negative.
*/
private void append( StringBuffer sb, String description, int indent )
{
for ( Iterator it = toLines( description, indent, indentSize, lineLength ).iterator(); it.hasNext(); )
{
sb.append( it.next().toString() ).append( '\n' );
}
}
/**
* Splits the specified text into lines of convenient display length.
*
* @param text The text to split into lines, must not be null
.
* @param indent The base indentation level of each line, must not be negative.
* @param indentSize The size of each indentation, must not be negative.
* @param lineLength The length of the line, must not be negative.
* @return The sequence of display lines, never null
.
* @throws NegativeArraySizeException if indent < 0
*/
private static List toLines( String text, int indent, int indentSize, int lineLength )
{
List lines = new ArrayList();
String ind = repeat( "\t", indent );
String[] plainLines = text.split( "(\r\n)|(\r)|(\n)" );
for ( int i = 0; i < plainLines.length; i++ )
{
toLines( lines, ind + plainLines[i], indentSize, lineLength );
}
return lines;
}
/**
* Adds the specified line to the output sequence, performing line wrapping if necessary.
*
* @param lines The sequence of display lines, must not be null
.
* @param line The line to add, must not be null
.
* @param indentSize The size of each indentation, must not be negative.
* @param lineLength The length of the line, must not be negative.
*/
private static void toLines( List lines, String line, int indentSize, int lineLength )
{
int lineIndent = getIndentLevel( line );
StringBuffer buf = new StringBuffer( 256 );
String[] tokens = line.split( " +" );
for ( int i = 0; i < tokens.length; i++ )
{
String token = tokens[i];
if ( i > 0 )
{
if ( buf.length() + token.length() >= lineLength )
{
lines.add( buf.toString() );
buf.setLength( 0 );
buf.append( repeat( " ", lineIndent * indentSize ) );
}
else
{
buf.append( ' ' );
}
}
for ( int j = 0; j < token.length(); j++ )
{
char c = token.charAt( j );
if ( c == '\t' )
{
buf.append( repeat( " ", indentSize - buf.length() % indentSize ) );
}
else if ( c == '\u00A0' )
{
buf.append( ' ' );
}
else
{
buf.append( c );
}
}
}
lines.add( buf.toString() );
}
/**
* Gets the indentation level of the specified line.
*
* @param line The line whose indentation level should be retrieved, must not be null
.
* @return The indentation level of the line.
*/
private static int getIndentLevel( String line )
{
int level = 0;
for ( int i = 0; i < line.length() && line.charAt( i ) == '\t'; i++ )
{
level++;
}
for ( int i = level + 1; i <= level + 4 && i < line.length(); i++ )
{
if ( line.charAt( i ) == '\t' )
{
level++;
break;
}
}
return level;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy